Redis HKEYS in Python (Detailed Guide w/ Code Examples)
Use Case(s)
The HKEYS
command in Redis is typically used when you want to fetch all the fields (keys) present in a particular hash. It's useful when dealing with data structures where each field represents some sort of attribute and you need to know what attributes are available.
Code Examples
Let's consider a scenario where we have a hash named user
that stores basic user details like name, email, and age. Here's how you can get all the keys from this hash using python's redis package.
import redis
# Create a connection to the Redis server
r = redis.Redis(host='localhost', port=6379, db=0)
# Set some fields in a hash
r.hset("user", "name", "Alice")
r.hset("user", "email", "alice@example.com")
r.hset("user", "age", 25)
# Get all the keys of the hash
keys = r.hkeys("user")
print(keys) # Output: [b'name', b'email', b'age']
In this example, hkeys
returns all the fields in the hash named "user". The returned values are byte strings, so if you require them as normal strings, you might need to decode them accordingly.
Best Practices
- Use hashes when you want to group related data together under a single key.
- Avoid using
HKEYS
on large hashes in a production environment as it may lead to high latency. If you must fetch keys from large hashes, consider scanning the hash incrementally instead of loading all keys at once.
Common Mistakes
Not being aware that HKEYS
can lead to high latency when used on large hashes. This command may block the server for a long time when running against large hashes by consuming a lot of CPU and memory.
FAQs
Q: What data does HKEYS
return? A: The HKEYS
command returns all field names (keys) in the hash stored at a specified key.
Q: What happens if the specified key doesn't exist? A: If the provided key doesn't exist, HKEYS
will return an empty list.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
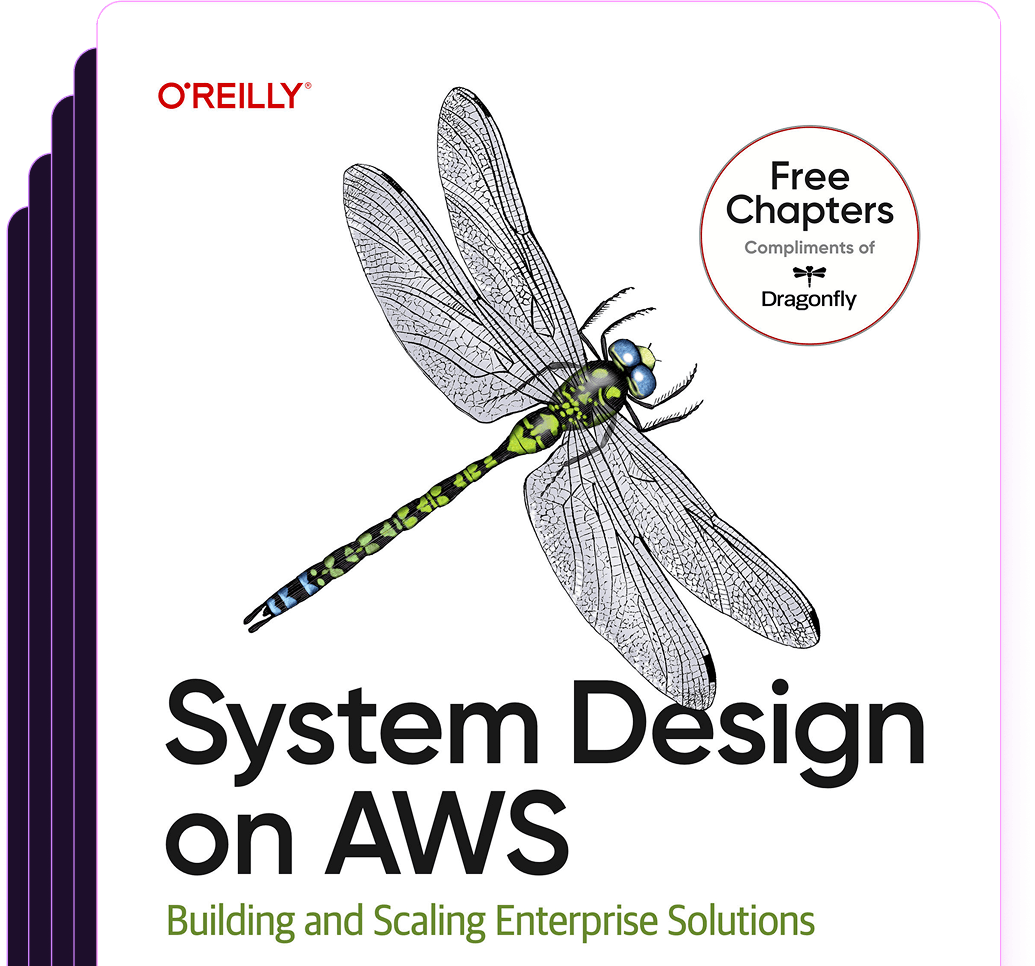
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost