Deleting Redis Keys by Pattern in Python (Detailed Guide w/ Code Examples)
Use Case(s)
Sometimes there might be a need to delete multiple keys that match a specific pattern in Redis. This is particularly common when you want to clean up or reset your cache, or when certain data becomes irrelevant.
In Python, the 'redis' library can be used to interact with a Redis database, including deleting keys based on patterns.
Code Examples
First, installation of redis library if not already installed:
pip install redis
Example 1: Deleting keys matching a specific pattern:
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
for key in r.scan_iter('pattern*'):
r.delete(key)
In this example, the scan_iter
function finds all keys that match the given pattern ('pattern*' in this case), and for each of these keys, the delete
method is called to remove them from the Redis database.
Best Practices
- Be careful when deleting keys; ensure you have the correct pattern, as it's not reversible.
- When dealing with large databases, consider using a cursor with
scan_iter
to avoid blocking the database for too long.
Common Mistakes
- A common mistake is not considering that the deletion operation could fail. Always check the return value of the
delete
method, which will indicate whether the deletion was successful.
FAQs
Q: Can I use wildcards in my pattern?
A: Yes, the pattern can include wildcard characters. For example, 'user:' will match all keys that start with 'user:', and '' will match all keys.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
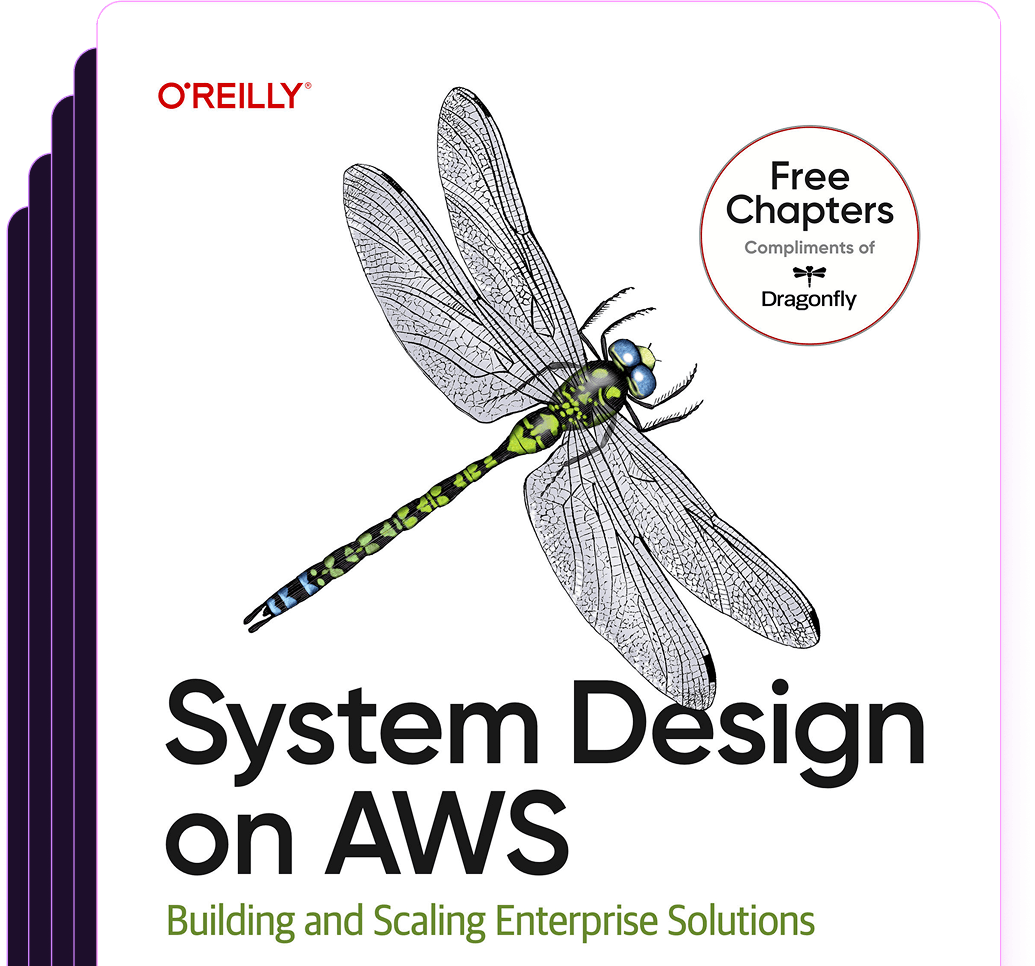
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost