Redis XREAD in Python (Detailed Guide w/ Code Examples)
Use Case(s)
The XREAD
command in Redis is used for reading data from a stream. It's commonly used in scenarios where you have real-time data being generated and consumed, such as a chat system, user activity tracking, logging systems, or any other producer-consumer problems.
Code Examples
Here is an example of using XREAD
with redis-py, the Python client for Redis:
import redis
# establish a connection to Redis
r = redis.Redis(host='localhost', port=6379, db=0)
# add some data to a stream
for i in range(10):
r.xadd("mystream", {"field": f"value{i}"})
# read data from the stream
messages = r.xread({"mystream": "0-0"}, count=5, block=1000)
for message in messages:
print(message)
In this example, we first establish a connection to Redis, then create a stream called 'mystream' and add 10 messages to it. We then use xread
to read up to 5 messages from the stream, waiting up to 1 second (1000 ms) if no messages are immediately available.
Best Practices
- While using blocking reads (
block
parameter inxread
), be aware that the calling thread will be blocked until there are items to read or the timeout is reached. This can impact application performance if not managed properly. XREAD
allows you to specify aCOUNT
option which provides control over the number of elements fetched per call. Be mindful of memory usage when setting a high count value.
Common Mistakes
- Not specifying the last ID (e.g. '0-0' or '$') when calling
xread
might result in reading from an unintended place in the stream. - Not handling the case when
xread
returns an empty list (when it times out), which can lead to unexpected behavior.
FAQs
Q: What does the "0-0" mean in the call to xread
?
A: The "0-0" is a special ID that represents the start of the stream. By passing this as the ID, we're telling Redis we want to read from the start of the stream.
Q: What does the block
parameter do in xread
?
A: The block
parameter specifies how long xread
should block if there are no items to read in the stream. It's specified in milliseconds.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
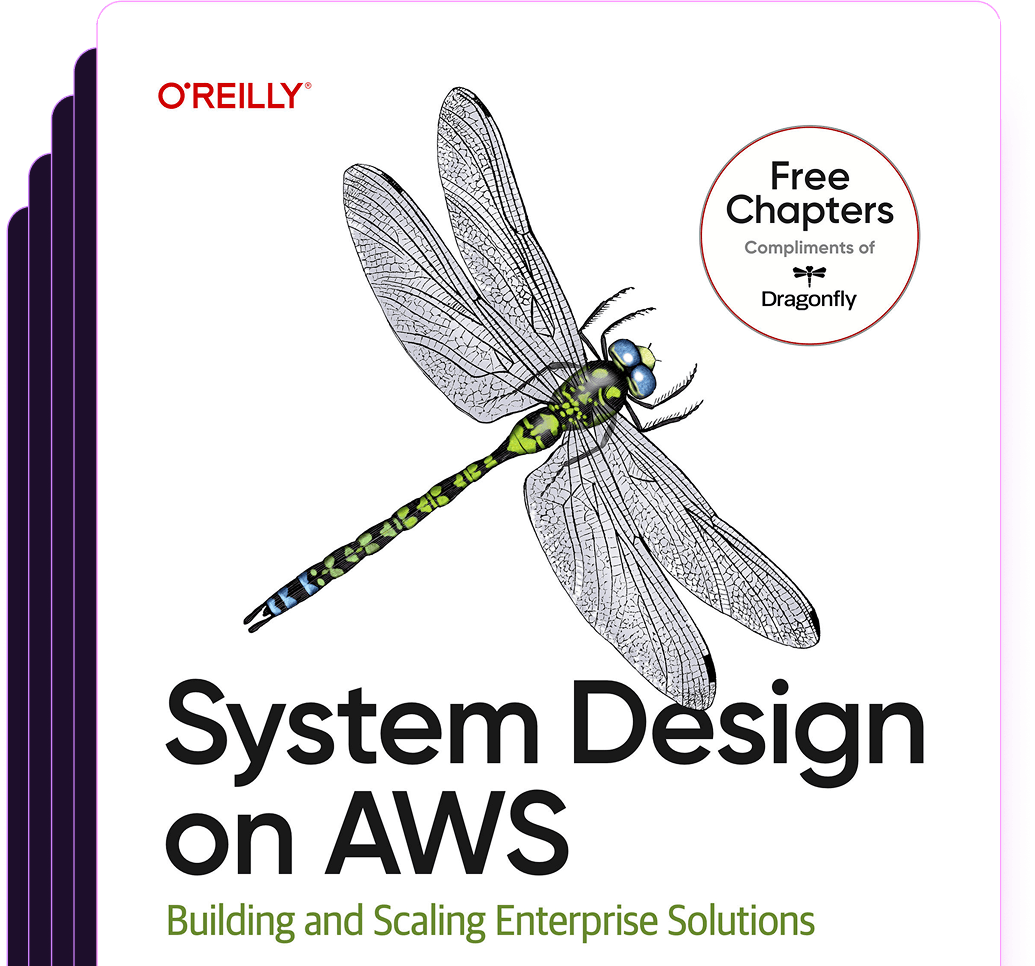
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost