Getting Master Status in Node Redis (Detailed Guide w/ Code Examples)
Use Case(s)
In a Redis replication setup, it's common to need to know the status of the master node. This could be used for monitoring health and performance, debugging synchronization issues, or managing automated failovers.
Code Examples
Node.js does not provide a direct function to check the master status of a Redis server. However, you can use the INFO
command which provides information about the Redis server including its role (master or slave).
Here is an example:
const redis = require('redis');
const client = redis.createClient();
client.info('Replication', function(err, reply) {
const lines = reply.split('\r\n');
const roleLine = lines.find(line => line.startsWith('role'));
console.log(roleLine); // Outputs: role:master or role:slave
});
This script connects to the Redis server using redis.createClient()
, then sends an INFO Replication
command to the server. The response is parsed to find and output the server's role.
Best Practices
When using Node.js with Redis, it's important to handle errors and close connections properly. Be sure to listen for error
events on your client objects and call quit
when you're done with a client to avoid leaking resources.
Common Mistakes
One common mistake is not handling Redis connection errors, which can lead to unhandled exceptions in your application. Always attach an error handler to your Redis clients.
FAQs
Q: How do I handle failovers in Node.js with Redis?
A: If you're using Sentinel or Cluster mode, the Node.js ioredis
library supports automatic failover. For standalone servers, you will need to implement failover logic in your application.
Q: Can I use this method to get other information about the Redis server?
A: Yes, the INFO
command returns a lot of information about the server including memory usage, CPU usage, client connections, and more. You can parse this information from the reply string as needed.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Node Redis: Get Replica
- Node Redis Get Key
- Node Redis: Get All Keys
- Node Redis: Get First 10 Keys
- Node Redis: Get All Keys and Values
- Node Redis: Get Length of List
- Get All Hash Keys with Redis in Node.js
- Node Redis: Get Hash Values at Key
- Node Redis: Get Current Memory Usage
- Node Redis: Get All Keys Matching Pattern
- Node Redis: Get Keys by TTL
- Node Redis: Getting All Databases
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
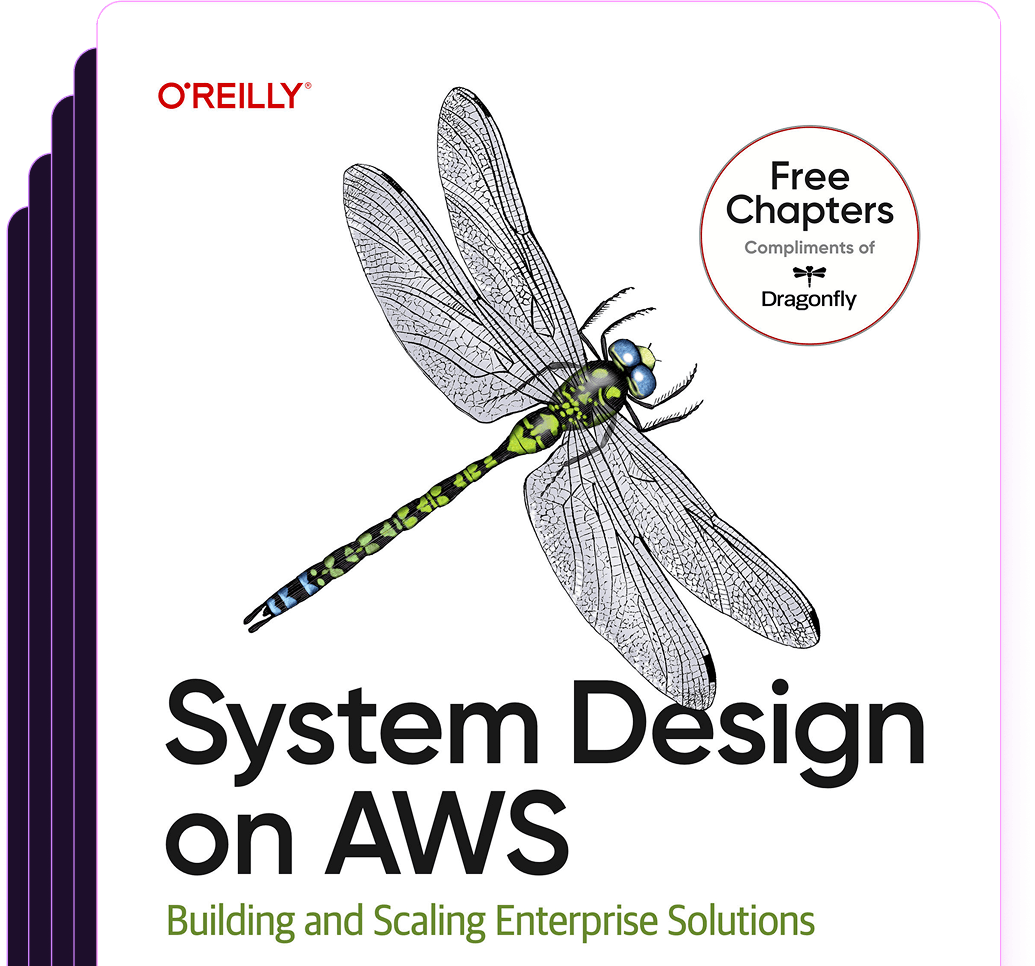
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost