Question: How do you create and use a timer in GameMaker?
Answer
In GameMaker, timers are used to execute code after a certain amount of time has passed. A timer can be implemented using alarms or by manually tracking the passage of time using variables.
Using Alarms for Timers
GameMaker provides a built-in feature called "alarms." Each instance has twelve alarms (from alarm[0]
to alarm[11]
) that can be set to go off after a certain number of steps (frames).
Here's how you set an alarm:
// Set alarm[0] to go off in 2 seconds (assuming room_speed is 60)
alarm[0] = 2 * room_speed;
And this is how you might respond to an alarm:
// In the Alarm 0 Event:
// Code to execute after 2 seconds
instance_destroy(); // As an example, destroy the instance
Creating a Manual Timer
Sometimes you might need more flexibility or additional timers beyond the 12 provided. For that, you can create your own timer using variables and step events.
Here's how to implement a custom timer:
// Create Event of an object:
my_timer = 120; // This is the countdown time in steps
timer_active = true; // Controls whether the timer is running
// Step Event of the same object:
if (timer_active) {
my_timer -= 1; // Decrease the timer by 1 every step
if (my_timer <= 0) {
// Time's up, put your code here
show_message("Timer finished!");
timer_active = false; // Stop the timer
}
}
Remember to manage the state of timer_active
as needed to start or stop the timer.
Delta Timing
For precise timing that takes into account potential variations in frame rate, you can use delta timing. GameMaker has the built-in variable delta_time
, which represents the time since the last step event in microseconds.
Here's an example of using delta_time
for a timer:
// Create Event of an object:
my_timer = 2000000; // 2 seconds in microseconds
// Step Event of the same object:
my_timer -= delta_time;
if (my_timer <= 0) {
// Timer has completed its countdown
show_message("Delta timer finished!");
// Do not forget to reset or deactivate your timer as required
}
Timers are essential for everything from delays between actions, cooldowns on abilities, to animations and transitions. Whether using alarms or creating manual timers, both methods require you to keep track of the passage of time within your game's events and respond accordingly when the time is right.
Alarms are straightforward and integrated into GameMaker but offer limited slots, while custom timers require more coding but give unlimited flexibility. The choice depends on your needs and the complexity of your game.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Engines Questions (and Answers)
- Can You Use C# in Unreal Engine?
- Is Unreal Engine Open Source?
- Can Unreal Engine make 2D games?
- Does Unreal Engine Use C++?
- Does Unreal Engine Use Python?
- What Language Does Unreal Engine Use?
- Does Unreal Engine Use JavaScript?
- How Do I Uninstall Unreal Engine 5?
- Is Blender or Unreal Engine Better?
- Is Unreal Engine Good for Beginners?
- Does Unreal Engine Work on Mac?
- Why Is Unreal Engine So Laggy?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
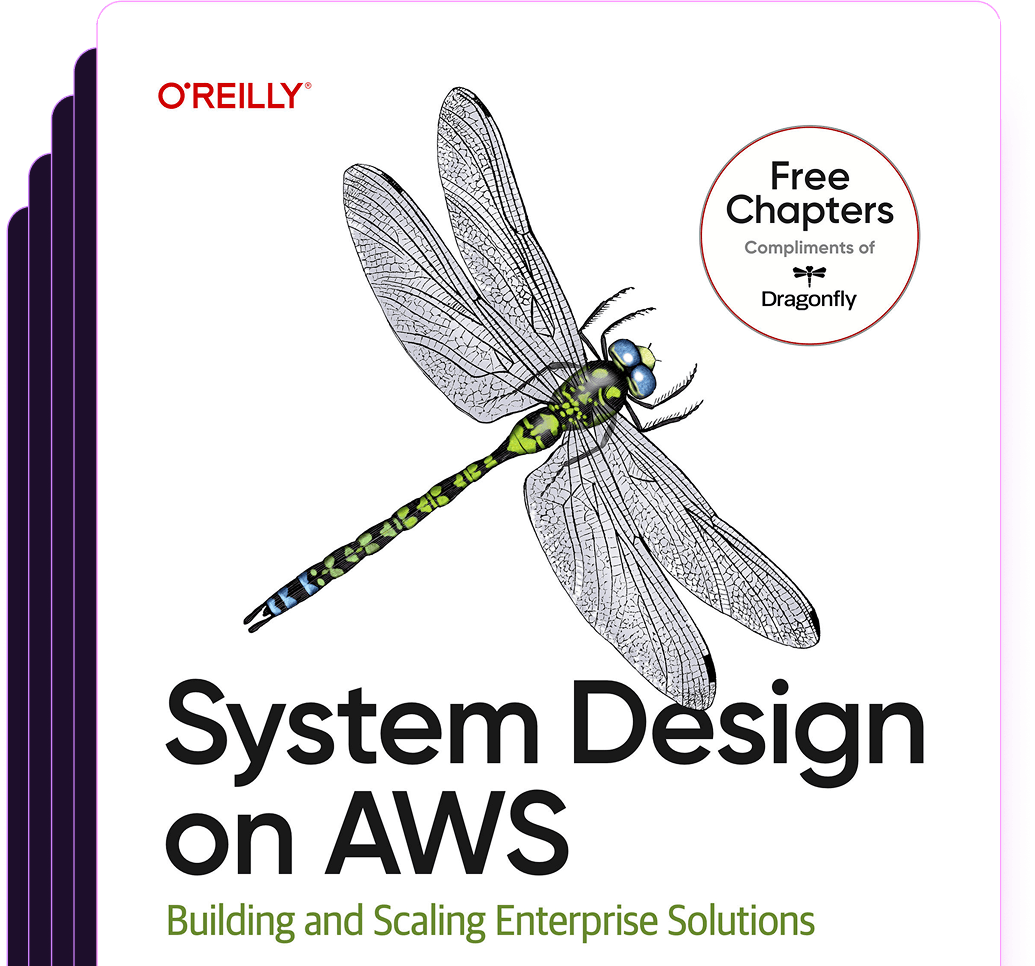
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost