Deleting Elements from a Sorted Set in Redis using Python (Detailed Guide w/ Code Examples)
Use Case(s)
- Deleting specific elements from a sorted set in Redis when you no longer need them.
- Removing all elements from a sorted set to clean up memory.
Code Examples
To interact with Redis in Python, we can use the redis-py library. Here are examples of how to delete elements from a sorted set.
Example 1: Deleting a single element:
In this example, we're deleting a single element from our sorted set. We'll use the ZREM command for this.
import redis
r = redis.Redis()
r.zrem('my_sorted_set', 'element')
In this code, 'my_sorted_set' is the name of our sorted set and 'element' is the value we want to remove.
Example 2: Deleting all elements:
If we want to remove all elements from a sorted set, it might be easier to just delete the entire set with the DEL command.
import redis
r = redis.Redis()
r.delete('my_sorted_set')
This will delete the entire sorted set named 'my_sorted_set'.
Best Practices
- Always check that an element or set exists before attempting to delete it to prevent errors.
- Be cautious when deleting sets as this cannot be undone.
Common Mistakes
- Attempting to delete an element from a non-existing set or a set that doesn't contain the element.
- Accidentally deleting the entire set instead of specific elements.
FAQs
Q: Can I delete multiple elements from a sorted set at once?
A: Yes, you can use the ZREM command with multiple arguments to remove more than one element at a time. For example: r.zrem('my_sorted_set', 'element1', 'element2', 'element3')
.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
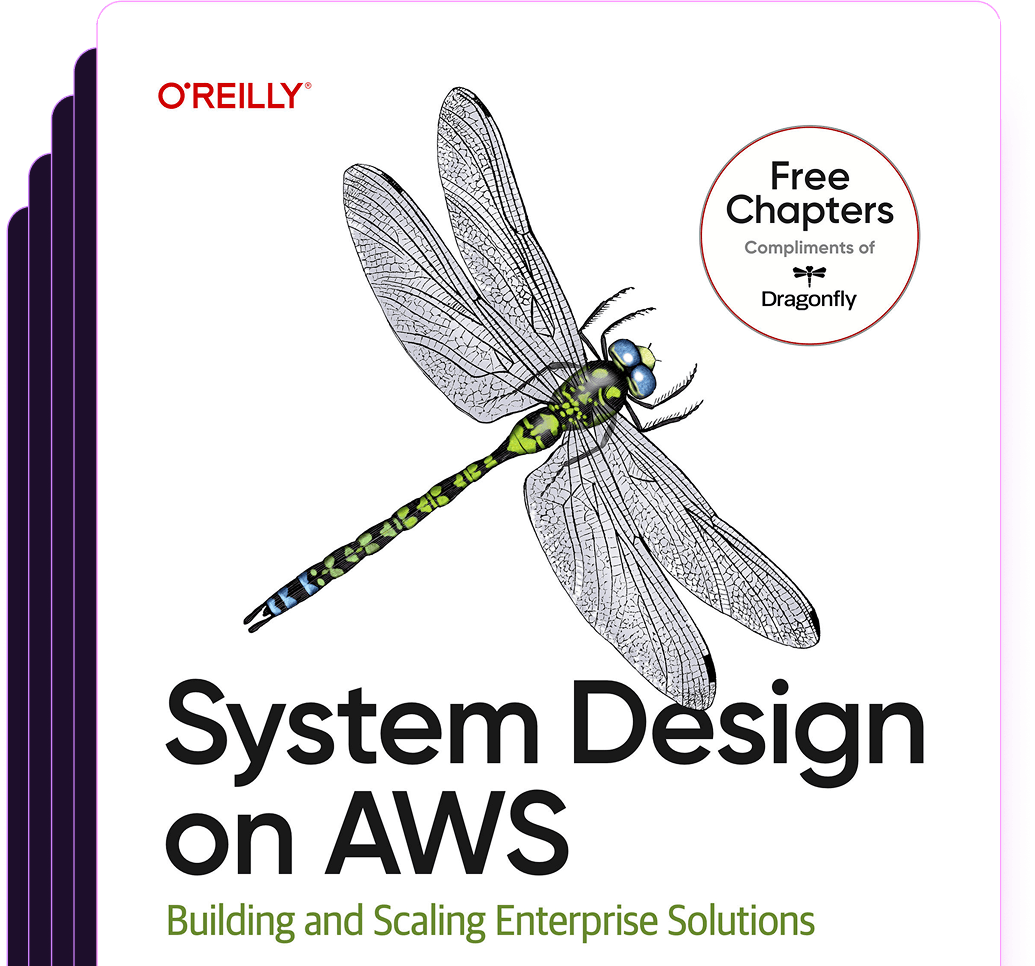
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost