Question: How do you display text in Love2D?
Answer
In Love2D, text can be displayed on the screen using the love.graphics.print
function or by creating a Font
object and then using it to print text. Here's how to use both methods:
Using love.graphics.print
This is the simpler method for drawing text directly on the screen. You specify the text, position, and optionally, rotation, scale, and other parameters.
function love.draw()
love.graphics.print("Hello, Love2D!", 400, 300)
end
Using Font
objects
For more control over the appearance of your text, you can create a Font object with a specific font and size, then use it to draw text.
function love.load()
-- Load a font file at size 14
myFont = love.graphics.newFont("font.ttf", 14)
end
function love.draw()
-- Set the active font
love.graphics.setFont(myFont)
-- Print text using the selected font
love.graphics.print("Hello, Love2D with custom font!", 400, 300)
end
To draw text with more advanced options like alignment and wrapping, you can use the love.graphics.printf
function:
function love.draw()
local text = "This text will be aligned and wrapped."
local x, y = 100, 100
local limit = 200 -- wrap the line after this many horizontal pixels
local align = "center" -- can also be 'left', 'right', or 'justify'
love.graphics.printf(text, x, y, limit, align)
end
When displaying text in Love2D, remember that the default font may not support all characters or look the way you want. In these cases, loading a custom font as shown above is the recommended approach.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Engines Questions (and Answers)
- Can You Use C# in Unreal Engine?
- Is Unreal Engine Open Source?
- Can Unreal Engine make 2D games?
- Does Unreal Engine Use C++?
- Does Unreal Engine Use Python?
- What Language Does Unreal Engine Use?
- Does Unreal Engine Use JavaScript?
- Does Unreal Engine work on Linux?
- How Do I Uninstall Unreal Engine 5?
- Is Blender or Unreal Engine Better?
- Is Unreal Engine Good for Beginners?
- Does Unreal Engine Work on Mac?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
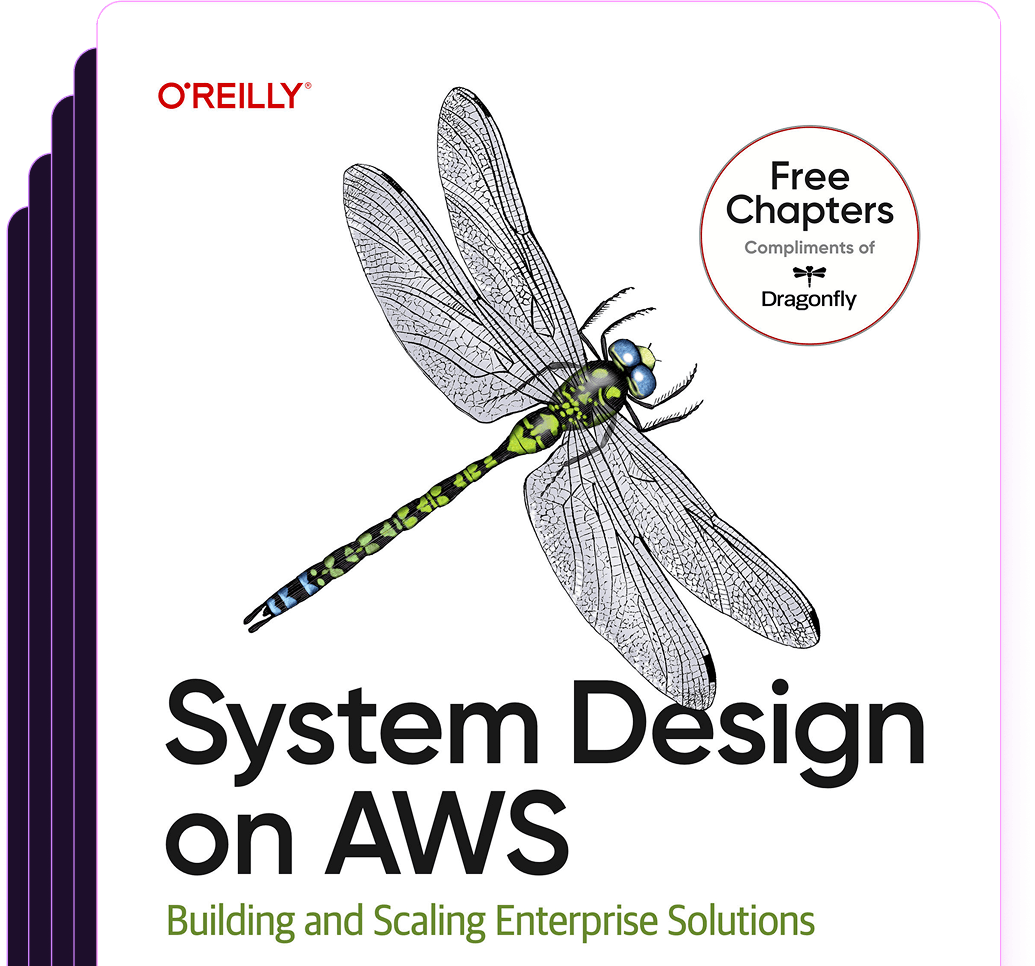
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost