Question: How do I install Love2D on my system?
Answer
Installing LÖVE (Love2D) is straightforward and depends on the operating system you are using. Below are instructions for Windows, macOS, and Linux:
Windows
- Go to the LÖVE website.
- Click on the download link for Windows.
- Choose either the 32-bit or 64-bit .exe file according to your system's architecture.
- Run the downloaded installer and follow the on-screen instructions to complete the installation.
macOS
- Visit the LÖVE website.
- Click on the download link for macOS.
- Drag the LÖVE application into your Applications folder.
You can also install LÖVE using Homebrew:
brew install --cask love
Linux
The installation method varies by distribution. Here are common methods for Ubuntu and Arch Linux:
Ubuntu
For Ubuntu, you can install LÖVE from the official PPA:
sudo add-apt-repository ppa:bartbes/love-stable
sudo apt-get update
sudo apt-get install love
Arch Linux
Arch Linux users can find LÖVE in the Community repository:
sudo pacman -S love
For other distributions, you might need to compile from source or find a suitable package. Refer to your distribution's package management tools and documentation.
Testing the Installation
After installing, you can test if LÖVE is working correctly by typing love
in your terminal or command prompt. If installed properly, the LÖVE window should pop up displaying the no-game screen, which is a LÖVE logo with version information.
Running Your First Love2D Game
To run a LÖVE game, you can drag and drop the game folder (which must contain a main.lua
file) onto the LÖVE application icon or use the terminal like so:
love /path/to/game/directory
This will open the game in the LÖVE window.
```markdown
Remember that the procedures may change with new versions of LÖVE, always check the official documentation for the most up-to-date instructions.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Engines Questions (and Answers)
- What Language Does Unreal Engine Use?
- Why is Unreal Engine so good?
- Is Unreal Engine Free?
- What is Godot written in?
- What are the differences between Unitys URP and HDRP?
- Why should developers use the Universal Render Pipeline (URP) in Unity?
- Is Unity URP good for mobile?
- Does GameMaker use C++?
- How do you export a game in GameMaker?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
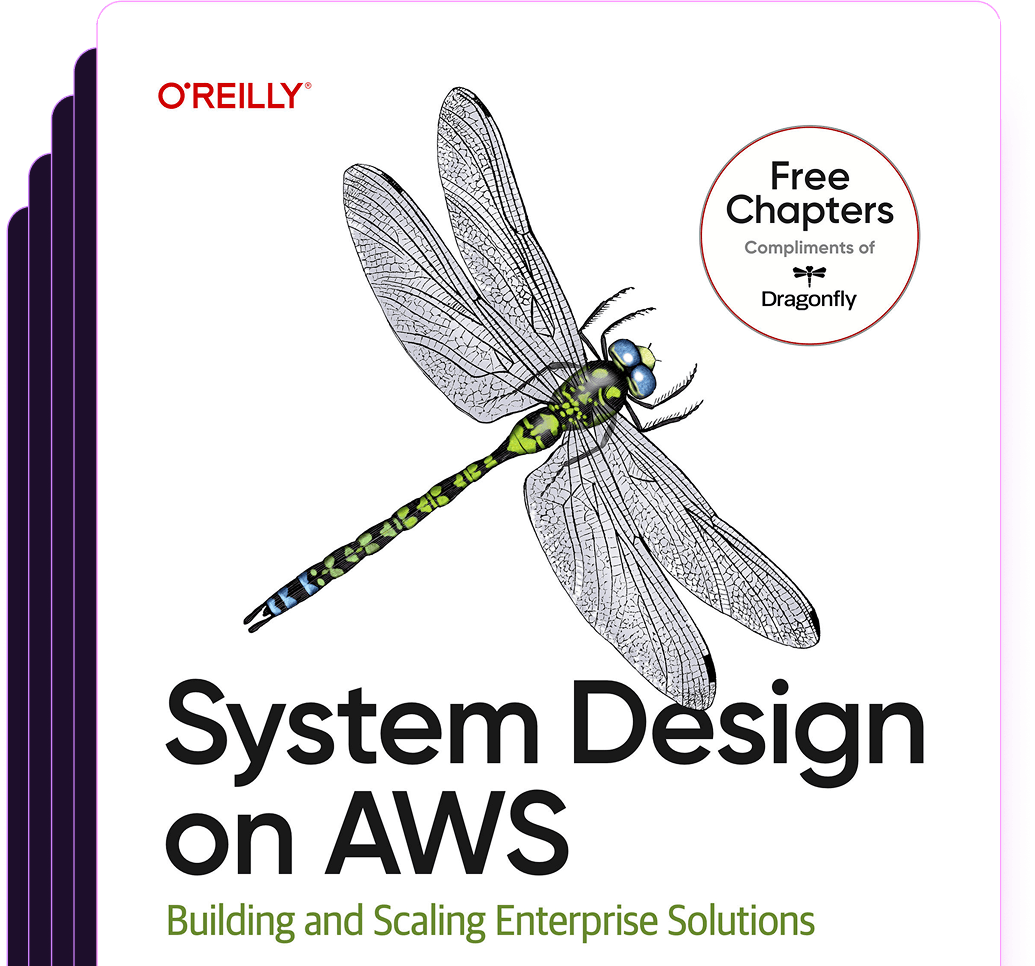
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost