Question: How do you implement tile collision in GameMaker Studio?
Answer
Tile collisions in GameMaker Studio are typically handled by checking for the presence of non-walkable tiles at the desired target location before actually moving an object. Here's how it can be accomplished:
Step 1: Define Walkable and Non-Walkable Tiles
In your tileset, you should have a clear distinction between walkable and non-walkable tiles. This is often represented as a boolean value or specific IDs that represent whether a character can move over a tile or not.
Step 2: Set Up Tile Layer and Collision Checking
When setting up your room with tiles, make sure to use layers correctly. You might have a separate layer for your collision tiles. When moving an object, before applying any movement, perform a collision check against this layer.
Step 3: Collision Code Example
Here's a basic example of how to perform a collision check based on the player's next position:
// Assuming you have variables for player speed and direction
var next_x = x + lengthdir_x(player_speed, player_direction);
var next_y = y + lengthdir_y(player_speed, player_direction);
// Calculate the grid position in the tilemap
var tilemap = layer_tilemap_get_id("Collision_Layer");
var tile_size = 32; // Change this to match your tile size
var grid_x = floor(next_x / tile_size);
var grid_y = floor(next_y / tile_size);
// Get the tile index at the calculated grid position
var tile_index = tilemap_get_at_pixel(tilemap, next_x, next_y);
// Check if the tile index corresponds to a solid tile
if (tile_index != -1 && tile_get_solid(tile_index))
{
// Collision detected! Handle accordingly.
}
else
{
// No collision, move the player
x = next_x;
y = next_y;
}
In this example, layer_tilemap_get_id
gets the ID of the tilemap layer named "Collision_Layer". The tilemap_get_at_pixel
function is used to check the tile index at the pixel position where the player intends to move. If tile_get_solid(tile_index)
returns true (assuming you have a function or a way to define which tiles are solid), it means there's a collision, and you should handle it by preventing movement or responding appropriately.
Step 4: Fine-Tuning
The above code assumes that your character aligns perfectly with the grid of your tilemap. If your game requires more complex movement (e.g., pixel-perfect collision or smaller step sizes), you'll need to adjust the collision code to check multiple points around your character's bounding box.
Step 5: Optimization
Checking every tile individually can be performance-heavy, especially with larger maps or many entities. As such, optimize by only checking tiles that your character is about to enter, and avoid unnecessary checks whenever possible.
Remember, you will need to tailor this solution to fit the particular needs and mechanics of your game, as collision systems can vary greatly depending on the type of game and movement mechanics you are implementing.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Engines Questions (and Answers)
- Can You Use C# in Unreal Engine?
- Is Unreal Engine Open Source?
- Can Unreal Engine make 2D games?
- Does Unreal Engine Use C++?
- Does Unreal Engine Use Python?
- What Language Does Unreal Engine Use?
- Does Unreal Engine Use JavaScript?
- Does Unreal Engine work on Linux?
- How Do I Uninstall Unreal Engine 5?
- Is Blender or Unreal Engine Better?
- Is Unreal Engine Good for Beginners?
- Does Unreal Engine Work on Mac?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
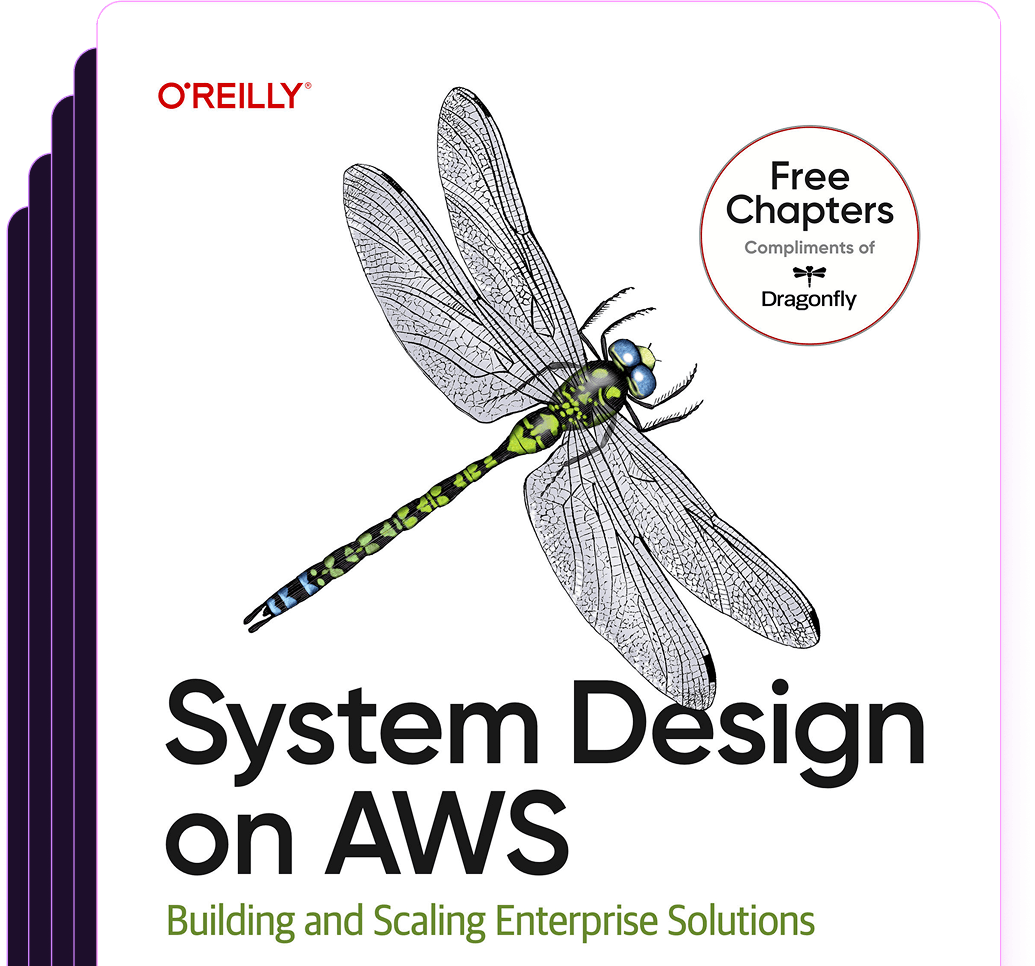
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost