Question: Is Love2D a good framework for game development?
Answer
Love2D, also known as LÖVE, is an open-source framework that is designed specifically for 2D game development. It utilizes the Lua programming language and is appreciated for its simplicity and ease of use. Here are some points that highlight the strengths and limitations of Love2D:
Benefits of Using Love2D:
- Simplicity: Love2D has a simple and easy-to-understand API, making it accessible for beginners.
- Lua Language: Lua is a very lightweight and fast scripting language which is great for games.
- Community: There is a supportive community around Love2D that contributes to its documentation, shares libraries, and helps with problem-solving.
- Cross-Platform: Games made with Love2D can be run on Windows, macOS, Linux, Android, and iOS with minimal platform-specific code.
Code Example:
Here's a quick example of how you might set up a window in Love2D:
function love.load()
love.window.setMode(800, 600, { resizable=false, vsync=true })
end
function love.draw()
love.graphics.print("Hello World", 400, 300)
end
Limitations of Love2D:
- 2D Focused: As the name implies, it's primarily designed for 2D games; 3D game development is possible but not as straightforward.
- Performance: While Lua is fast for a scripting language, performance-intensive tasks may not run as well as they would in lower-level languages like C++.
- Learning Curve: For those unfamiliar with Lua, there is a learning curve associated with the language.
Conclusion:
Love2D is a good choice for developers who are interested in creating 2D games and who want a framework that allows them to get started quickly. Its balance of simplicity, flexibility, and performance make it suitable for hobbyists, indie game developers, and educational purposes. However, for large-scale or 3D projects, other engines like Unity or Unreal might be more appropriate choices.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Engines Questions (and Answers)
- Can You Use C# in Unreal Engine?
- Is Unreal Engine Open Source?
- Can Unreal Engine make 2D games?
- Does Unreal Engine Use C++?
- Does Unreal Engine Use Python?
- What Language Does Unreal Engine Use?
- Does Unreal Engine Use JavaScript?
- How Do I Uninstall Unreal Engine 5?
- Is Blender or Unreal Engine Better?
- Is Unreal Engine Good for Beginners?
- Does Unreal Engine Work on Mac?
- Why Is Unreal Engine So Laggy?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
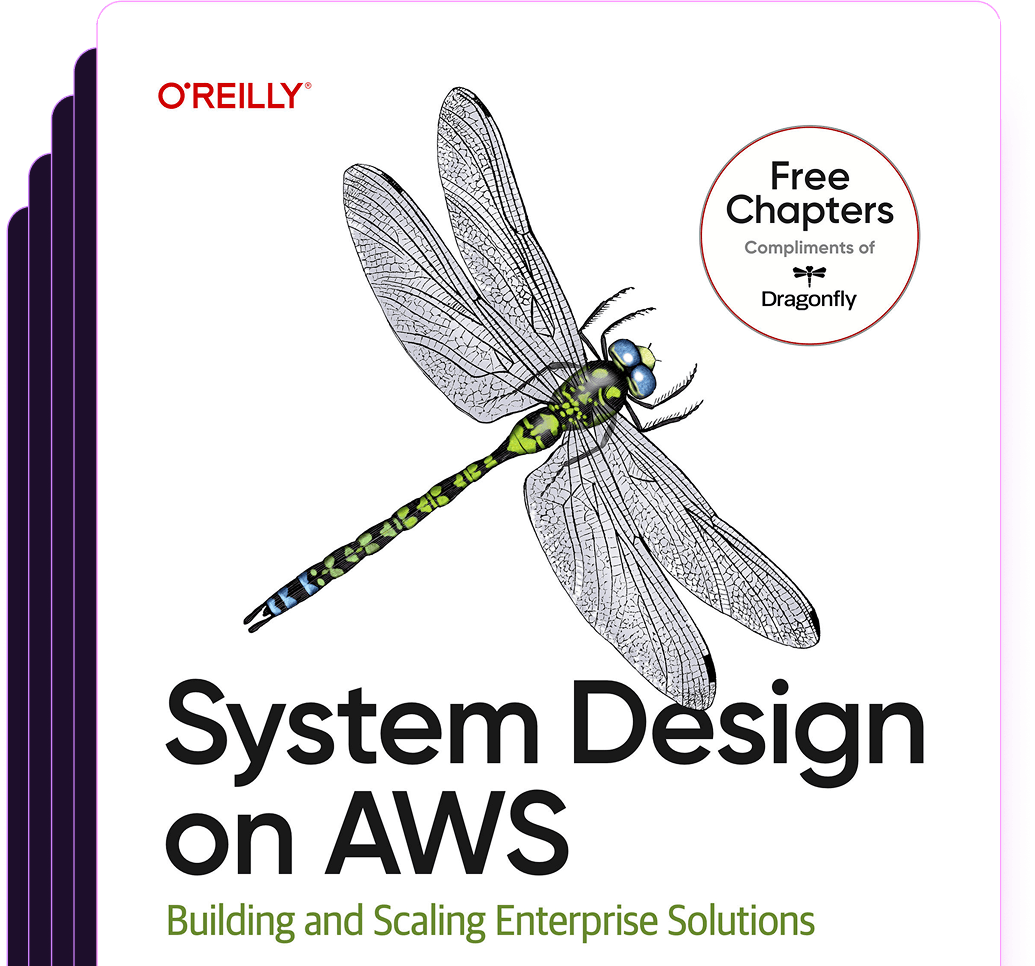
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost