Redis HGETALL in Python (Detailed Guide w/ Code Examples)
Use Case(s)
The hgetall()
method in Redis is commonly used when:
- Retrieving all fields and values of a hash stored at a key.
- Implementing features that require fetching complete data structures, such as user profiles, configuration settings, or any other form of metadata.
Code Examples
Example 1: Fetching All Fields and Values in a Hash
import redis
# create a connection to the Redis server
r = redis.Redis(host='localhost', port=6379, db=0)
# set a hash with multiple fields
r.hmset("user:1001", {"name": "John", "email": "john@example.com", "age": 25})
# get all fields and values of the hash
user_data = r.hgetall("user:1001")
# print the user data
print(user_data)
In this example, we first connect to the Redis server, then we use the hmset()
function to set a hash with multiple fields for user:1001
. The hgetall()
function is used to fetch all fields and values of the hash associated with user:1001
.
Best Practices
- Be aware that the
hgetall()
operation can be expensive if the size of the hash is large, as it retrieves all fields and their values. Use it judiciously to avoid performance issues. - Always check if the key exists before calling
hgetall()
to prevent unexpected results and errors.
Common Mistakes
- Misunderstanding the return value of
hgetall()
: It returns a dictionary containing all fields and corresponding values, not a list of keys. - Assuming that
hgetall()
will return fields in the order they were inserted: Redis doesn't preserve insertion order in hashes.
FAQs
- What happens if the specified key does not exist?
hgetall()
will return an empty dictionary if the key does not exist.
- Can I use
hgetall()
for data types other than hashes in Redis?
- No,
hgetall()
is specifically designed for hash data type. Using it on other types will result in a error.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
White Paper
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
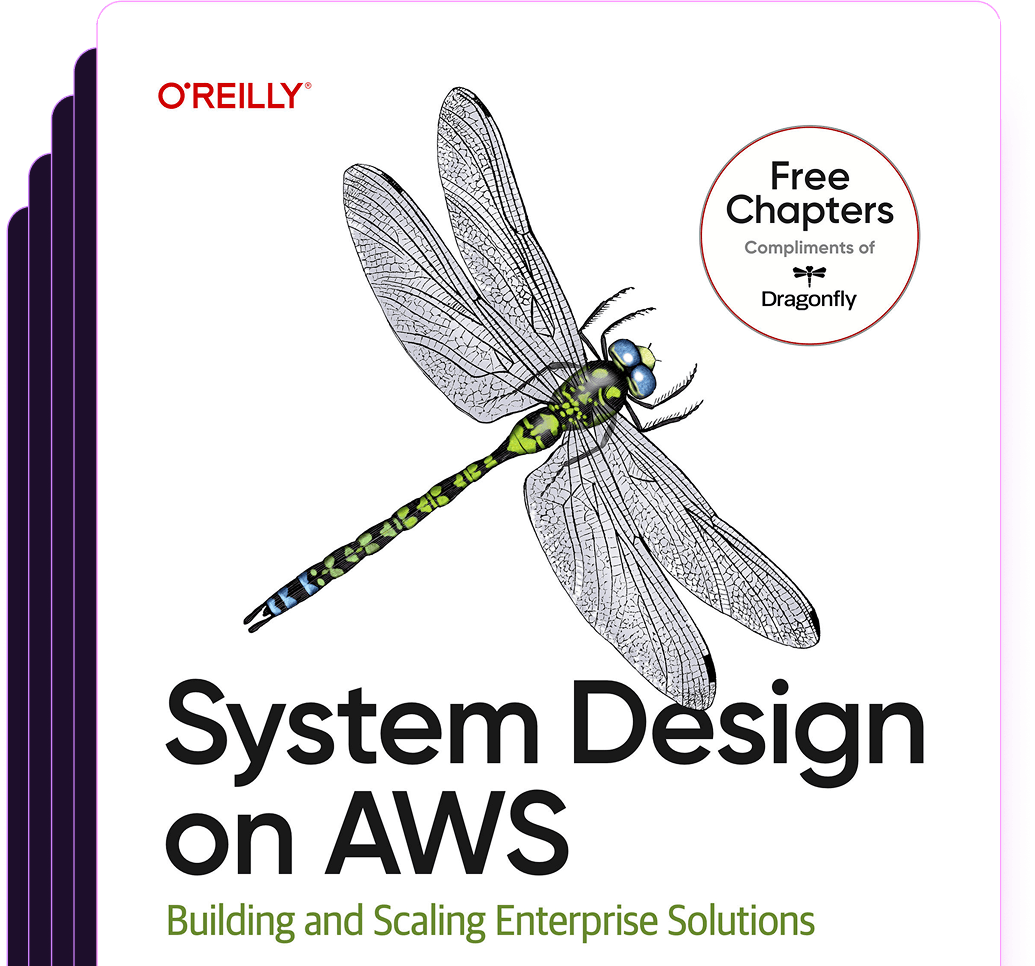
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost