Question: How do you get a variable from another object in GameMaker?
Answer
In GameMaker, you can access variables from other instances (objects) using the dot operator or with specific functions like object_get_variable
or instance_find
. Here’s how you do it:
Using the Dot Operator
If you know the instance ID of the object from which you want to get a variable, you can use the dot operator to access its variables. The instance ID is often obtained when you create an object using instance_create_layer
or instance_create_depth
. For example:
var other_instance_id = instance_create_layer(x, y, "Instances", obj_Enemy);
var enemy_health = other_instance_id.health;
In this code, obj_Enemy
has a variable named health
, and we are accessing it using the instance ID stored in other_instance_id
.
Using Instance Functions
If you don’t have the instance ID but know that there is only one instance of the object in the room, you can use instance_find
to get the instance ID:
var enemy_instance_id = instance_find(obj_Enemy, 0);
var enemy_health = enemy_instance_id.health;
This finds the first instance of obj_Enemy
in the room.
Accessing Variables by Object Index
When there are multiple instances of an object and you want any one of them, you could use the object index directly:
var enemy_health = obj_Enemy.health;
This will get the health
variable from the first instance of obj_Enemy
that was created.
Using with
Statement
You can also use the with
statement to perform operations on all instances of an object. If you just want to retrieve a value once, you should assign it to a variable outside of the with
scope:
var first_enemy_health;
with (obj_Enemy) {
first_enemy_health = health; // Assuming 'health' is a variable within obj_Enemy
break; // This exits the with structure after getting the first instance's health
}
Cautionary Notes
- Ensure that the object or instance you're trying to access the variable from is active and exists in the current context; otherwise, you'll get an error.
- It is good practice to use
instance_exists
before trying to access a variable from another instance to avoid runtime errors.
These are some of the ways you can get a variable from another object in GameMaker. Choose the method that best fits your situation.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Engines Questions (and Answers)
- Can You Use C# in Unreal Engine?
- Is Unreal Engine Open Source?
- Can Unreal Engine make 2D games?
- Does Unreal Engine Use C++?
- Does Unreal Engine Use Python?
- What Language Does Unreal Engine Use?
- Does Unreal Engine Use JavaScript?
- How Do I Uninstall Unreal Engine 5?
- Is Blender or Unreal Engine Better?
- Is Unreal Engine Good for Beginners?
- Does Unreal Engine Work on Mac?
- Why Is Unreal Engine So Laggy?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
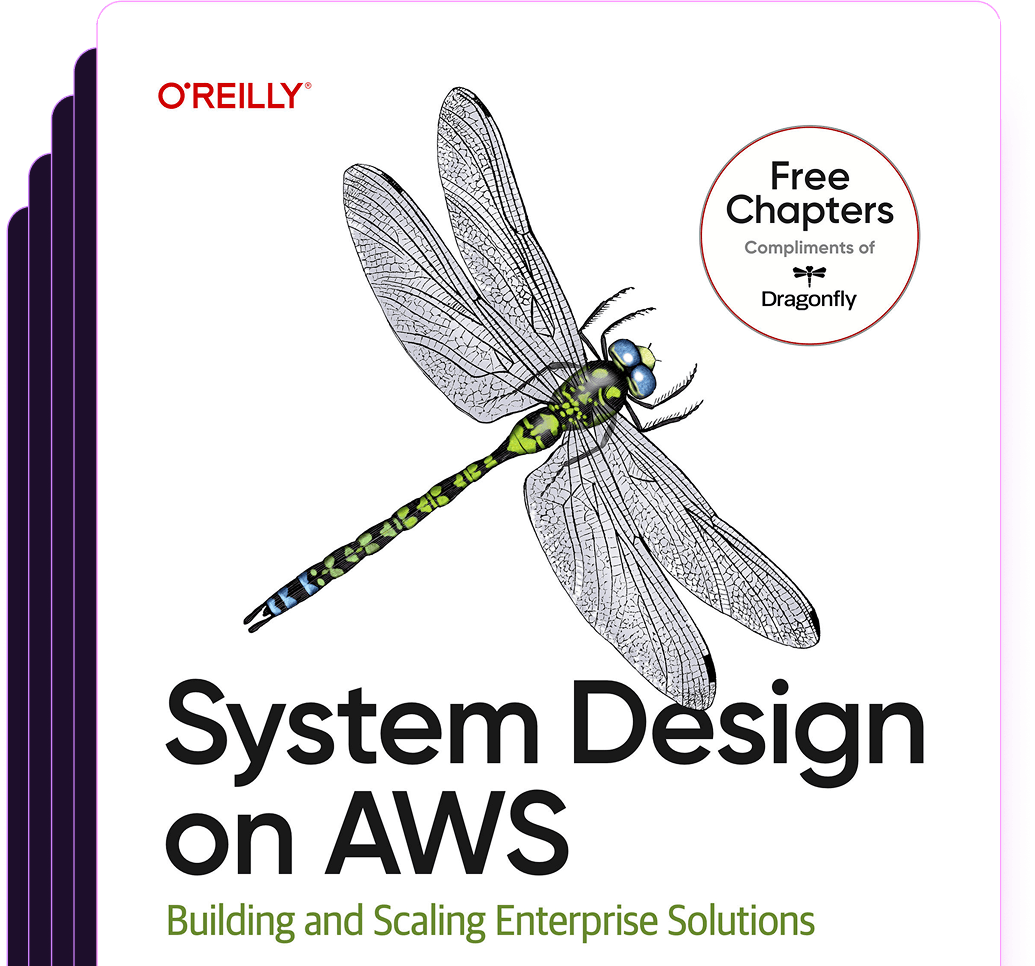
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost