Deleting a Redis Key after Time in Python (Detailed Guide w/ Code Examples)
Use Case(s)
Using Redis in Python often involves setting keys that might have to be deleted after a particular time. This can be useful in various scenarios such as caching data which needs to be refreshed after a certain period, sessions expiring after inactivity, etc.
Code Examples
Here's how you can set a key to be deleted automatically after a specified time in Redis using Python:
import redis
r = redis.Redis()
r.setex('my_key', 'my_value', 60) # The key will be deleted after 60 seconds
In this code snippet, the setex()
method is used which sets a key to a value with an expiry time. The first argument is the key, second is the value and the third is the time-to-live (TTL) in seconds after which the key will be automatically deleted from Redis.
If you already have a key-value pair in Redis and you want to set a TTL for it, you can use the expire()
method like so:
import redis
r = redis.Redis()
r.set('my_key', 'my_value')
r.expire('my_key', 120) # The key will expire after 120 seconds
The expire()
method takes two arguments - the key and the TTL in seconds.
Best Practices
- Be careful with the TTL values. Setting them too short might cause the keys to disappear before they are used. Setting them too long might defeat the purpose of setting them at all.
- Always check the return value of the
expire()
function. If it returns False, it means the key does not exist or the timeout could not be set.
Common Mistakes
One common mistake is to use a Redis instance that is not persistent for storing keys with TTL. If the Redis server is restarted, all data in it will be lost. So, ensure your Redis data persists across restarts if you're using TTLs.
FAQs
Q: Can I check the remaining TTL of a key? A: Yes, you can use the ttl()
method to get the remaining time-to-live for a key. It returns -1 if the key exists but has no associated expire.
Q: What happens if I set a new TTL for a key that already had a TTL? A: The old TTL will be overwritten with the new one.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
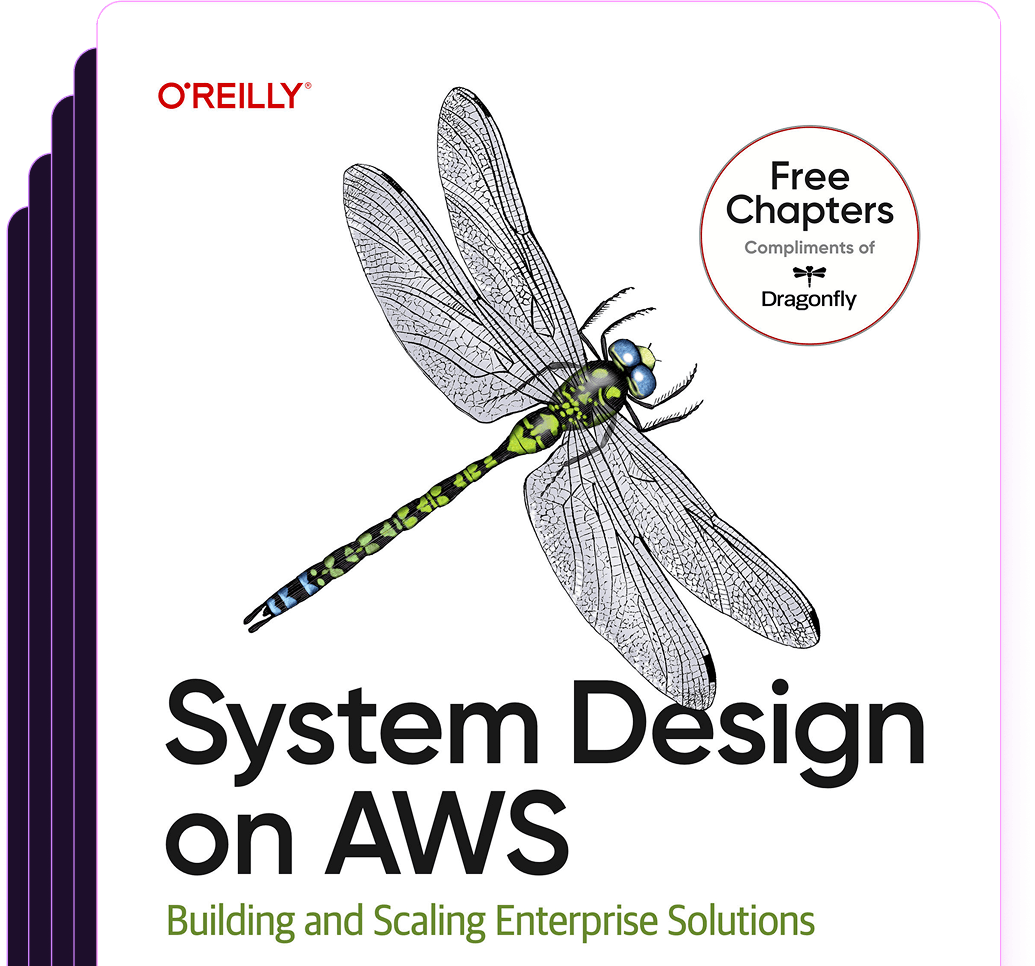
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost