Redis Delete Key After Time in PHP (Detailed Guide w/ Code Examples)
Use Case(s)
The specific use case for deleting a key after some time (expiry) in Redis using PHP is to clear up space and maintain only relevant data. This is particularly useful in scenarios such as cache management, session storage, or any temporary data storage.
Code Examples
Example 1: Using expire
function
In PHP, we can set an expiry time on a key in Redis by using the expire
function. Here's an example where we're setting an expiry of 10 seconds on a key named 'test'.
$redis = new Redis();
$redis->connect('127.0.0.1', 6379);
$redis->set('test', 'value');
$redis->expire('test', 10); // key will be deleted after 10 seconds
Example 2: Using setex
function
Alternatively, you can use the setex
method to store a key-value pair where the key automatically gets deleted after a specified amount of time.
$redis = new Redis();
$redis->connect('127.0.0.1', 6379);
$redis->setex('test', 10, 'value'); // key will be deleted after 10 seconds
Best Practices
- Only use keys expiration when necessary as it adds extra overhead to the Redis server.
- Try to use the
setex
function when you know that the key should expire at the time of setting the value itself.
Common Mistakes
- One common mistake is to forget handling the scenario when the key has already expired before it's accessed.
FAQs
- Can I get the remaining time of a key in Redis using PHP?
Yes, you can use the ttl
function in PHP to get the remaining time in seconds for a key in Redis.
$redis = new Redis();
$redis->connect('127.0.0.1', 6379);
$redis->set('test', 'value');
$redis->expire('test', 10); // key will be deleted after 10 seconds
echo $redis->ttl('test'); // prints the remaining time for the key in seconds
- What happens if I set a negative expire time for a key in Redis?
If you set a negative expiry time, the key will be deleted immediately.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
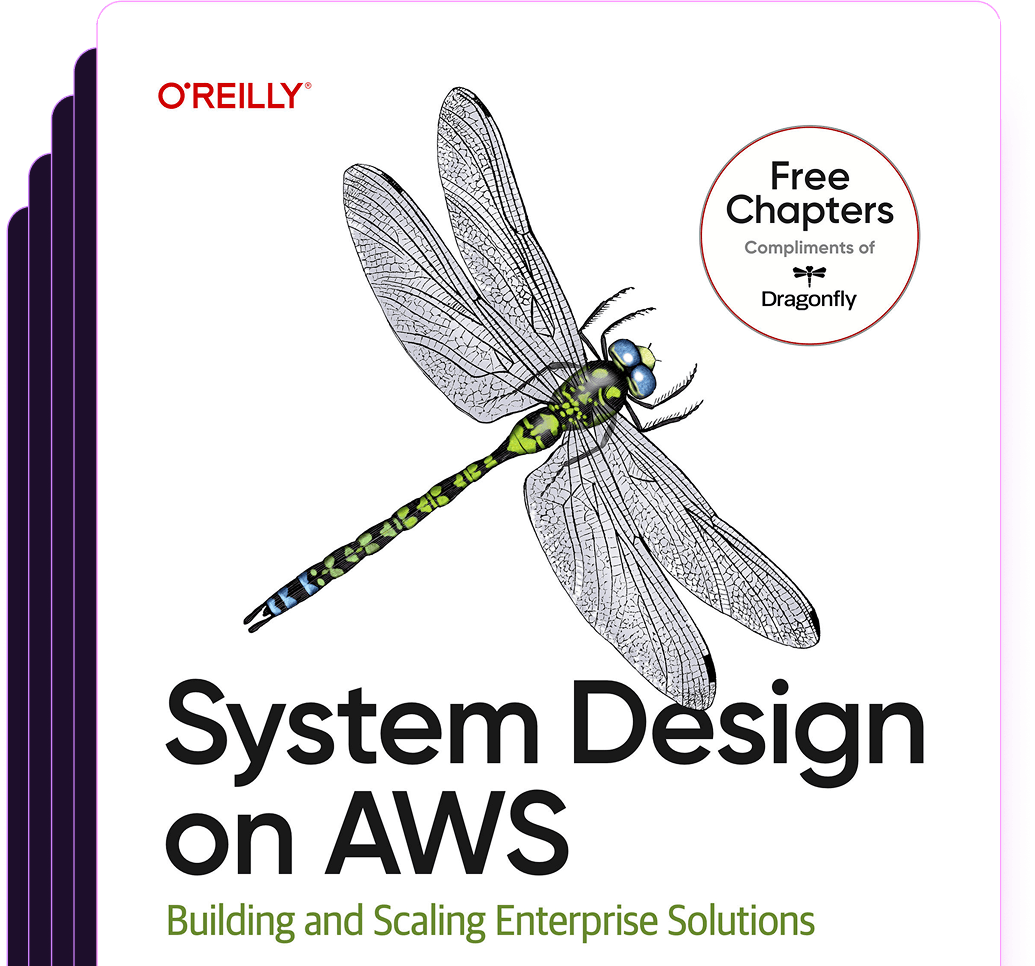
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost