Question: How do you check keyboard input in GameMaker?
Answer
To check for keyboard input in GameMaker, you typically use the function keyboard_check(key)
for continuous checks, or keyboard_check_pressed(key)
and keyboard_check_released(key)
for single events.
Here is how you might use these functions:
// To check if a specific key is being held down (continuous)
if (keyboard_check(vk_left)) {
// Move player left
}
// To check if a specific key has just been pressed (once per press)
if (keyboard_check_pressed(vk_space)) {
// Make player jump
}
// To check if a specific key has just been released
if (keyboard_check_released(vk_right)) {
// Stop moving player to the right
}
In these examples, vk_left
, vk_space
, and vk_right
are virtual key codes representing the left arrow key, spacebar, and right arrow key, respectively. GameMaker provides a wide range of constants for various keys on the keyboard such as vk_up
, vk_down
, vk_enter
, etc.
For custom key mappings or checking alphanumeric keys, you need to provide the appropriate ASCII value or use GameMaker's built-in constants like ord("A")
for the 'A' key:
// Custom key mapping using ASCII values
var shootKey = ord("S");
if (keyboard_check(shootKey)) {
// Shoot action
}
Make sure to place your keyboard check code within an appropriate event such as the Step event of an object, so that it gets executed continuously or whenever you need it to check for key presses.
Additionally, you can also check if any key is pressed using keyboard_check_any()
, which returns true if any key is currently being pressed.
GameMaker also provides keyboard_lastkey
which holds the key code of the last key that was pressed, which can be handy in certain scenarios.
Remember that keyboard input can vary depending on the system the game is running on, so ensure to test your game across different systems if possible.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Engines Questions (and Answers)
- Can You Use C# in Unreal Engine?
- Is Unreal Engine Open Source?
- Can Unreal Engine make 2D games?
- Does Unreal Engine Use C++?
- Does Unreal Engine Use Python?
- What Language Does Unreal Engine Use?
- Does Unreal Engine Use JavaScript?
- How Do I Uninstall Unreal Engine 5?
- Is Blender or Unreal Engine Better?
- Is Unreal Engine Good for Beginners?
- Does Unreal Engine Work on Mac?
- Why Is Unreal Engine So Laggy?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
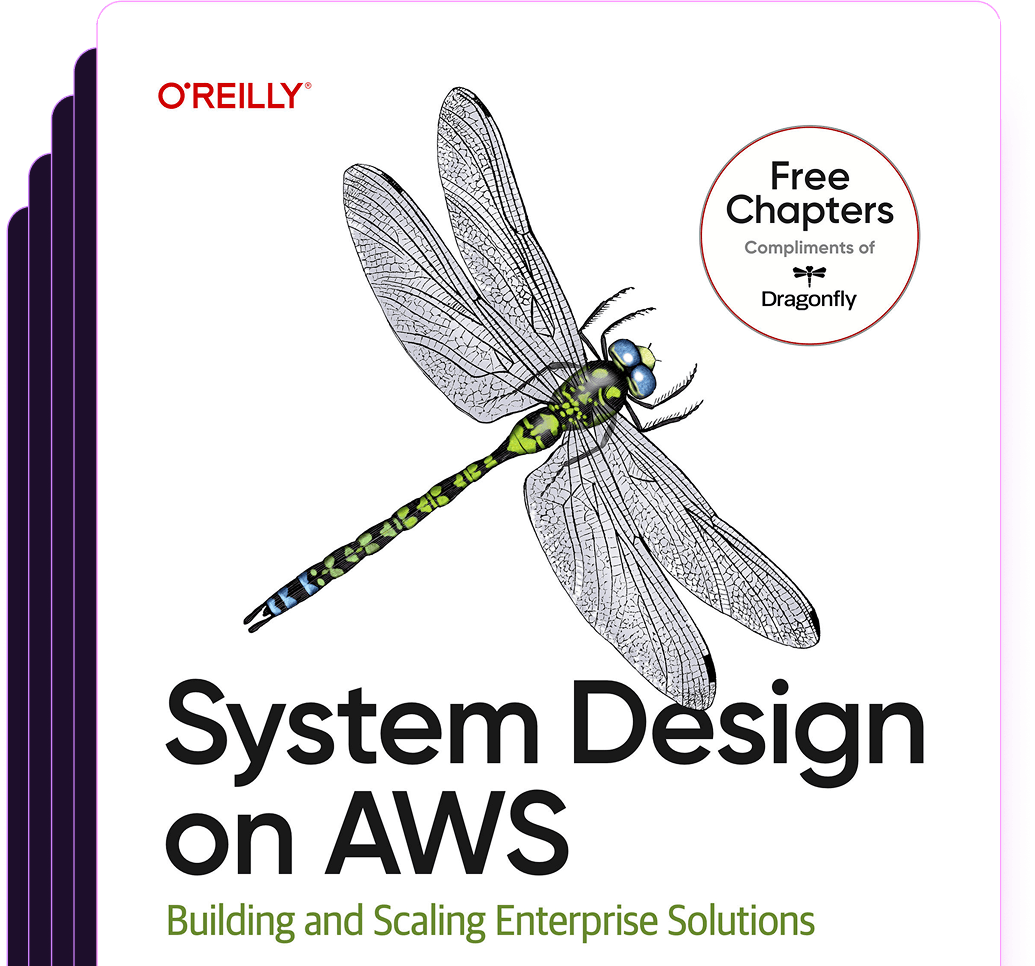
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost