Redis XREADGROUP in Python (Detailed Guide w/ Code Examples)
Use Case(s)
XREADGROUP
is a command used in Redis Streams. It's primarily used when you want to distribute the stream of messages among multiple consumers (or consumer groups). This allows for better scalability and reliability in processing streams.
Code Examples
Below are Python examples using redis-py
, a Python client for Redis.
Example 1: Creating a group and reading from it
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
# Creating a stream 'mystream' if doesn't exist.
r.xadd('mystream', {'field': 'value'})
# Creating a group named 'mygroup' with consumer 'myconsumer'
r.xgroup_create('mystream', 'mygroup', id='0')
# Reading from group
messages = r.xreadgroup('mygroup', 'myconsumer', {'mystream': '>'}, count=1)
print(messages)
This code first creates a stream and adds a message to it. Then it creates a consumer group. The 'mygroup' consumer group is created at the start of the mystream ('0'). Finally, it reads a message from the group with the xreadgroup
command.
Example 2: Acknowledging a message
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
# Assume the stream and group are already created
# Reading from group and acknowledging
message_id, message = r.xreadgroup('mygroup', 'myconsumer', {'mystream': '>'}, count=1)[0]
r.xack('mystream', 'mygroup', message_id)
In this example, after reading the message from the stream, the message is acknowledged using xack
. This informs Redis that the message has been processed and it can be safely deleted when needed.
Best Practices
- Always acknowledge messages after processing. Unacknowledged messages might be delivered again in case of a failure.
- Use the 'count' option of the
xreadgroup
function to limit the number of messages read at once. This can help manage memory usage.
Common Mistakes
- Not creating groups before reading from them.
- Not acknowledging messages which leads to repeated delivery of the same messages.
FAQs
Q: What does the '>' mean in xreadgroup command? A: The '>' symbol tells Redis to return the new messages that are arriving in the stream after the consumer’s last ID.
Q: How does Redis handle unacknowledged messages? A: Redis will re-deliver these messages to any available consumer requesting data from the stream. This ensures no data loss in case of consumer failures.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
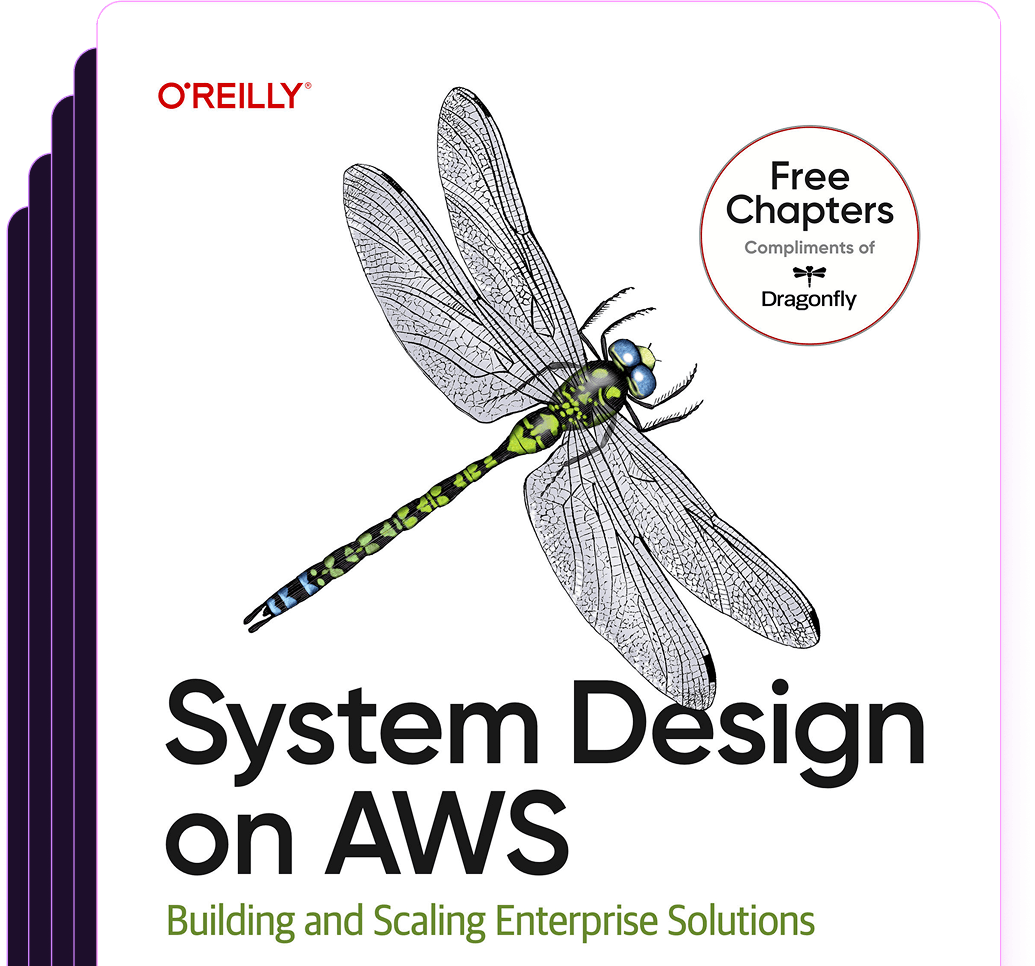
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost