Getting Hash Values at a Key in Redis using Python (Detailed Guide w/ Code Examples)
Use Case(s)
Using a hash in Redis is common when you need to group related key-value pairs under a single key. This might be useful, for example, when storing an object with multiple fields (like a user with username
, email
, etc). The keyword 'python redis get hash values at key' refers to retrieving all the values of a hash stored at a specific key.
Code Examples
In Python, we can use the redis-py
library's hgetall()
method to retrieve all fields and their values from a hash stored at a specific key. Here's how:
import redis
# Establish a connection
r = redis.Redis(host='localhost', port=6379, db=0)
# Set some hash values
r.hset('user:1000', 'username', 'john_doe')
# Get hash values at key
user_values = r.hgetall('user:1000')
print(user_values)
This will output:
{b'username': b'john_doe'}
Which is a dictionary where both keys and values are byte strings. To get them as normal strings, you might want to decode them:
user_values_decoded = {k.decode('utf-8'): v.decode('utf-8') for k, v in user_values.items()}
print(user_values_decoded)
This results in:
{'username': 'john_doe'}
Best Practices
- While using Redis, always remember to close the connection when you're done. Using
with
statement is a good practice as it automatically closes the connection. - Also, handle exceptions that might arise during network operations and provide appropriate fallback mechanisms.
Common Mistakes
- Not checking if the key exists before trying to retrieve its value. If the key does not exist,
hgetall()
will return an empty dictionary. - Forgetting that keys and values retrieved from Redis are byte strings. Always decode them to normal strings if necessary.
FAQs
- What happens if the specified key does not exist in Redis? The
hgetall
function will return an empty dictionary. - Are the keys case-sensitive in Redis? Yes, Redis keys are case-sensitive.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Getting All Keys Matching a Pattern in Redis using Python
- Getting the Length of a Redis List in Python
- Getting Server Info from Redis in Python
- Getting Number of Connections in Redis Using Python
- Getting Current Redis Version in Python
- Getting Memory Stats in Redis using Python
- Redis Get All Databases in Python
- Redis Get All Keys and Values in Python
- Retrieving a Key by Value in Redis Using Python
- Python Redis: Get Config Settings
- Getting All Hash Keys Using Redis in Python
- Get Total Commands Processed in Redis Using Python
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
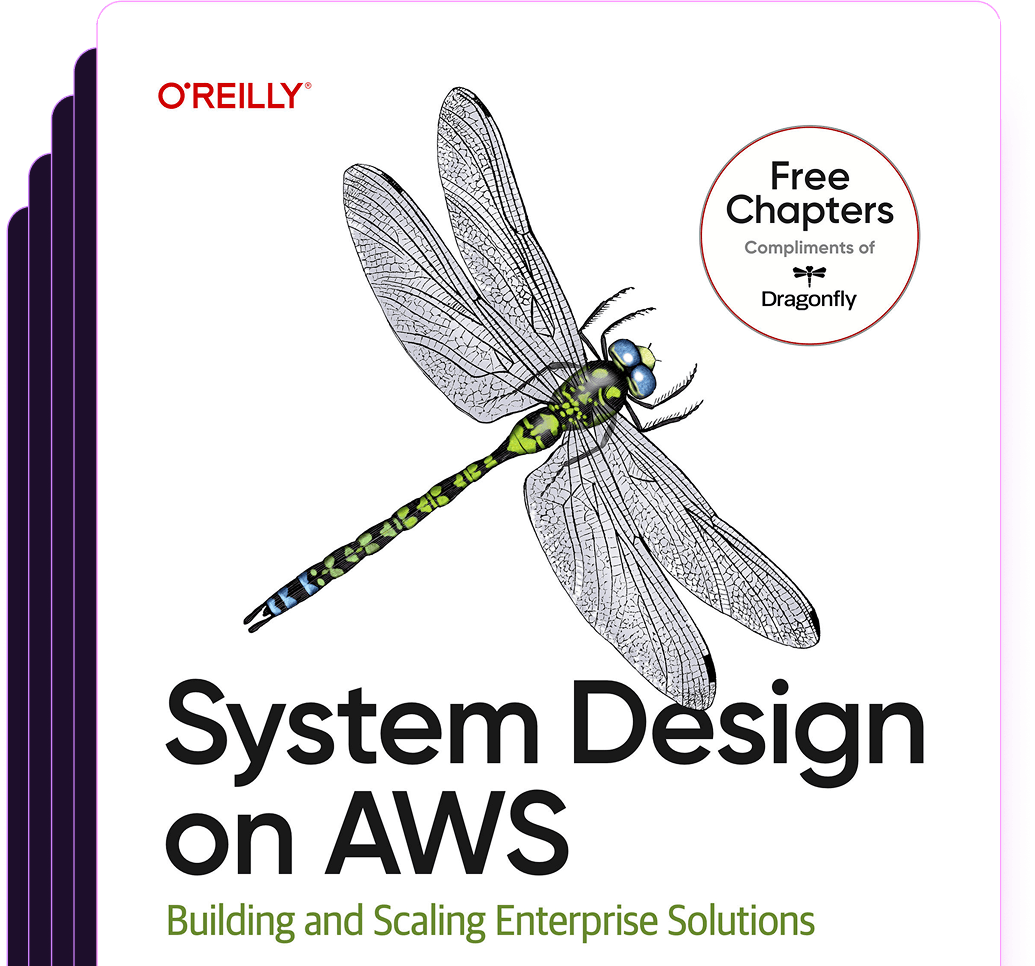
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost