Deleting a Key in Redis with PHP (Detailed Guide w/ Code Examples)
Use Case(s)
One common use case for deleting keys in Redis using PHP includes removing outdated or no longer necessary data from the cache to maintain its efficiency.
Code Examples
Example 1:
<?php
$redis = new Redis();
$redis->connect('127.0.0.1',6379);
$redis->delete('key');
?>
In this example, we initialize a new Redis object and connect it to the Redis server running on localhost at port 6379. Then we delete the key named 'key'.
Example 2:
<?php
$redis = new Redis();
$redis->connect('127.0.0.1',6379);
$keysToDelete = ['key1', 'key2', 'key3'];
foreach ($keysToDelete as $key) {
$redis->delete($key);
}
?>
In this example, we delete multiple keys 'key1', 'key2', 'key3' from the Redis server. We loop over an array of keys and delete each one.
Best Practices
- Always check if a key exists before attempting to delete it. This will prevent any potential errors.
- Bulk delete operations should be used with caution as they can lead to high memory and CPU usage.
Common Mistakes
- Attempting to delete a non-existing key. This might not cause a runtime error, but it can lead to confusion when debugging.
FAQs
Q: What happens if I try to delete a key that doesn't exist? A: Redis will simply ignore this and continue with the execution. It won't return an error.
Q: Does the delete method work with all types of Redis keys, like lists, sets, hashes, etc.? A: Yes, the delete method can be used to remove any type of key-value pair in Redis, regardless of the data type.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
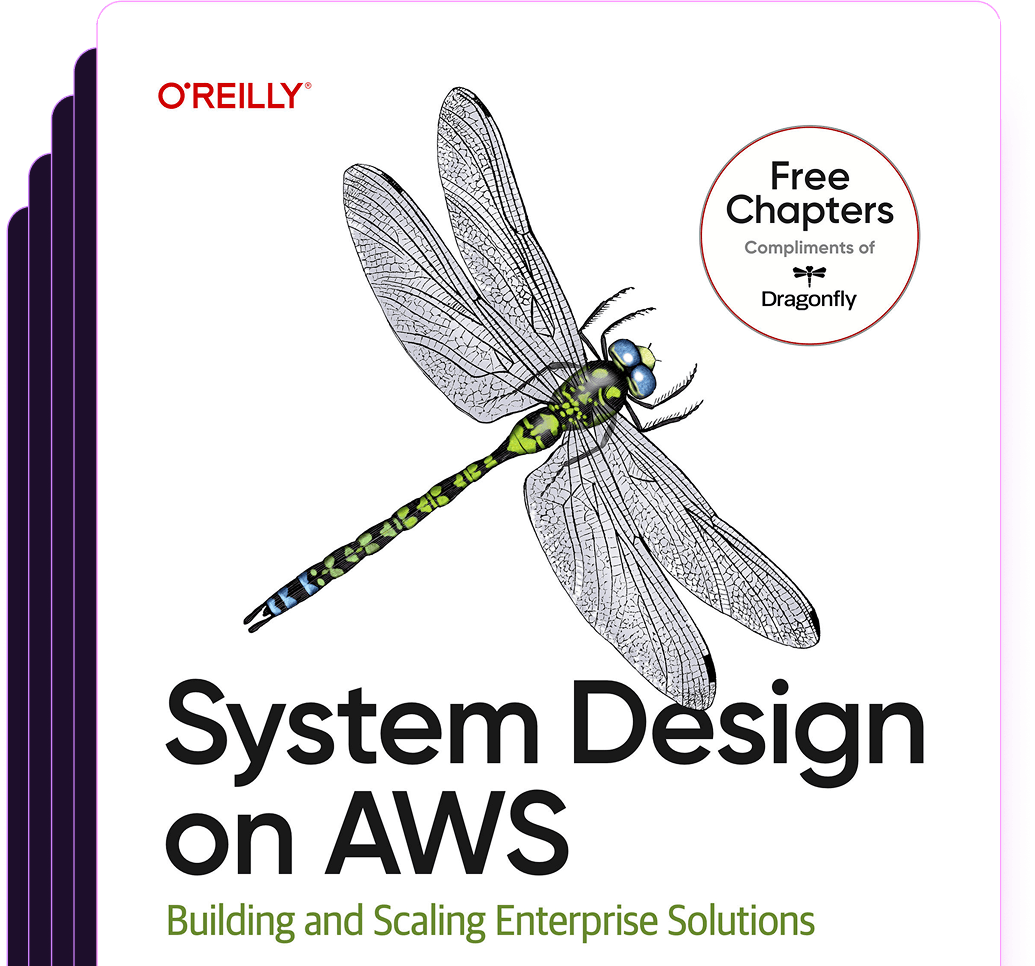
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost