Question: When should you use signals in Godot?
Answer
Signals in Godot are a way to implement the Observer pattern, which is useful for decoupling code and implementing a publish/subscribe notification system within your game.
Here are some scenarios where you might want to use signals:
- When an Event Occurs: If something happens in your game that other nodes or scripts may be interested in, like a player collecting a power-up or an enemy being defeated, you can emit a signal.
- For UI Elements: Buttons, sliders, and other UI elements emit signals to indicate interaction. For example, when a button is pressed, it emits a
pressed
signal. - Game State Changes: Signals can notify parts of your game when the state changes, such as transitioning from one level to another or when the game is paused.
- Asynchronous Operations: If you're performing a task that takes time, such as loading a resource or making a web request, you can emit a signal once the operation is complete.
- Custom Notifications: When you want to create a custom notification system, such as informing AI agents to change their behavior, use signals to broadcast this information.
Here's an example of how to define and emit a signal in a Godot script:
extends Node
# Define a signal called 'health_depleted'
signal health_depleted
func _ready():
# Connect the 'health_depleted' signal to a listener method on another node
connect("health_depleted", self, "_on_Health_depleted")
func take_damage(amount):
health -= amount
if health <= 0:
# Emit the 'health_depleted' signal when health runs out
emit_signal("health_depleted")
func _on_Health_depleted():
print("Health has been depleted!")
And here's how to connect to a signal from another script or node:
# Assuming 'player' is a node with the 'health_depleted' signal
player.connect("health_depleted", self, "_on_Player_health_depleted")
func _on_Player_health_depleted():
print("Player's health has been depleted!")
In summary, signals in Godot are essential for creating reactive, modular, and maintainable code, allowing different parts of your game to communicate effectively without tightly coupling them together.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Engines Questions (and Answers)
- Can You Use C# in Unreal Engine?
- Is Unreal Engine Open Source?
- Can Unreal Engine make 2D games?
- Does Unreal Engine Use C++?
- Does Unreal Engine Use Python?
- What Language Does Unreal Engine Use?
- Does Unreal Engine Use JavaScript?
- Does Unreal Engine work on Linux?
- How Do I Uninstall Unreal Engine 5?
- Is Blender or Unreal Engine Better?
- Is Unreal Engine Good for Beginners?
- Does Unreal Engine Work on Mac?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
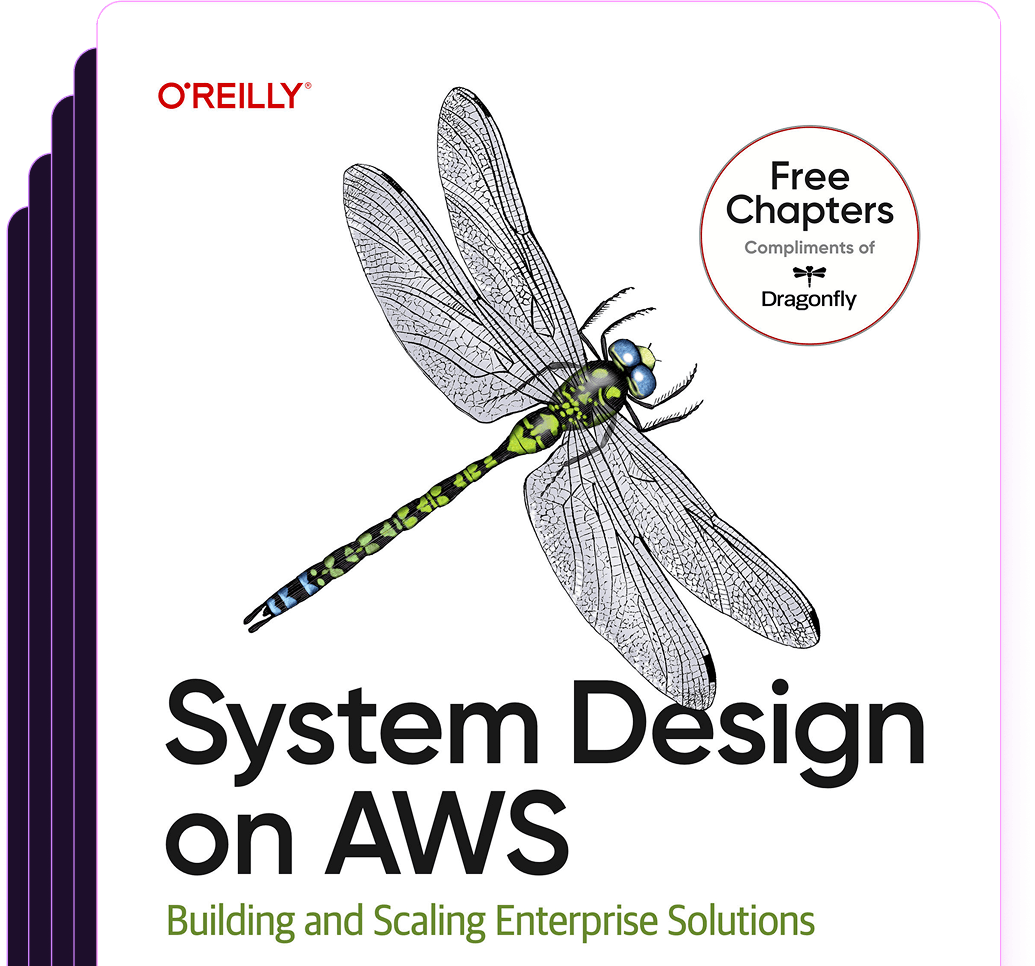
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost