Redis XACK in Python (Detailed Guide w/ Code Examples)
Use Case(s)
The XACK
command in Redis is used when working with Redis Streams. It's a way to acknowledge that a message has been processed. This comes into play in consumer groups, where multiple consumers might be processing messages from the same stream.
Code Examples
Here's an example of how you can use XACK
:
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
stream_name = 'mystream'
group_name = 'mygroup'
consumer_name = 'myconsumer'
# Add some data to the stream
r.xadd(stream_name, {'field1': 'value1'})
# Create a new consumer group if it doesn't exist yet
if not r.xgroup_create(stream_name, group_name, mkstream=True):
print('Consumer group already exists')
# Read the data using xreadgroup command
messages = r.xreadgroup(group_name, consumer_name, {stream_name: '>'}, count=1)
message_id = messages[0][1][0][0]
# Acknowledge the data using xack command
r.xack(stream_name, group_name, message_id)
In this example, we first add some data to the Redis Stream and then create a new consumer group. Then we read one message from the stream using the xreadgroup
command and finally acknowledge that message using xack
.
Best Practices
It's important to handle exceptions when working with XACK
, especially in a distributed environment where network issues can occur. Always ensure you confirm that your message has been acknowledged.
Common Mistakes
One common mistake when working with XACK
is forgetting to acknowledge a message after it's been processed. If a message isn't acknowledged, Redis will continue to consider it unprocessed and it might be delivered again in the future.
FAQs
Q: What happens if I don't acknowledge a message? A: If you don't acknowledge a message, it remains as an unprocessed message. Depending on your application's configuration, it might be delivered again in the future.
Q: Can I acknowledge multiple messages at once? A: Yes, you can pass multiple message IDs to the XACK
command to acknowledge them all at once.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
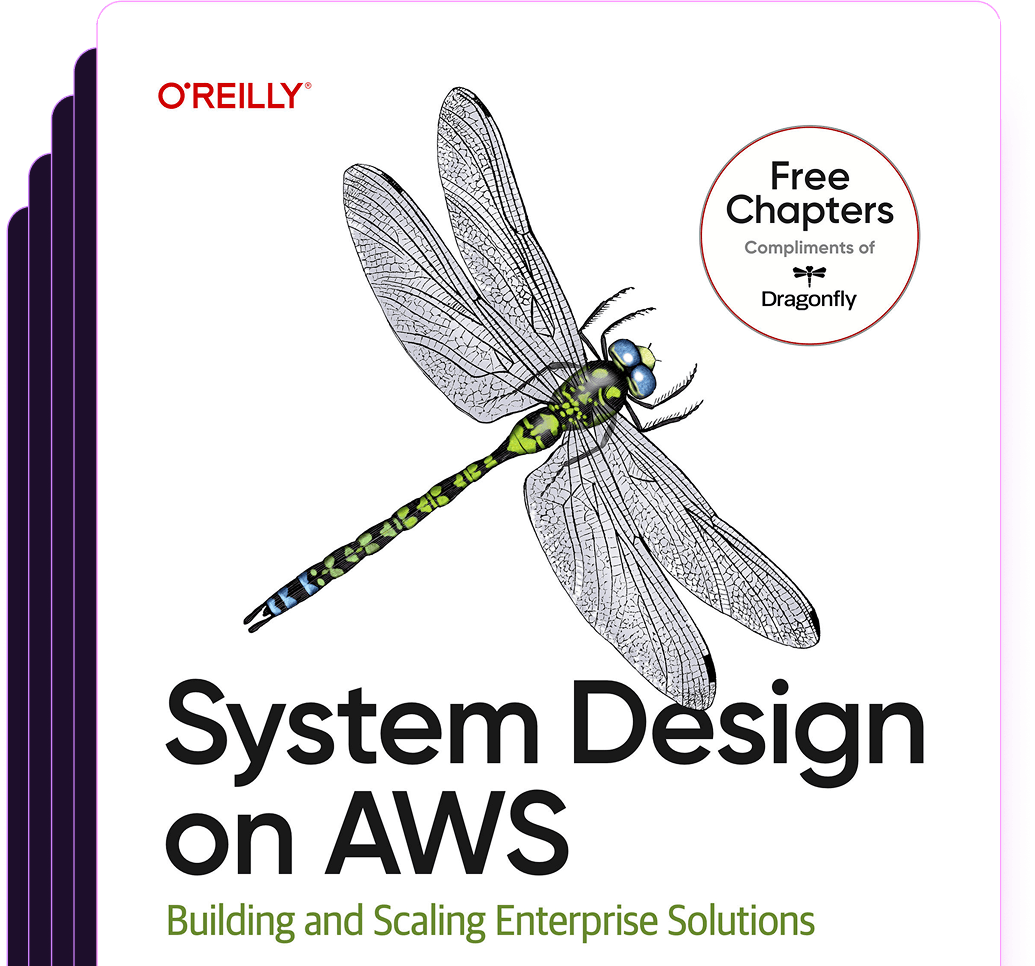
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost