Question: How do I use the print function in Love2D?
Answer
In Love2D, the print
function is used for printing text to the console, which is helpful for debugging purposes. However, if you want to display text within the window of your game or application, you will need to use the graphics library provided by Love2D, specifically the love.graphics.print
function.
Here's an example of how you can draw text on the screen:
function love.draw()
love.graphics.setFont(love.graphics.newFont(14)) -- Sets the default font with size 14
love.graphics.setColor(1, 1, 1) -- Sets the color to white
love.graphics.print("Hello, World!", 400, 300)
end
In this example:
love.graphics.setFont
sets the current font. If you don't set a font, Love2D uses the default font with a default size.love.graphics.setColor
is setting the color of the text. The values are in the range [0, 1] for Red, Green, Blue, and optionally Alpha (transparency).love.graphics.print
takes the string to print and the x and y coordinates where it should be drawn on the screen.
The love.graphics.print
function has more advanced uses where you can specify additional parameters such as the angle of rotation, scale factors, and more. Here's an example demonstrating these optional parameters:
function love.draw()
local x, y = 400, 300
local text = "Rotated Text"
local angle = math.pi / 4 -- 45 degrees
love.graphics.print(text, x, y, angle)
end
In this extended example, the text "Rotated Text"
is drawn at a 45-degree angle. The x
and y
variables are still controlling the position, but now we also pass angle
to rotate the text.
For even more control over the appearance of text, you can create a Text
object using love.graphics.newText
, which allows for efficient rendering of static text.
function love.load()
local font = love.graphics.newFont(14)
myText = love.graphics.newText(font, "Hello, World!")
end
function love.draw()
love.graphics.draw(myText, 400, 300)
end
This technique is especially useful when dealing with text that doesn't change frequently, as it avoids the overhead of generating the text image each frame.
These are some of the basic ways to use the print functionality in Love2D to draw text onto the game window.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Engines Questions (and Answers)
- Can You Use C# in Unreal Engine?
- Is Unreal Engine Open Source?
- Can Unreal Engine make 2D games?
- Does Unreal Engine Use C++?
- Does Unreal Engine Use Python?
- What Language Does Unreal Engine Use?
- Does Unreal Engine Use JavaScript?
- Does Unreal Engine work on Linux?
- How Do I Uninstall Unreal Engine 5?
- Is Blender or Unreal Engine Better?
- Is Unreal Engine Good for Beginners?
- Does Unreal Engine Work on Mac?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
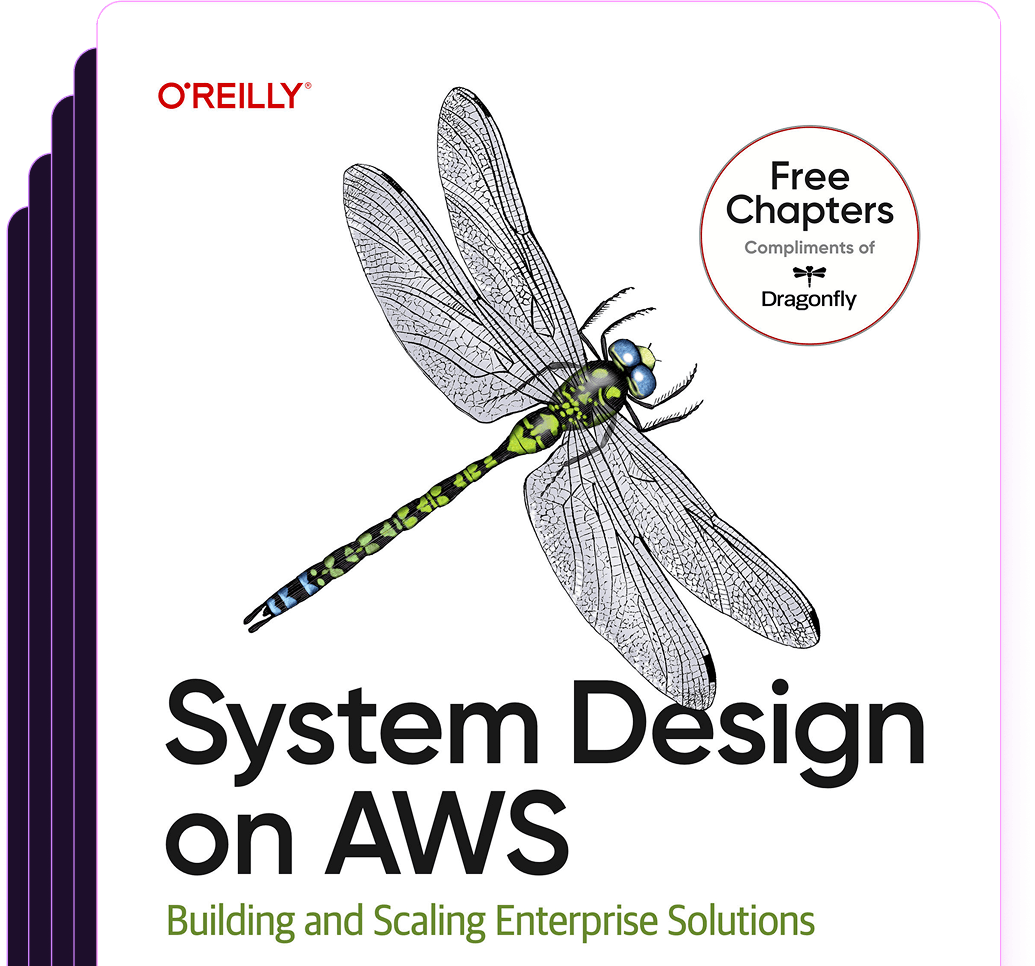
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost