Question: How can I debug in Love2D?
Answer
Debugging in Love2D, like in any programming language or framework, is an essential skill. Here are some strategies and tools you can use:
- Print Statements: The simplest form of debugging involves adding
print()
statements in your code to output the values of variables or to indicate that a certain line of code was reached.
```lua
function love.update(dt)
print("Update function is called")
-- Your update logic here
local x, y = player.getPosition()
print("Player position", x, y)
end
```
- Love2D Built-in Error Screen: Love2D has a built-in error screen that shows up when there's an unhandled error. It gives the stack trace of where the error occurred.
- ZeroBrane Studio: ZeroBrane Studio is an IDE that supports Lua and Love2D. It provides a more sophisticated way to debug Love2D projects with features such as breakpoints, step through code, watch variables, and evaluate expressions.
To use ZeroBrane Studio for debugging Love2D:
- Install and open ZeroBrane Studio.
- Go to
Project -> Lua Interpreter -> LÖVE
. - Set the path to your Love2D executable if it's not detected automatically.
- Open your project and place breakpoints.
- Start debugging by clicking
Project -> Start Debugging
.
- External Libraries: There are libraries available which can be integrated into your Love2D game to help with debugging. An example is
love-debug
which provides an on-screen console where you can enter commands, inspect variables, and even run Lua code on the fly.
```lua
local debug = require('debugger')
function love.load()
-- Your load logic here
end
function love.update(dt)
debug.update(dt) -- Update debug console
-- Your update logic here
end
function love.draw()
debug.draw() -- Draw debug console
-- Your draw logic here
end
function love.textinput(t)
debug.textinput(t) -- Pass text input to debug console
end
function love.keypressed(key)
debug.keypressed(key) -- Pass key presses to debug console
end
```
- Mobile Debugger (for mobile development): If you're developing a Love2D game for mobile devices, you might use a mobile debugger tool like MobDebug which is included with ZeroBrane Studio.
Using these methods, you can track down errors, inspect variables at runtime, and understand the flow of your program better. Combining various techniques will provide a more robust debugging approach for your Love2D projects.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Engines Questions (and Answers)
- Can You Use C# in Unreal Engine?
- Is Unreal Engine Open Source?
- Can Unreal Engine make 2D games?
- Does Unreal Engine Use C++?
- Does Unreal Engine Use Python?
- What Language Does Unreal Engine Use?
- Does Unreal Engine Use JavaScript?
- How Do I Uninstall Unreal Engine 5?
- Is Blender or Unreal Engine Better?
- Is Unreal Engine Good for Beginners?
- Does Unreal Engine Work on Mac?
- Why Is Unreal Engine So Laggy?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
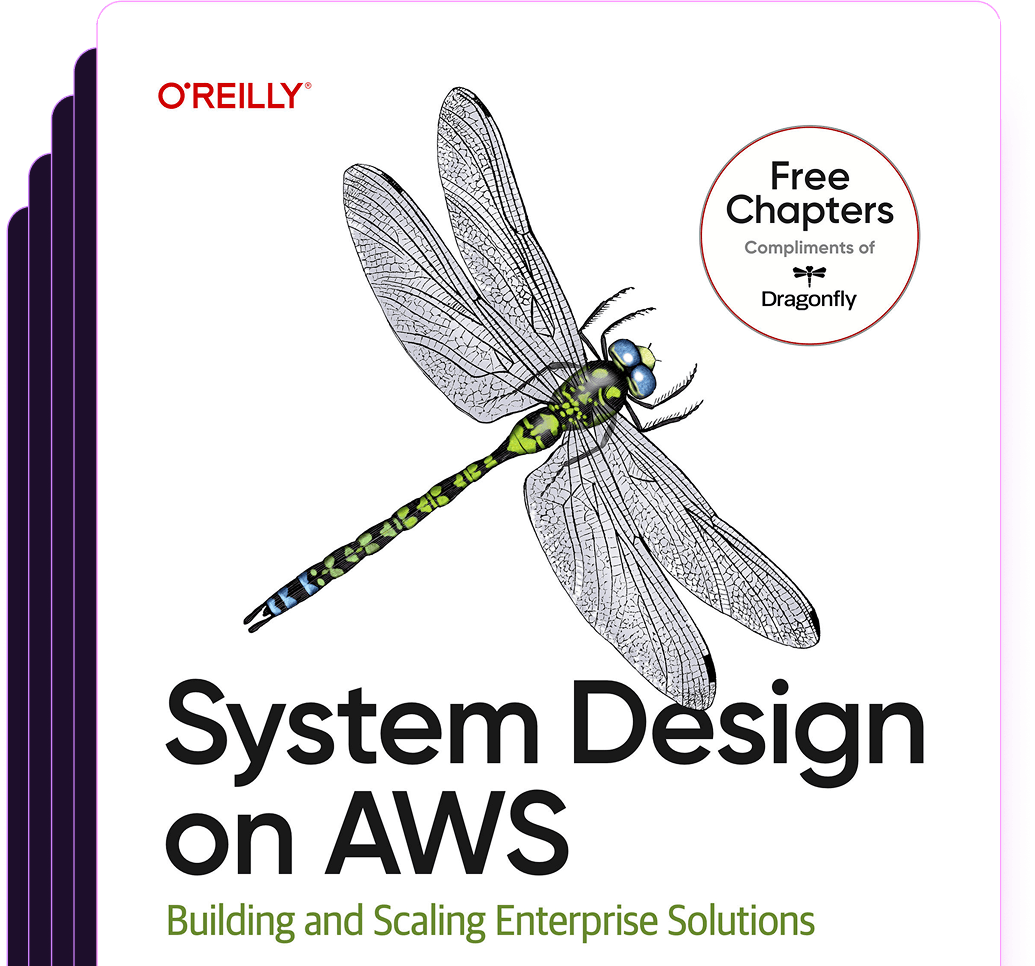
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost