Question: How do you export a GameMaker project to HTML5?
Answer
GameMaker Studio 2 offers the capability to export games as HTML5 projects. Here's a comprehensive guide to exporting your GameMaker project to HTML5:
- Setup HTML5 Module: Before exporting, make sure you have the HTML5 module installed in GameMaker Studio 2. This might require an additional purchase if it was not included in your original GameMaker license.
- Configure Your Project: Go to the
Game Options
and select theMain
tab under the HTML5 option. Here you will set various options such as game scaling, audio settings, and loader style. - Optimize Your Game: Since HTML5 games run in browsers, performance can vary across different devices. Optimize your game by minimizing the use of large sprites, excessive particles, and complex shaders which can affect performance on weaker hardware.
- Testing: Use the built-in runner to test your game within GameMaker. This can help catch platform-specific issues before you do a full export.
- Exporting the Game:
- Go to
File
>Export Project
. - Choose
HTML5
from the list of available platforms. - Select a location to save your project and configure any additional settings specific to your game.
- Go to
- Post Export: Once exported, you'll have a folder with an
index.html
file and associated assets. You can host this on any web server. For testing, you can run a local server or use GameMaker's built-in server feature. - Debugging: After hosting your game online, use browser tools for debugging. The developer tools in browsers like Chrome or Firefox are invaluable for diagnosing and fixing issues.
Here is an example of a simple GameMaker script that could be used when preparing to export your game, assuming you want to adjust a setting only for the HTML5 version:
if os_type == os_html5 {
// Adjust HTML5 specific settings here
display_set_gui_size(1024, 768);
}
And here's how you could modify your game_end
event specifically for HTML5 exports to ensure proper behavior:
if os_type == os_html5 {
// Insert code to run when ending the game in HTML5
window.location.href = "http://www.yourwebsite.com";
}
- Hosting: After exporting, you'll need to host your game on a server that supports HTML5 content. Games often require a MIME type setup for
.json
and some servers require additional configuration for WebSockets, etc.
Remember to test your game thoroughly on different browsers and systems since HTML5 games can behave differently depending on the user's environment.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Engines Questions (and Answers)
- Can You Use C# in Unreal Engine?
- Is Unreal Engine Open Source?
- Can Unreal Engine make 2D games?
- Does Unreal Engine Use C++?
- Does Unreal Engine Use Python?
- What Language Does Unreal Engine Use?
- Does Unreal Engine Use JavaScript?
- How Do I Uninstall Unreal Engine 5?
- Is Blender or Unreal Engine Better?
- Is Unreal Engine Good for Beginners?
- Does Unreal Engine Work on Mac?
- Why Is Unreal Engine So Laggy?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
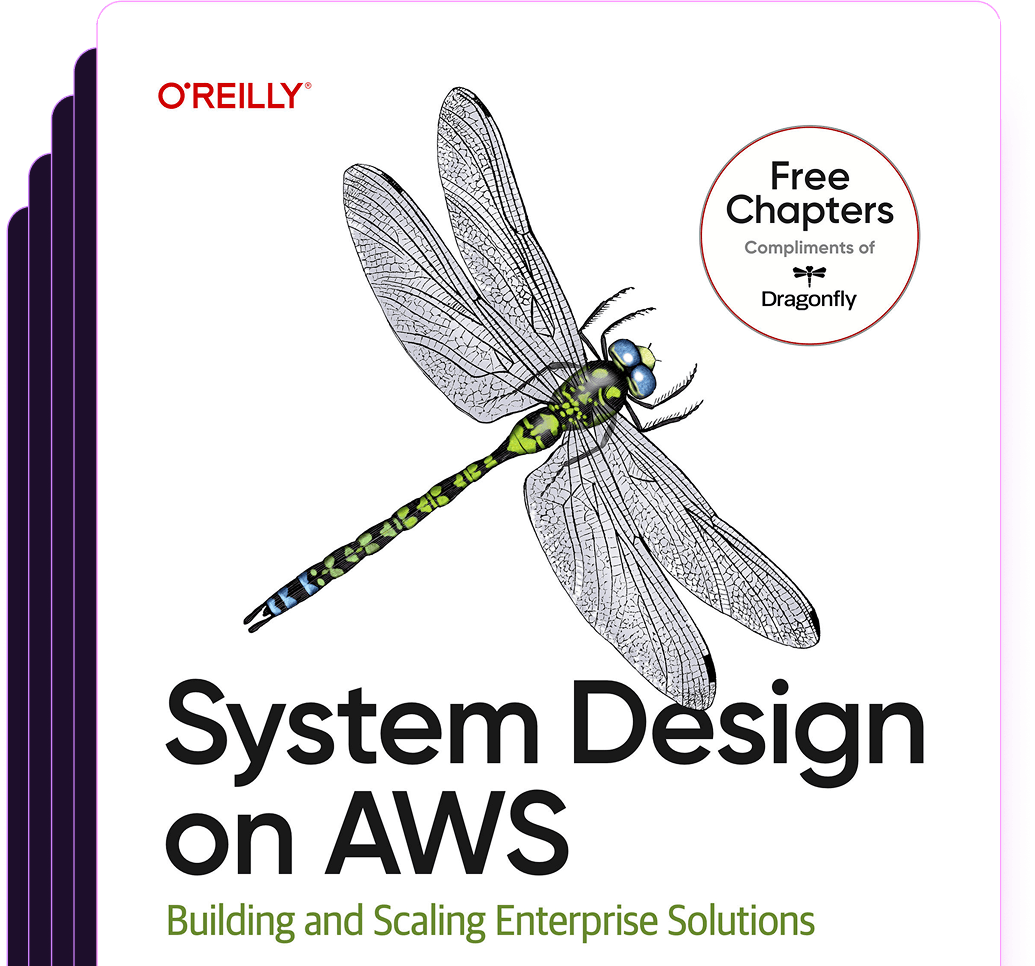
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost