Node Redis Get Key (Detailed Guide w/ Code Examples)
Use Case(s)
In Node.js, the Redis GET command is used to retrieve the value of a given key. It is commonly used when you need the value associated with a key for further processing. This could be during user authentication to fetch user details, caching data to improve performance, or managing sessions.
Code Examples
Here are a few examples of how you use the get
command in Node.js with Redis:
Example 1: Simple usage of GET
const redis = require('redis');
const client = redis.createClient();
client.get('key', function(err, reply) {
console.log(reply);
});
In this example, we simply connect to the Redis server and get the value of 'key'. We then log the reply to the console.
Example 2: Error handling with GET
const redis = require('redis');
const client = redis.createClient();
client.get('nonexistentkey', function(err, reply) {
if (err) {
console.error('There was an error', err);
} else if (reply === null) {
console.log('The key does not exist');
} else {
console.log(reply);
}
});
In this example, we try to get the value of a key that doesn't exist. In the callback function, we handle any potential errors and also check whether the reply is null to determine if the key exists.
Best Practices
Ensure proper error handling is in place, as shown in the second example. This includes checking for null values which indicate that a key does not exist.
Common Mistakes
One common mistake is forgetting to check if a key exists before attempting to retrieve its value. If you do this and the key doesn't exist, Redis will return null, which could potentially lead to undesired behavior in your application.
FAQs
Q: What happens if I try to get the value of a key that doesn't exist? A: Redis will return null
.
Q: What are other common commands in Node.js Redis? A: Some other common commands include SET, DEL, EXPIRE, etc.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Node Redis: Get Replica
- Node Redis: Get All Keys
- Node Redis: Get First 10 Keys
- Node Redis: Get All Keys and Values
- Node Redis: Get Length of List
- Get All Hash Keys with Redis in Node.js
- Node Redis: Get Hash Values at Key
- Node Redis: Get Current Memory Usage
- Node Redis: Get All Keys Matching Pattern
- Node Redis: Get Keys by TTL
- Node Redis: Getting All Databases
- Getting Master Status in Node Redis
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
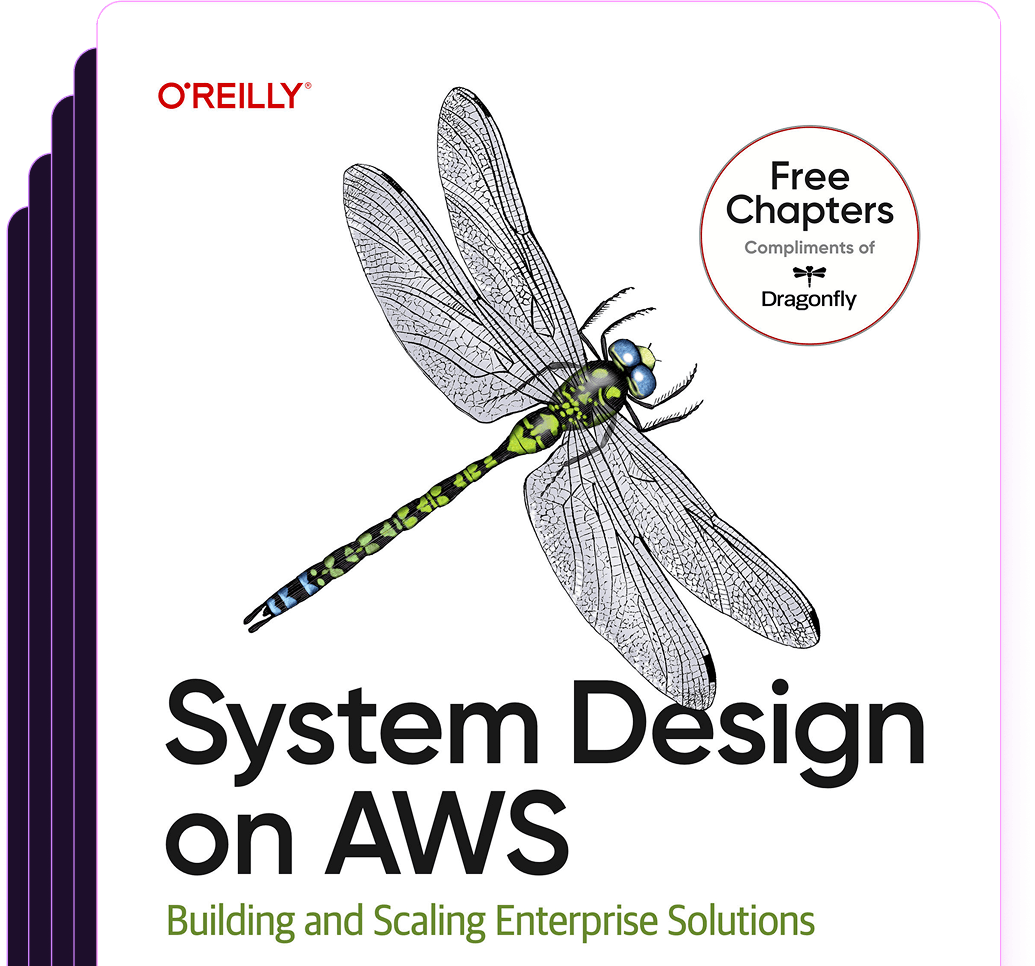
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost