Redis HMGET in Python (Detailed Guide w/ Code Examples)
Use Case(s)
The HMGET
command in Redis is commonly used when there's a need to retrieve multiple field values from a hash stored at a specific key. In the context of Python, Redis' HMGET
is often used in applications that require high-speed access to structured data like user profiles, stateful data, or cached web pages.
Code Examples
Let's say we have a hash representing a User, with fields like "name", "email", and "age". You can use HMGET
to fetch multiple fields at once.
Here's an example using the redis-py library:
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
# Set some initial values
r.hmset("user:1000", {"name": "Jack", "email": "jack@example.com", "age": 30})
# Get multiple field values
values = r.hmget("user:1000", "name", "email")
print(values) # Output: [b'Jack', b'jack@example.com']
The hmget
method takes the key of the hash and the field names you want to retrieve. It returns a list of values corresponding to the given field names.
Best Practices
- Make sure the fields you are getting actually exist within the hash. If a specified field does not exist in the hash or the key does not exist,
HMGET
will return aNone
value for that field. - Use
HMGET
instead of multipleHGET
commands if you need to get multiple fields from a hash. It reduces the number of round-trip times between your application and Redis server.
Common Mistakes
- Using
HMGET
on non-hash keys: If the key exists in the database but its associated value is not a hash, an error will be returned. - Not checking for
None
values in the return list: If a field does not exist in the hash,HMGET
will returnNone
for that field.
FAQs
Q: Can I use HMGET to get all fields from a hash? A: No, HMGET
requires you to specify the fields you want to retrieve. If you want to get all fields and their values, use HGETALL
.
Q: What if the specified key does not exist in Redis? A: If the key does not exist, HMGET
simply returns None
values for all requested fields.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
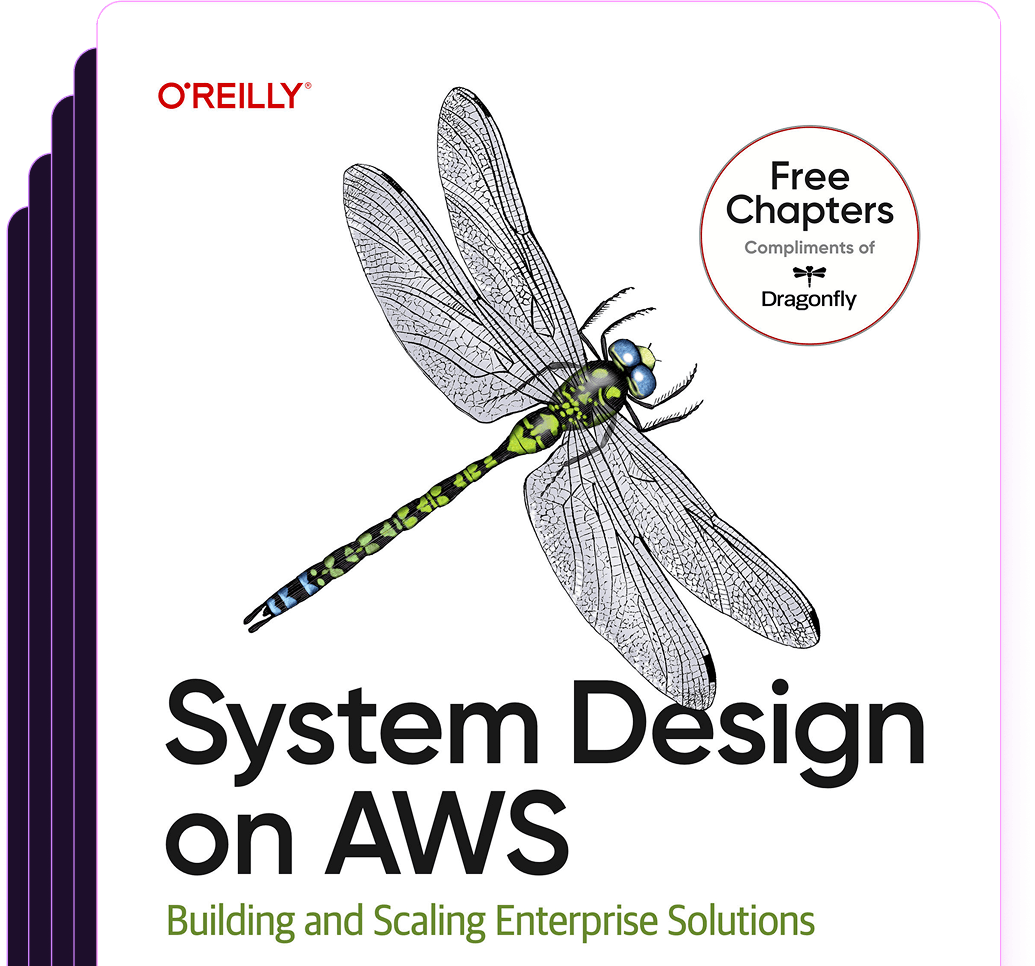
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost