Redis HDEL in Python (Detailed Guide w/ Code Examples)
Use Case(s)
The HDEL
command in Redis is used to delete one or more fields from a hash stored at a key. It's commonly used when you need to remove specific data points from a large dataset stored in a Redis Hash.
Code Examples
Here's a simple example using the redis-py
library in Python: CODE_BLOCK_PLACEHOLDER_0
In this example, we first create a connection to the local Redis server. Then, a hash with key user:1000
is created with three fields - 'name', 'age', and 'email'. The hdel
command is used to delete the 'email' field from our hash. The remaining fields are then printed to the console.
Best Practices
- Be sure to always check if the key exists before trying to delete a field from it.
- Keep track of your fields and have a system for naming them to ensure you don't accidentally delete necessary data.
Common Mistakes
- Trying to delete a field from a non-existing key, which will result in a
None
response. - Deleting a field from a key that holds data types other than hash, it can lead to unexpected behavior.
FAQs
1. What if the field does not exist in the hash? If the specified field does not exist in the hash or the key does not exist, HDEL
will simply ignore it and return 0.
2. Can I delete multiple fields at once? Yes, HDEL
supports deletion of multiple fields at once. Just pass the fields as additional arguments: r.hdel('user:1000', 'field1', 'field2', ...)
.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
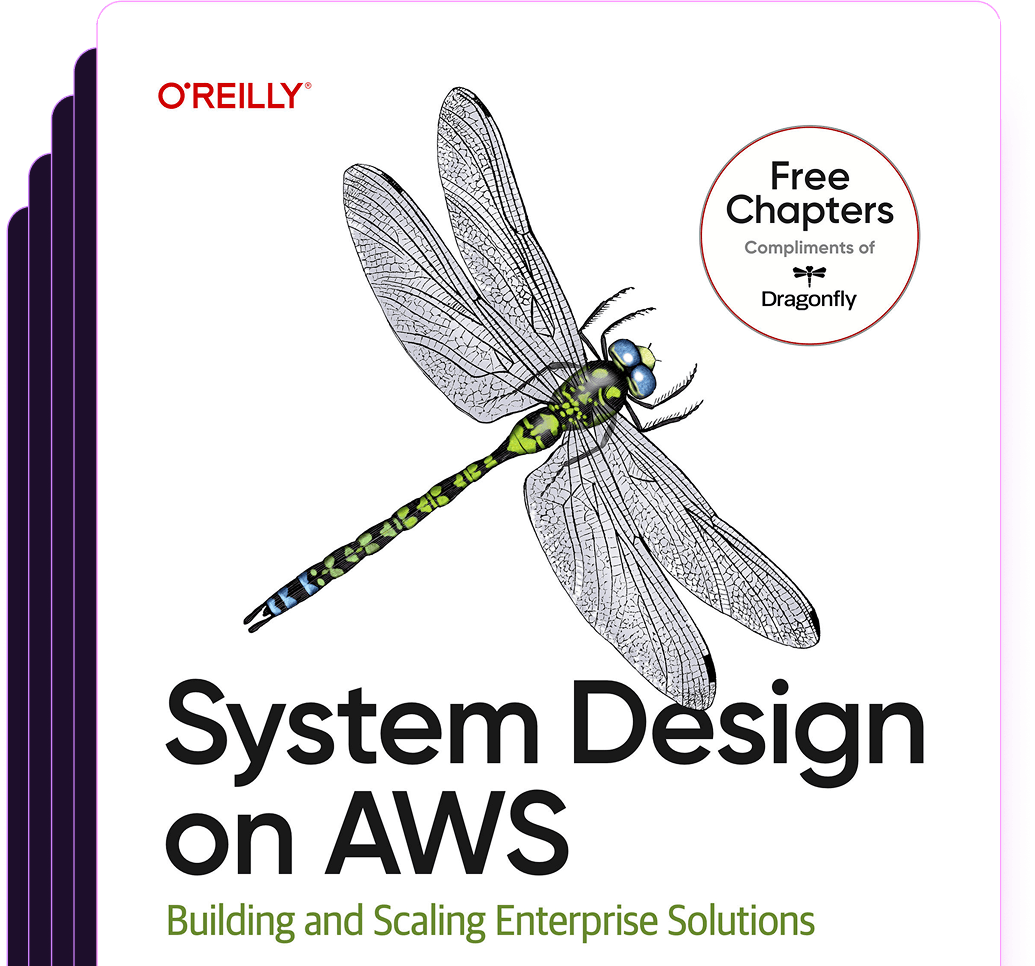
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost