Question: Does Unity Use Python?
Answer
Unity is a popular game development platform known for its ease of use and powerful 3D rendering capabilities. Typically, Unity uses C# as its primary scripting language, which allows developers to interact with the engine and customize their game's behavior.
However, Unity does not natively support Python for game scripting or editor scripting within the engine. While C# remains the standard, there are ways to integrate Python into the Unity workflow for various purposes such as automation, tooling, and data handling through external plugins or by using IronPython, a version of Python that can interact with .NET languages like C#.
For most game development tasks, including gameplay programming, AI, UI, and interaction, you will use C# scripts. Here is a simple example of a C# script in Unity that might control a player's movement:
using UnityEngine;
public class PlayerController : MonoBehaviour
{
public float speed = 5.0f;
void Update()
{
float horizontalInput = Input.GetAxis("Horizontal");
float verticalInput = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(horizontalInput, 0.0f, verticalInput) * speed;
transform.Translate(movement * Time.deltaTime);
}
}
In contrast, if you were able to use Python directly in Unity (which, again, isn't supported natively), the equivalent script might look something like this hypothetically:
import UnityEngine as unity
class PlayerController(unity.MonoBehaviour):
def __init__(self):
self.speed = 5.0
def Update(self):
horizontal_input = unity.Input.GetAxis("Horizontal")
vertical_input = unity.Input.GetAxis("Vertical")
movement = unity.Vector3(horizontal_input, 0.0, vertical_input) * self.speed
self.transform.Translate(movement * unity.Time.deltaTime)
Despite the hypothetical Python code, remember that such integration would require significant effort to work within the Unity environment as it does not provide built-in support for Python scripting.
For specialized tasks or those more comfortable with Python, it may be possible to find or create tools that allow for Python scripts to run outside of Unity and communicate with the Unity engine, but these solutions are generally non-standard and can introduce additional complexity into the development process.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Engines Questions (and Answers)
- Can You Use C# in Unreal Engine?
- Is Unreal Engine Open Source?
- Can Unreal Engine make 2D games?
- Does Unreal Engine Use C++?
- Does Unreal Engine Use Python?
- What Language Does Unreal Engine Use?
- Does Unreal Engine Use JavaScript?
- Does Unreal Engine work on Linux?
- How Do I Uninstall Unreal Engine 5?
- Is Blender or Unreal Engine Better?
- Is Unreal Engine Good for Beginners?
- Does Unreal Engine Work on Mac?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
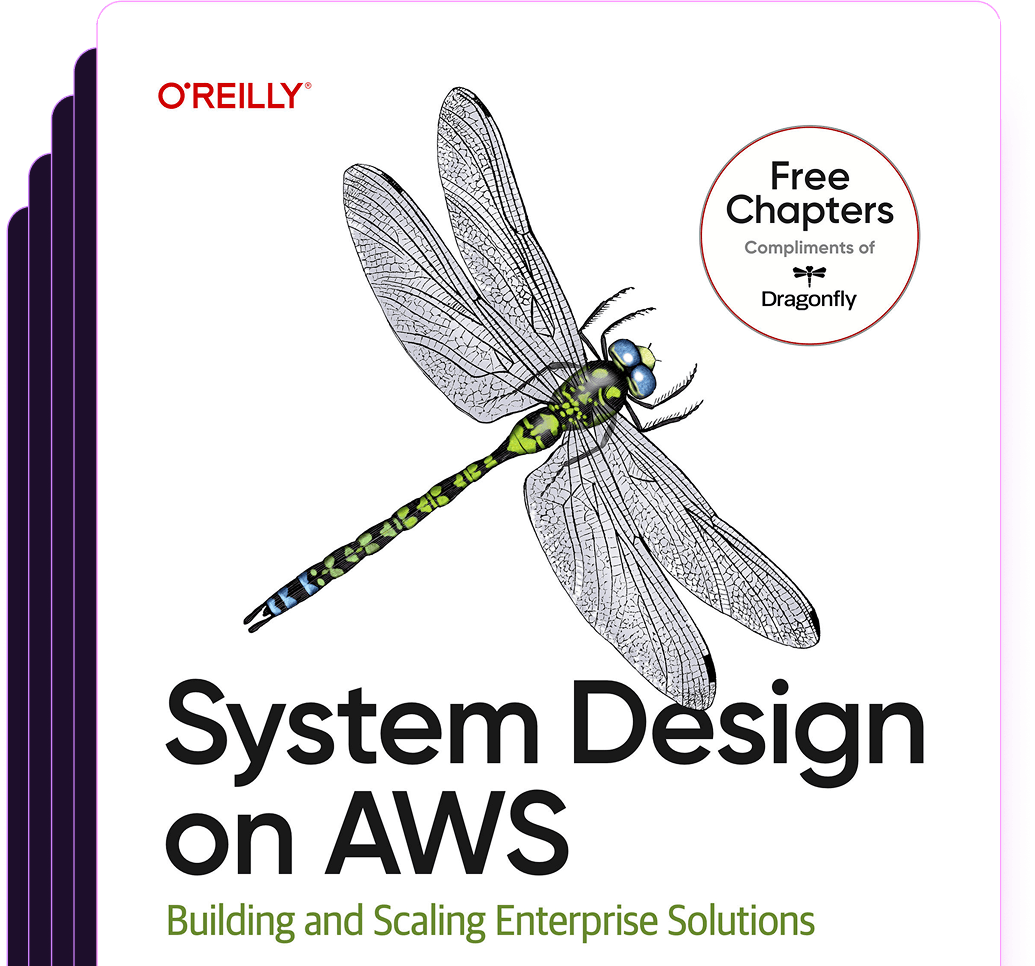
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost