PHP Redis: Deleting Hash (Detailed Guide w/ Code Examples)
Use Case(s)
Deleting a hash in Redis is a common operation when you want to remove an entire hash data structure from your Redis database. This can be useful when the hash is no longer needed or when you want to clear up space.
Code Examples
In PHP, you can use the hDel
function of the Redis class to delete one or more keys from a hash. You need to pass the name of the hash and the key(s) you want to delete.
$redis = new Redis();
$redis->connect('127.0.0.1', 6379);
$hashName = 'my_hash';
$keyToDelete = 'key1';
$redis->hDel($hashName, $keyToDelete);
In this example, we are deleting the 'key1' from 'my_hash'. If you want to delete multiple keys, you can just add more keys as additional arguments:
$keysToDelete = ['key1', 'key2'];
foreach ($keysToDelete as $key) {
$redis->hDel($hashName, $key);
}
This will delete 'key1' and 'key2' from 'my_hash'.
To delete the entire hash, you can use the del
method:
$redis->del($hashName);
This will delete the entire 'my_hash'.
Best Practices
Always check if the hash and the key exist before attempting to delete to avoid unnecessary operations. Additionally, be careful when deleting hashes as this operation cannot be undone.
Common Mistakes
Avoid using del
method when you only want to delete specific keys from the hash. Using del
will delete the entire hash.
FAQs
- Can I undo a deletion? - No, once a hash or a key is deleted, it cannot be undone.
- How can I check if a key exists in a hash? - You can use the
hExists
method of the Redis class in PHP.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
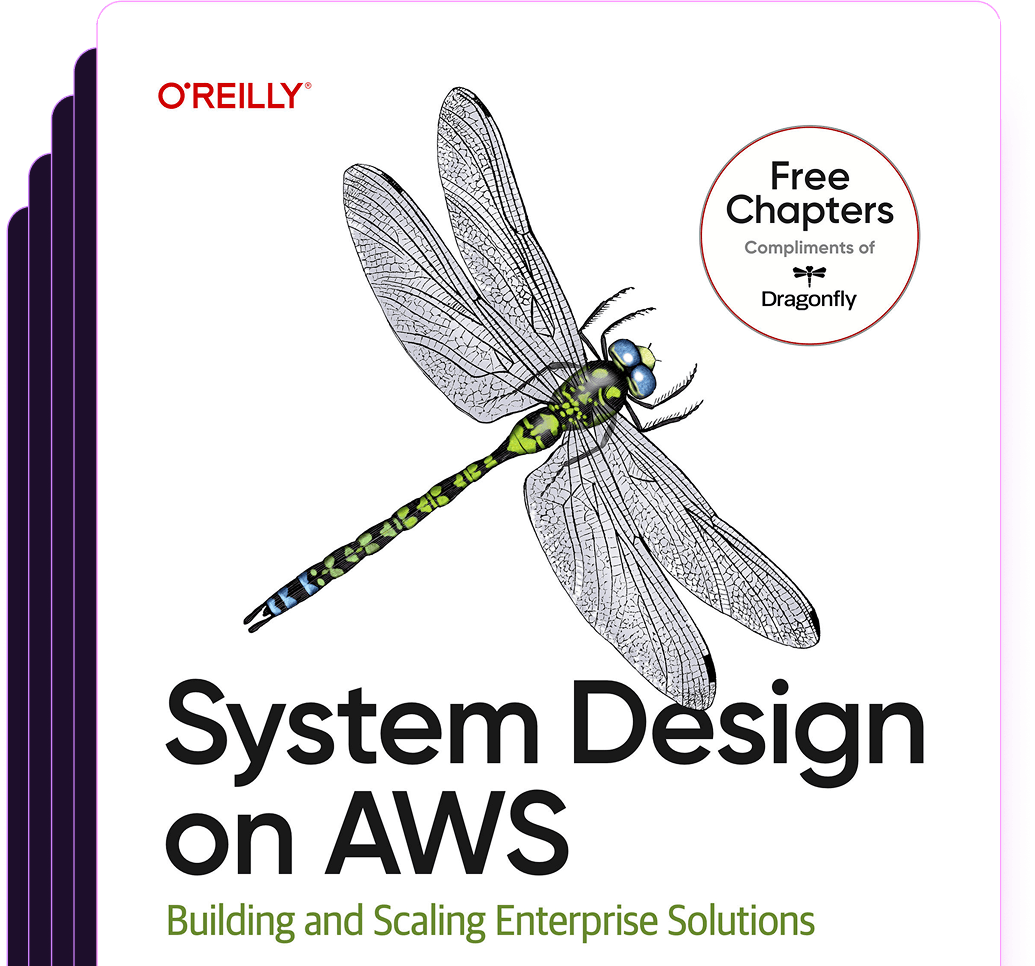
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost