Get All Hash Keys with Redis in Node.js (Detailed Guide w/ Code Examples)
Use Case(s)
Using Redis with Node.js can provide efficient and scalable data storage for your applications. One common use case is retrieving all hash keys stored within a specific hash. This is useful when you need to examine or manipulate all entries of a hash.
Code Examples
To fetch all keys in a hash, you'll want to use the HKEYS
command. Here's an example using the node_redis
package:
const redis = require('redis');
const client = redis.createClient();
client.hkeys('hashKey', function(err, keys) {
if (err) throw err;
console.log(keys); // Will log array of keys
});
In this example, 'hashKey' is the key of the hash you want to retrieve all keys from. The callback function will be called with an error (or null) and an array of keys.
Best Practices
- Always handle errors that may arise during the execution of the
hkeys
command. - Close the connection to the Redis client after you've finished your operations to prevent memory leaks.
Common Mistakes
- Not checking if the targeted hash exists before trying to retrieve keys from it. This will result in an empty list without any indication that there might be an error in your code.
FAQs
Q: Can I get all keys and values at the same time? A: Yes. For this, you can use the HGETALL
command instead of HKEYS
. This will return an object with key-value pairs.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Node Redis: Get Replica
- Node Redis Get Key
- Node Redis: Get All Keys
- Node Redis: Get First 10 Keys
- Node Redis: Get All Keys and Values
- Node Redis: Get Length of List
- Node Redis: Get Hash Values at Key
- Node Redis: Get Current Memory Usage
- Node Redis: Get All Keys Matching Pattern
- Node Redis: Get Keys by TTL
- Node Redis: Getting All Databases
- Getting Master Status in Node Redis
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
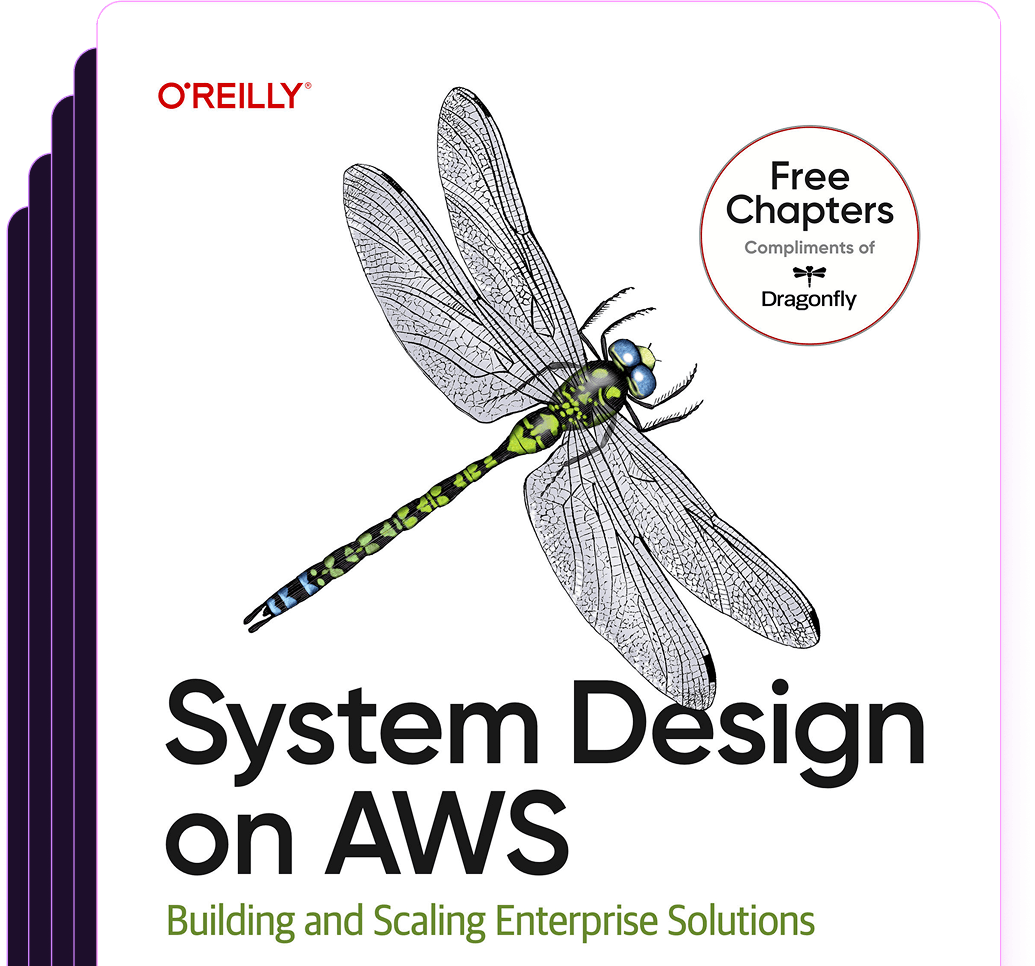
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost