Deleting Redis Cache in Python (Detailed Guide w/ Code Examples)
Use Case(s)
Deleting a Redis cache in Python is commonly used when you want to remove specific data from your cache. This could be because the data is no longer needed, or it has become outdated. It's also useful when you need to clear all the data from your cache to free up memory space.
Code Examples
Deleting a single key
You can use the delete()
method provided by the redis-py
client to remove a specific key from the Redis cache.
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
r.delete('key')
In this example, we're connecting to a local Redis server and deleting the key labeled 'key'.
Deleting multiple keys
If you need to delete multiple keys at once, you can pass them as arguments to the delete()
method.
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
r.delete('key1', 'key2', 'key3')
This example demonstrates how to delete 'key1', 'key2', and 'key3' from the Redis cache.
Best Practices
- Always check if the key exists before trying to delete it to prevent errors.
- Be careful while deleting keys, especially when using patterns, as you might end up deleting important data.
Common Mistakes
- Trying to delete a key that doesn't exist. Although Redis will not throw an error, it can lead to confusion.
- Not being aware that the
delete
command is case sensitive. Ensure that the case of your keys matches when attempting to delete.
FAQs
- Can I delete all keys in a Redis cache using Python? Yes, you can delete all keys by using the
flushdb()
orflushall()
methods. Be cautious as this will clear your entire database or all databases respectively.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
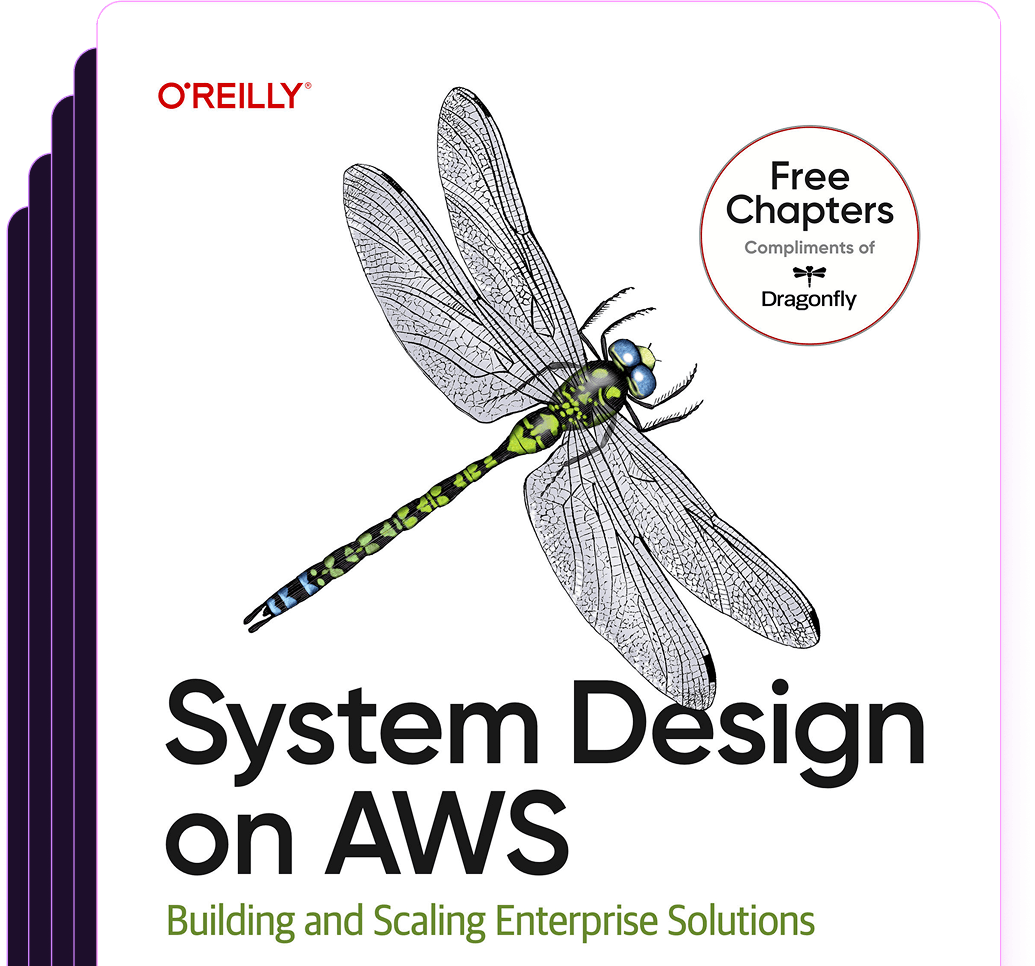
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost