Question: How do you make a character move in GameMaker?
Answer
In GameMaker, moving a character typically involves changing their x and y coordinates on the screen. Here's a step-by-step guide to making a basic character movement system:
Step 1: Create the Character Object
- In your GameMaker project, create a new object (e.g.,
obj_player
).
Step 2: Set Up Movement Variables
- In the Create Event of
obj_player
, initialize your movement variables. CODE_BLOCK_PLACEHOLDER_0
Step 3: Write the Movement Code
- To prevent permanent movement when keys are not pressed, ensure motion only occurs during key presses. Add this code in the Step Event of
obj_player
: CODE_BLOCK_PLACEHOLDER_1
Step 4: Add Sprites and Collision (Optional)
- Assign sprites to your
obj_player
to visually represent the character. - If necessary, add collision detection to prevent the character from moving through solid objects.
Step 5: Test Your Game
- Place
obj_player
in a room and run your game to test the movement.
This is a very basic example of character movement. Depending on your needs, you might want to implement acceleration, friction, or more complex mechanics like jumping or pathfinding. Also, consider using keyboard_check_pressed
for one-time key presses and keyboard_check_released
when you want an action to occur after releasing a key.
As your game development progresses, you may want to look into finite state machines (FSM) for more complex character behaviors and better code structure and organization.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Engines Questions (and Answers)
- Can You Use C# in Unreal Engine?
- Is Unreal Engine Open Source?
- Can Unreal Engine make 2D games?
- Does Unreal Engine Use C++?
- Does Unreal Engine Use Python?
- What Language Does Unreal Engine Use?
- Does Unreal Engine Use JavaScript?
- How Do I Uninstall Unreal Engine 5?
- Is Blender or Unreal Engine Better?
- Is Unreal Engine Good for Beginners?
- Does Unreal Engine Work on Mac?
- Why Is Unreal Engine So Laggy?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
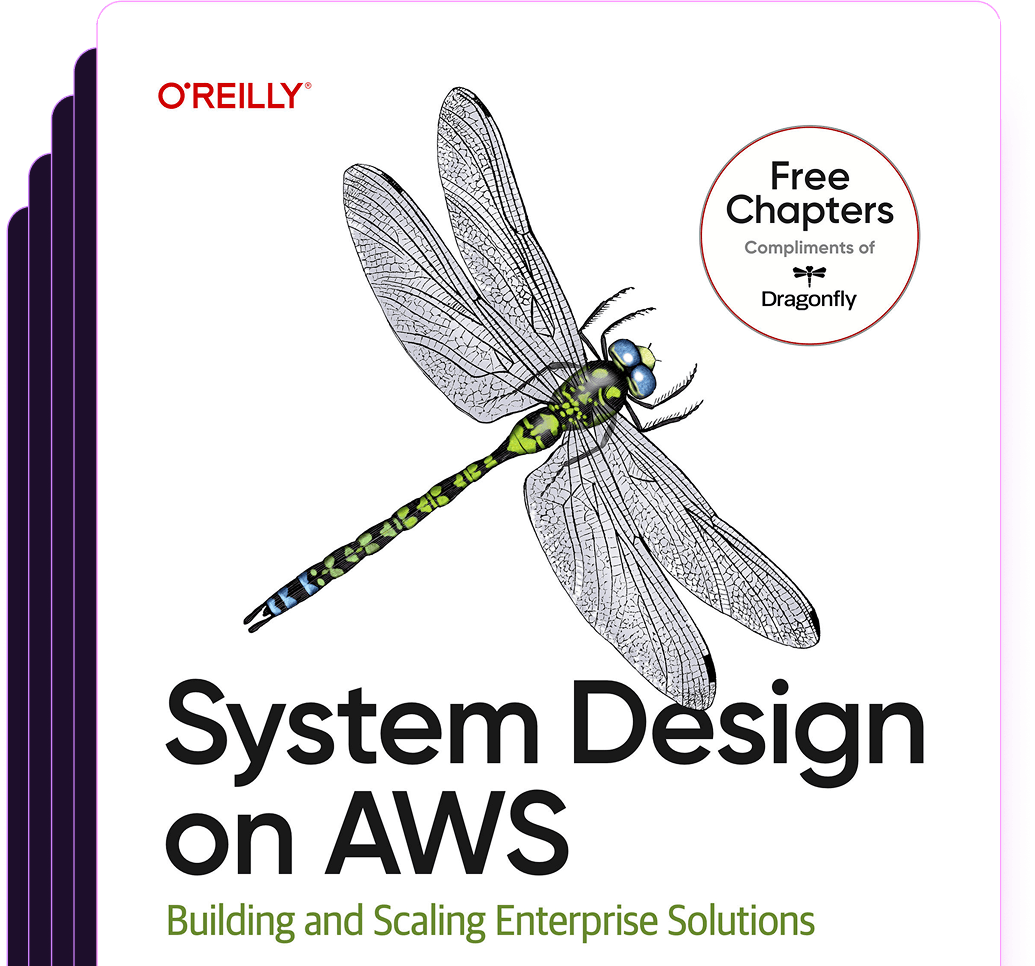
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost