Question: How do you handle input in Love2D?
Answer
Handling input in Love2D is straightforward and involves using the built-in keyboard and mouse functions provided by the Love2D API. Here are some common ways to handle input:
Keyboard Input: To detect if a specific key is pressed, use love.keyboard.isDown(key)
within the love.update(dt)
function.
function love.update(dt)
if love.keyboard.isDown("space") then
-- Spacebar was pressed, perform an action here
end
end
Key Presses and Releases: For single keypress events, use the love.keypressed(key)
and love.keyreleased(key)
callbacks.
function love.keypressed(key)
if key == "escape" then
love.event.quit()
end
end
Mouse Input: You can check the position and click status of the mouse with love.mouse.getPosition()
and love.mouse.isDown(button)
.
function love.update(dt)
if love.mouse.isDown(1) then -- 1 is the primary button (usually left-click)
local x, y = love.mouse.getPosition()
-- Process mouse click at position (x, y)
end
end
Mouse Click Events: The love.mousepressed(x, y, button, istouch, presses)
and love.mousereleased(x, y, button, istouch, presses)
callbacks can be used for handling mouse click events.
function love.mousepressed(x, y, button, istouch, presses)
if button == 1 then -- 1 is the primary button (usually left-click)
-- Mouse was pressed at position (x, y), handle it here
end
end
Joystick Input: Love2D also supports joystick input via love.joystick
module. Check if a button on the joystick is pressed with joystick:isDown(button)
.
function love.update(dt)
local joysticks = love.joystick.getJoysticks()
for i, joystick in ipairs(joysticks) do
if joystick:isDown(1) then
-- First button on the joystick is pressed
end
end
end
Text Input: For text input, enable text input mode with love.keyboard.setTextInput(true)
and use the love.textinput(text)
callback.
function love.load()
love.keyboard.setTextInput(true)
end
function love.textinput(text)
-- Append entered text to a string buffer or process it here
end
Using these functions and callbacks will allow you to react to user input in your Love2D game or application.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Engines Questions (and Answers)
- Can You Use C# in Unreal Engine?
- Is Unreal Engine Open Source?
- Can Unreal Engine make 2D games?
- Does Unreal Engine Use C++?
- Does Unreal Engine Use Python?
- What Language Does Unreal Engine Use?
- Does Unreal Engine Use JavaScript?
- Does Unreal Engine work on Linux?
- How Do I Uninstall Unreal Engine 5?
- Is Blender or Unreal Engine Better?
- Is Unreal Engine Good for Beginners?
- Does Unreal Engine Work on Mac?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
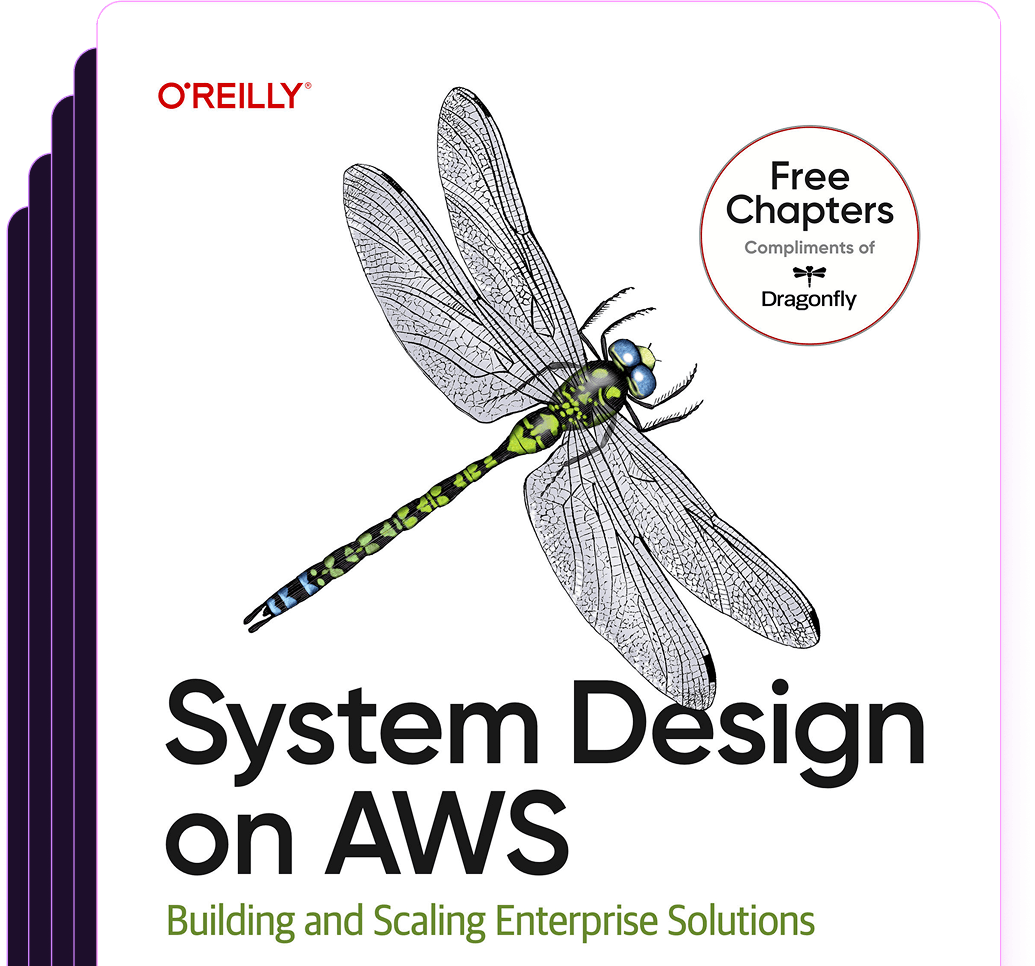
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost