Redis HEXISTS in Python (Detailed Guide w/ Code Examples)
Use Case(s)
The HEXISTS
command in Redis is used to determine if a specific field is an existing key in a hash. Common use cases are for checking the existence of data before performing operations such as updates or deletes, and for conditional logic in scripts or applications.
Code Examples
Let's assume we have Redis running in localhost and a hash named "user:1000" containing fields like "name", "email".
Here's how you can use the HEXISTS function with python and redis-py:
import redis
# Connect to local Redis instance
r = redis.Redis(host='localhost', port=6379, db=0)
# Check if the 'email' field exists
result = r.hexists("user:1000", "email")
print(result) # Prints True if exists, False otherwise
In this example, r.hexists("user:1000", "email")
checks if the field email
exists in the hash user:1000
. It returns True if it exists, False otherwise.
Best Practices
- Always check for the existence of a hash field with
HEXISTS
before trying to access it. Proper handling of non-existing keys will prevent unexpected results. - Use Redis hashes when there are logical groups of keys that belong together. This can lead to more efficient memory usage compared to using individual keys.
Common Mistakes
- Trying to perform operations on non-existing keys without checking for their existence first. This may lead to errors or unexpected behavior.
- Misunderstanding the return value.
HEXISTS
returns 1 (True in Python) if the field exists in the hash, and 0 (False in Python) if the field does not exist or hash does not exist.
FAQs
1. What does HEXISTS
return if the specified hash doesn't exist?
It returns 0 (False in Python). It means either the hash or the field does not exist.
2. Is it necessary to use HEXISTS
before using HGET
or HSET
?
No, it's not necessary but it is a good practice to check whether a field exists before trying to access or modify it.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
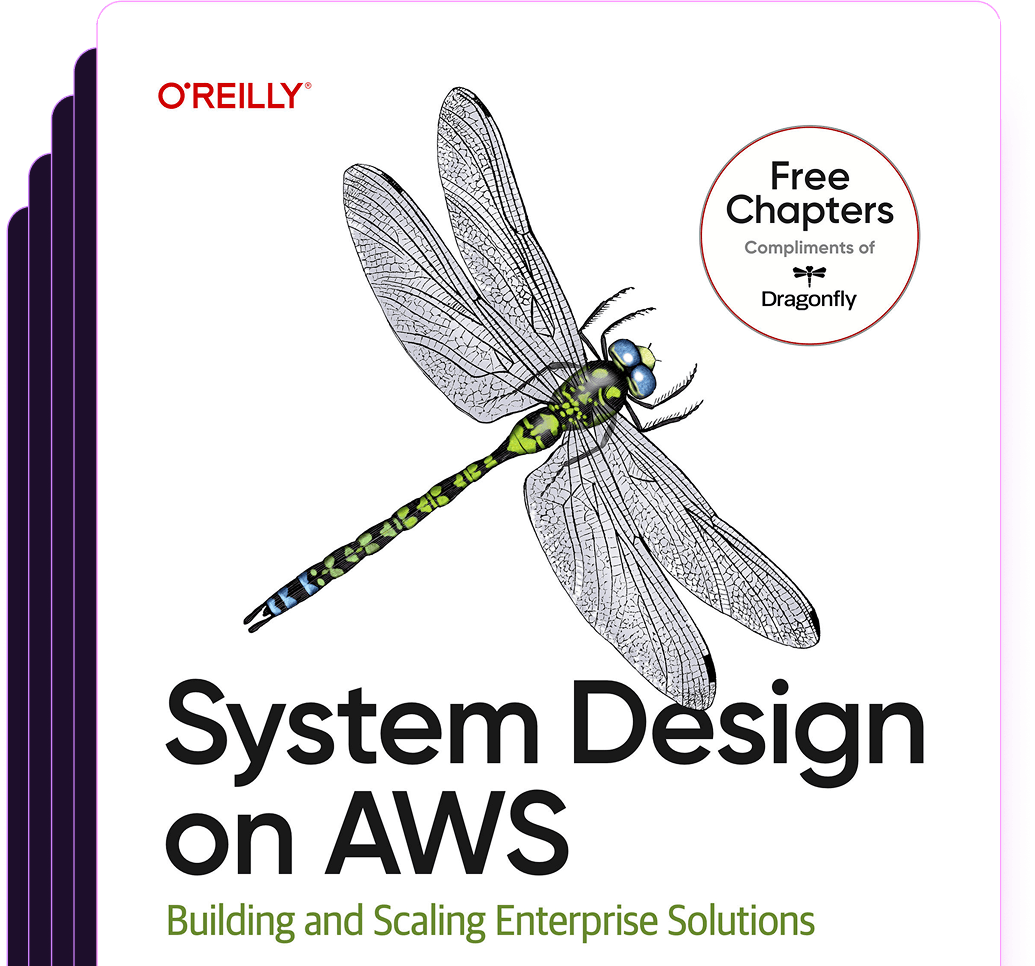
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost