Redis HSET in Python (Detailed Guide w/ Code Examples)
Use Case(s)
The HSET
command is used in Redis to set a field in a hash stored at a specific key. Some common use cases for HSET
include:
- Storing complex data structures, such as objects, as it allows you to associate multiple fields and values within a single Redis key.
- Tracking user information or metadata in web applications, where each field can represent a different attribute of the user.
Code Examples
First, install the redis-py client with pip:
pip install redis
Then, you need to connect to your Redis instance:
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
Now, let's create a hash with the key 'user:1000' and set some fields in it:
r.hset('user:1000', 'name', 'John Doe')
r.hset('user:1000', 'email', 'john.doe@example.com')
r.hset('user:1000', 'password', 's3cr3t')
In these examples, 'user:1000' is the key of our hash. The other parameters are pairs of fields and values.
Best Practices
- It's good practice to use meaningful names for keys and hashes, following a schema that suits your application's logic.
- Be aware that while Redis is pretty fast, involving it in a heavy write traffic could lead to performance issues.
Common Mistakes
- One common mistake is to leave Redis unprotected. You should always password protect your Redis server.
- Another common mistake is not handling exceptions when interacting with Redis. Network errors, for example, can occur at any time and should be properly managed.
FAQs
Q: What happens if the field already exists in the hash? A: If the field already exists, HSET
will update its value.
Q: What if the key does not exist? A: Redis will create a new hash if the key does not exist.
Q: Can I set multiple fields at once? A: Yes, to set multiple fields at once, you can pass a Python dictionary as argument:
r.hset('user:1000', mapping={'field1': 'value1', 'field2': 'value2'})
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
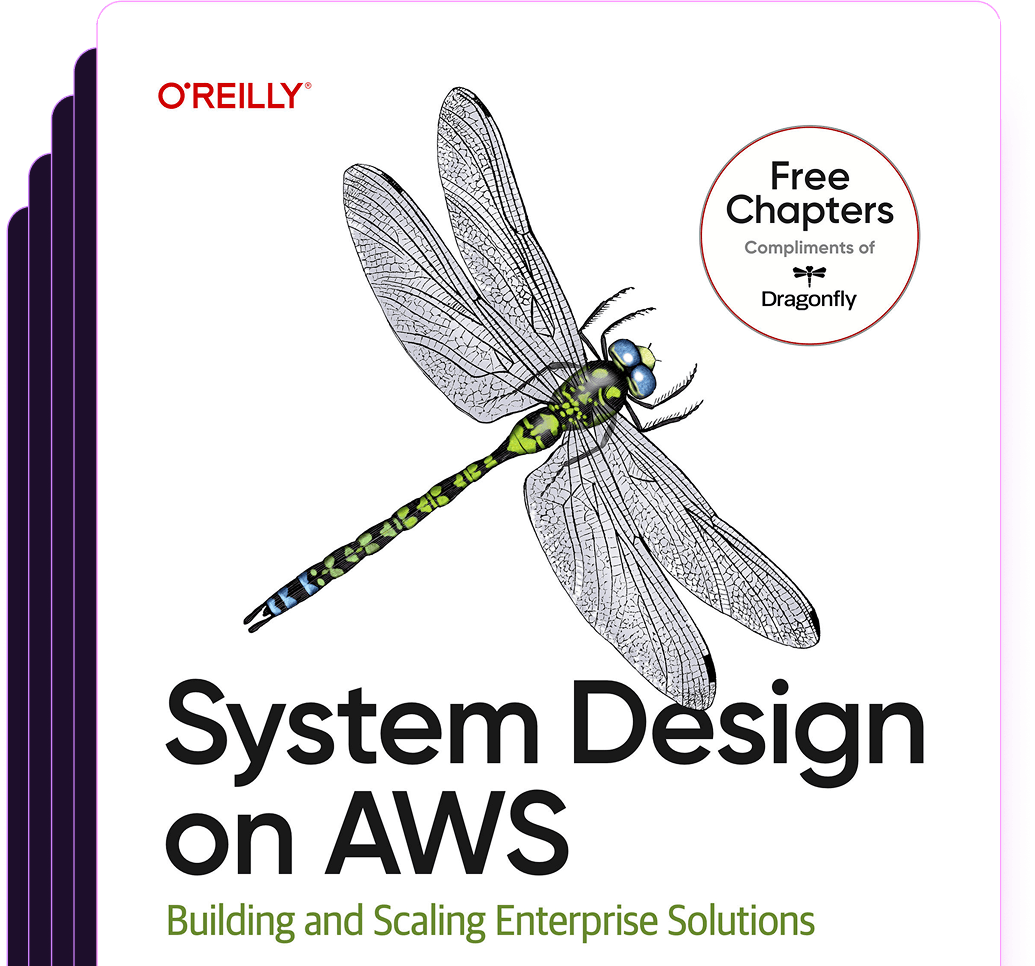
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost