Question: Does Godot support C++?
Answer
Yes, Godot supports C++. While Godot's primary scripting language is GDScript, which is designed to be easy to pick up for beginners, the engine also supports several other languages for scripting, one of which is C++.
Godot provides a way to write game logic in C++ through a feature called GDNative. GDNative allows users to load compiled C++ code as a native script. This can lead to performance benefits and give developers access to all features and optimizations their C++ compilers provide.
Here's how you might typically set up a C++ project to work with Godot using GDNative:
- Setup a C++ Project: Create your C++ project structure with source files and a SConstruct file if you are using SCons as your build system.
- Write Your Code: Write your C++ classes, inheriting from
godot::GodotScript<T>
where T is the type of Node that you want to extend (for instance,godot::GodotScript<godot::Sprite>
to create a custom sprite class).
#include <Godot.hpp>
#include <Sprite.hpp>
namespace godot {
class MySprite : public GodotScript<Sprite> {
GODOT_CLASS(MySprite, Sprite)
public:
static void _register_methods() {
register_method("_process", &MySprite::_process);
}
void _init() {
// Initialization code here.
}
void _process(float delta) {
// Frame update code here.
}
};
// Don't forget to register the class!
extern "C" void GDN_EXPORT godot_gdnative_init(godot_gdnative_init_options *o) {
Godot::gdnative_init(o);
}
extern "C" void GDN_EXPORT godot_gdnative_terminate(godot_gdnative_terminate_options *o) {
Godot::gdnative_terminate(o);
}
extern "C" void GDN_EXPORT godot_nativescript_init(void *handle) {
Godot::nativescript_init(handle);
register_class<MySprite>();
}
}
- Compile Your Code: Compile your C++ code into a shared library (
.dll
on Windows,.so
on Linux, or.dylib
on macOS). - Create GDNative Scripts: In your Godot project, create .gdns files that point to the compiled libraries and use the native scripts as you would typically use a GDScript.
- Attach GDNative Scripts to Nodes: Attach the
.gdns
files to nodes in your scene.
By following this process, you can develop parts or all of your application in C++ while still taking advantage of Godot's engine features. It is important to note that interfacing with the engine this way is more complex than using GDScript and not typically recommended for beginners. However, it allows for high-performance code and the use of external C++ libraries in your Godot project, which can be critical for certain applications.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Engines Questions (and Answers)
- Can You Use C# in Unreal Engine?
- Is Unreal Engine Open Source?
- Can Unreal Engine make 2D games?
- Does Unreal Engine Use C++?
- Does Unreal Engine Use Python?
- What Language Does Unreal Engine Use?
- Does Unreal Engine Use JavaScript?
- How Do I Uninstall Unreal Engine 5?
- Is Blender or Unreal Engine Better?
- Is Unreal Engine Good for Beginners?
- Does Unreal Engine Work on Mac?
- Why Is Unreal Engine So Laggy?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
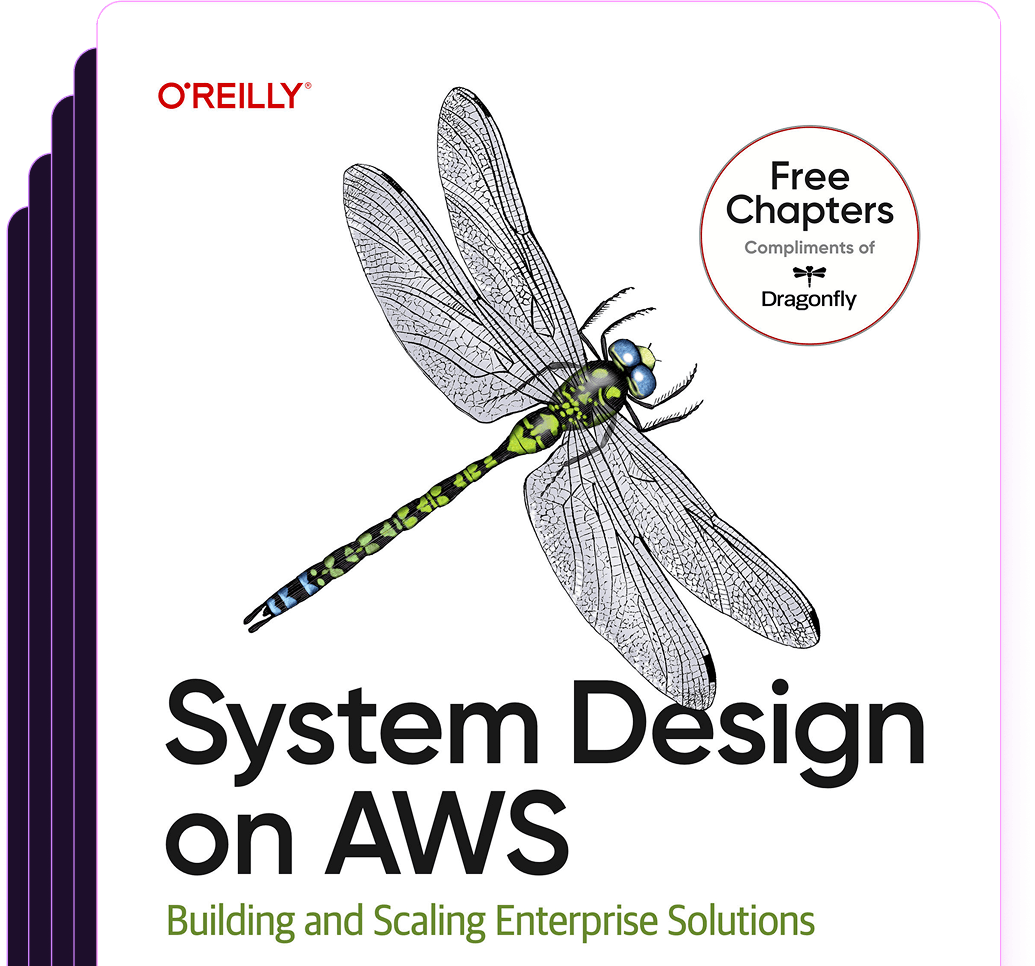
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost