Delete Redis Cache in PHP (Detailed Guide w/ Code Examples)
Use Case(s)
PHP developers often use a Redis cache to increase the performance of their applications by storing frequently accessed dataset. However, there are times when you might need to delete certain keys or even all keys from the Redis cache. This operation could be implemented during development/testing phase or when the cached data becomes stale and needs to be refreshed.
Code Examples
- Deleting a single key
In PHP, you can use del
method of Redis class to delete a key from the Redis cache:
$redis = new Redis();
$redis->connect('localhost', 6379);
$redis->del('key');
This code connects to the Redis server running on localhost at port 6379, and deletes the key named 'key'.
- Deleting multiple keys
You can also delete multiple keys at once:
$redis = new Redis();
$redis->connect('localhost', 6379);
$redis->del(['key1', 'key2', 'key3']);
This code deletes the keys 'key1', 'key2', and 'key3'.
- Deleting all keys
To delete all keys from the Redis cache, you can use the flushAll
method:
$redis = new Redis();
$redis->connect('localhost', 6379);
$redis->flushAll();
Best Practices
- Always connect to the Redis server in a try-catch block to handle connection errors gracefully.
- Use appropriate data structures provided by Redis to minimize the use of
del
orflushAll
commands that can affect performance.
Common Mistakes
- Not handling exceptions while connecting to the Redis server, which could lead to application crash if the server is not available.
- Using delete operations frequently. It's better to design the cache in such a way that it automatically invalidates stale data.
FAQs
- Can I recover keys once they are deleted from the Redis cache?
No, once a key is deleted, it cannot be recovered.
- What is the difference between
del
andflushAll
methods?
The del
method deletes specific keys from the Redis cache while flushAll
deletes all keys.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
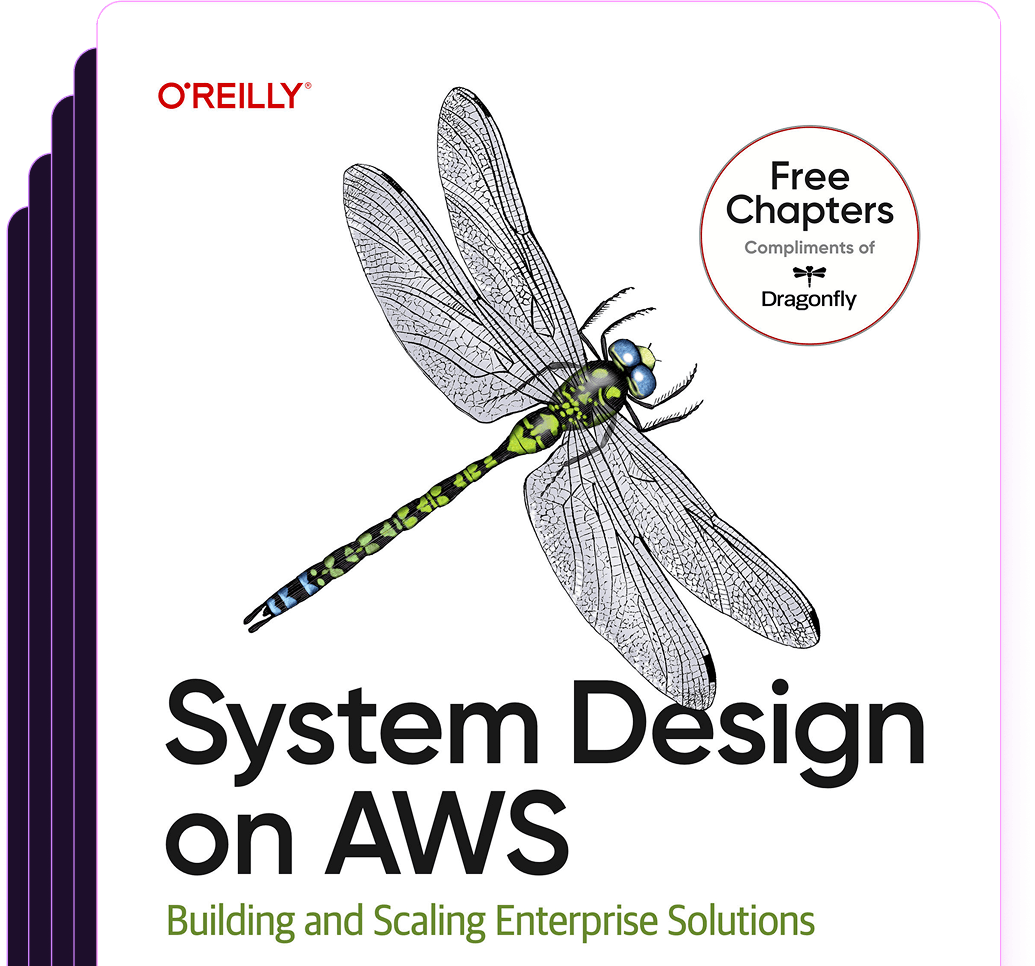
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost