Node Redis: Get All Keys and Values (Detailed Guide w/ Code Examples)
Use Case(s)
In Node.js applications using Redis as a database, you might want to retrieve all keys and their corresponding values from Redis. This is common when you need to analyse or process all the data stored within the database.
Code Examples
Let's assume we have a connection to our Redis server already established using the redis
package in Node.js.
Example 1: Retrieving all keys and their values:
const redis = require('redis');
const client = redis.createClient();
client.keys('*', (err, keys) => {
if (err) throw err;
keys.forEach(key => {
client.get(key, (err, value) => {
if (err) throw err;
console.log(`${key}: ${value}`);
});
});
});
In this example, the keys
command is used with a wildcard '*' to fetch all keys. Then for each key, we use get
to fetch the value.
Best Practices
Ensure error handling is in place when working with Redis operations in Node.js. It's also a good practice to close the Redis connection once operations are completed to free up resources.
Common Mistakes
Avoid using the keys
command in a production environment since it can potentially block the server while it retrieves keys, particularly when dealing with large databases.
FAQs
Q: Can I get all keys and values in one command in Redis? A: No, Redis does not natively support fetching all keys and their values in a single command. Instead, you need to first get all keys, then iterate over them to get each value.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Node Redis: Get Replica
- Node Redis Get Key
- Node Redis: Get All Keys
- Node Redis: Get First 10 Keys
- Node Redis: Get Length of List
- Get All Hash Keys with Redis in Node.js
- Node Redis: Get Hash Values at Key
- Node Redis: Get Current Memory Usage
- Node Redis: Get All Keys Matching Pattern
- Node Redis: Get Keys by TTL
- Node Redis: Getting All Databases
- Getting Master Status in Node Redis
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
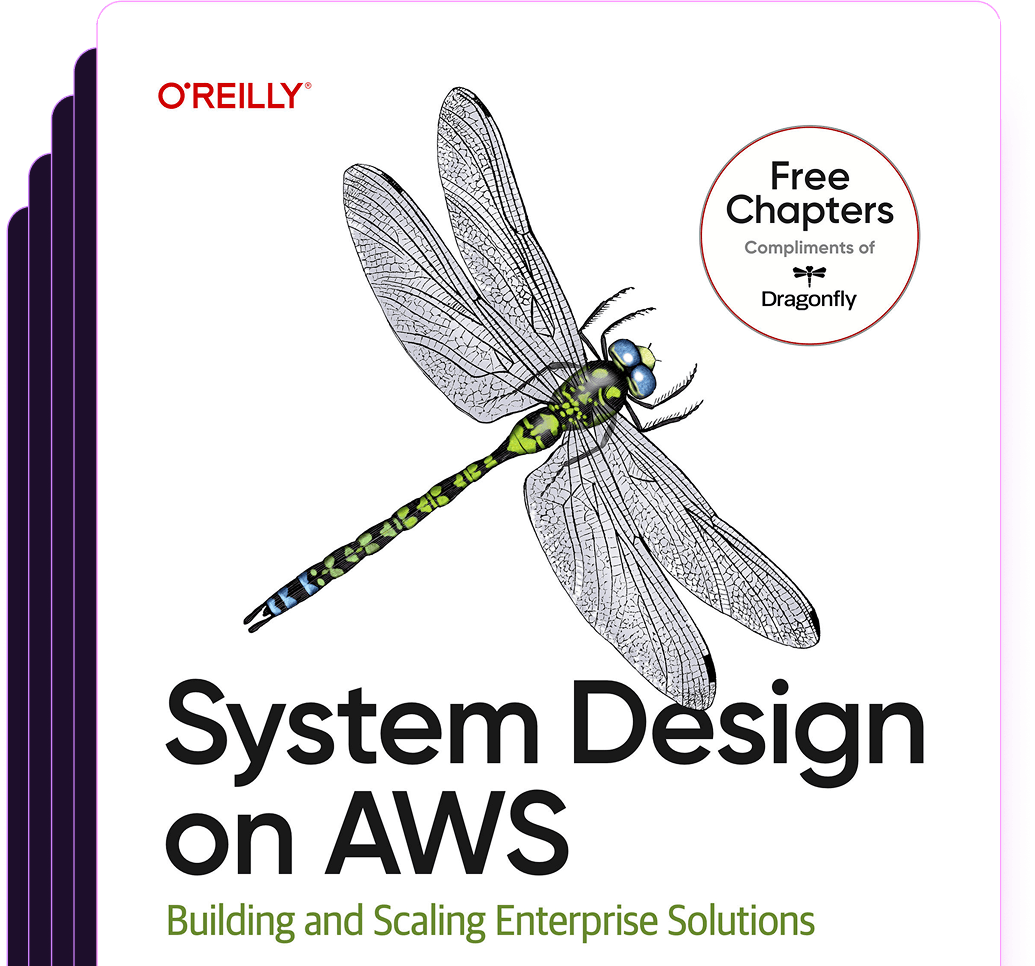
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost