Question: How do you implement WASD movement in GameMaker?
Answer
To implement WASD movement in GameMaker, you will need to use GameMaker Language (GML) to check for keyboard input and move an instance (such as the player character) accordingly. Below is a simple code example that you could put in the Step event of your player object to achieve basic WASD movement.
// Define movement speed
var move_speed = 4;
// Get player input
var move_x = keyboard_check(ord('D')) - keyboard_check(ord('A'));
var move_y = keyboard_check(ord('S')) - keyboard_check(ord('W'));
// Normalize diagonal movement
if (move_x != 0 && move_y != 0)
{
move_x *= 0.7071; // Approximately 1/sqrt(2)
move_y *= 0.7071; // Approximately 1/sqrt(2)
}
// Apply movement
x += move_x * move_speed;
y += move_y * move_speed;
This code checks if the keys 'W', 'A', 'S', 'D' are pressed and sets move_x
and move_y
accordingly. If both move_x
and move_y
are non-zero (i.e., the player is moving diagonally), their values are multiplied by approximately 0.7071
to ensure that the player's diagonal movement speed is consistent with their horizontal and vertical speeds.
The keyboard_check()
function returns true
if the specified key is being pressed and false
otherwise. The ord()
function converts a character to its ASCII value, which is necessary because keyboard_check()
expects a numerical key code.
Lastly, the x
and y
coordinates of the instance are updated by adding the product of the direction (move_x
or move_y
) and the move_speed
. This moves the instance in the intended direction according to the player's input.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Engines Questions (and Answers)
- Can You Use C# in Unreal Engine?
- Is Unreal Engine Open Source?
- Can Unreal Engine make 2D games?
- Does Unreal Engine Use C++?
- Does Unreal Engine Use Python?
- What Language Does Unreal Engine Use?
- Does Unreal Engine Use JavaScript?
- How Do I Uninstall Unreal Engine 5?
- Is Blender or Unreal Engine Better?
- Is Unreal Engine Good for Beginners?
- Does Unreal Engine Work on Mac?
- Why Is Unreal Engine So Laggy?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
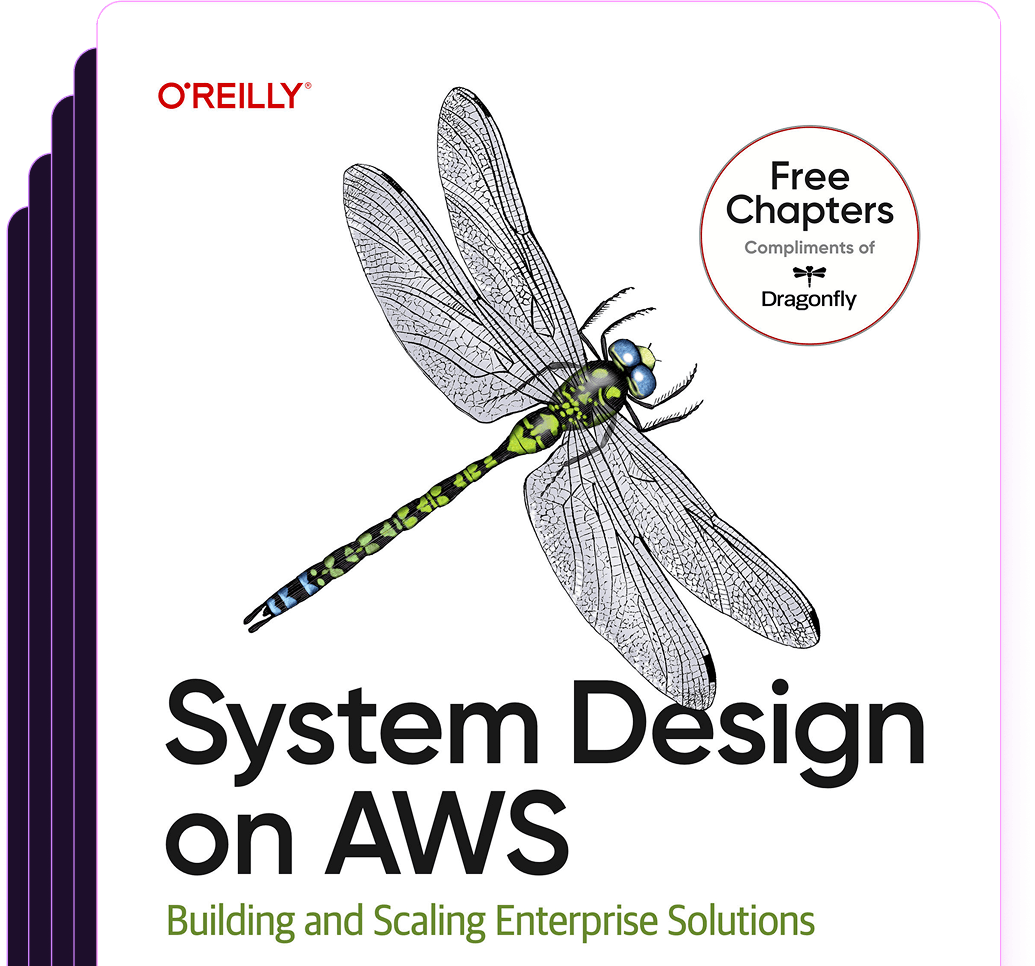
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost