Question: What Language Does Unreal Engine Use?
Answer
Unreal Engine (UE) is a versatile game development platform known for its powerful graphics and flexible programming options. Developers can use multiple programming languages to create games and interactive content in UE, each offering different strengths depending on the project requirements.
C++
Overview
C++ is the primary programming language used in Unreal Engine for game development. It offers robust control over hardware and graphical processes, making it ideal for high-performance games.
Code Example
// Basic C++ Actor class in Unreal Engine
#include "GameFramework/Actor.h"
#include "CoreMinimal.h"
class AMyActor : public AActor
{
GENERATED_BODY()
public:
// Sets default values for this actor's properties
AMyActor();
// Called every frame
virtual void Tick(float DeltaTime) override;
protected:
// Called when the game starts or when spawned
virtual void BeginPlay() override;
private:
float RunningTime;
};
Blueprints Visual Scripting
Overview
Blueprints is Unreal Engine's visual scripting system that allows developers to create gameplay elements without prior programming knowledge. It's user-friendly and integrates seamlessly with C++.
Visual Example
Imagine a node-based interface where you drag and connect different actions and events to create complex game logic.
Python
Overview
Python is used in Unreal Engine primarily for scripting repetitive tasks and automations within the editor, rather than for gameplay logic.
Code Example
# Example of Python script for Unreal Engine to automate a task
import unreal
# Load an asset by its path
asset_path = '/Game/Textures/MyTexture'
texture = unreal.load_asset(asset_path)
# Print the texture's type
print(texture.get_class().get_name())
Conclusion
Unreal Engine supports multiple languages, primarily C++ for core game development and Blueprints for visual scripting. Python is also available for editor automation. This diversity allows developers of different skill levels and preferences to work effectively in Unreal Engine.
For more detailed examples and tutorials, visit the official Unreal Engine documentation or community forums.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Engines Questions (and Answers)
- Can You Use C# in Unreal Engine?
- Is Unreal Engine Open Source?
- Can Unreal Engine make 2D games?
- Does Unreal Engine Use C++?
- Does Unreal Engine Use Python?
- Does Unreal Engine Use JavaScript?
- How Do I Uninstall Unreal Engine 5?
- Is Blender or Unreal Engine Better?
- Is Unreal Engine Good for Beginners?
- Does Unreal Engine Work on Mac?
- Why Is Unreal Engine So Laggy?
- Can Unreal Engine Make Mobile Games?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
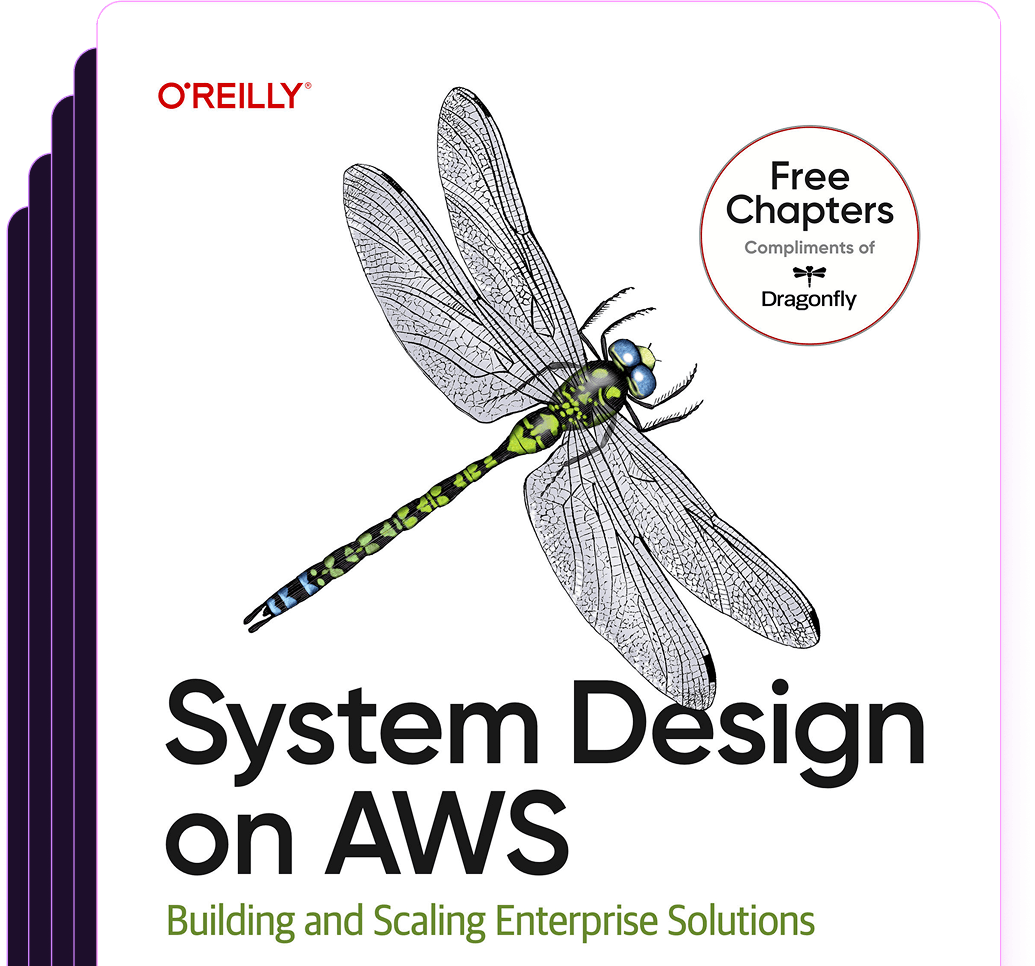
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost