Getting JSON Values from Redis in Python (Detailed Guide w/ Code Examples)
Use Case(s)
Retrieving JSON data from Redis is a common operation when using Python and Redis together. This operation comes handy in scenarios such as caching complex data structures, session management, or working with task queues involving structured data.
Code Examples
Example 1: Using json.loads
to deserialize JSON data
In this example, we'll use Redis' GET command to retrieve a JSON serialized value. We'll then deserialize it using Python's json library.
import redis
import json
client = redis.Redis(host='localhost', port=6379, db=0)
value = client.get('key')
# Ensure value exists and then deserialize
if value:
data = json.loads(value.decode('utf-8'))
In this code, after retrieving the value from Redis, we decode the byte-string to a normal string before deserializing it into a Python object.
Best Practices
- It's a good practice to handle the case where a key might not exist in Redis. Using an
if value:
check before trying to deserialize can prevent errors. - Whenever possible, try to keep your JSON objects small. Large JSON objects can lead to increased memory usage and slow down your application.
Common Mistakes
- One common mistake is forgetting to decode the byte-string to a normal string before attempting to deserialize it. Python's json library expects a string, not bytes.
FAQs
Q: Can I store complex Python objects as JSON in Redis?
A: Yes, you can serialize most Python objects to JSON before storing them in Redis. However, some types of Python objects, like custom classes, cannot be directly serialized to JSON.
Q: What if the key does not exist in Redis?
A: If the key does not exist, Redis will return None
. It's important to handle this case in your application code.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Getting All Keys Matching a Pattern in Redis using Python
- Getting the Length of a Redis List in Python
- Getting Server Info from Redis in Python
- Getting Number of Connections in Redis Using Python
- Getting Current Redis Version in Python
- Getting Memory Stats in Redis using Python
- Redis Get All Databases in Python
- Redis Get All Keys and Values in Python
- Retrieving a Key by Value in Redis Using Python
- Python Redis: Get Config Settings
- Getting All Hash Keys Using Redis in Python
- Get Total Commands Processed in Redis Using Python
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
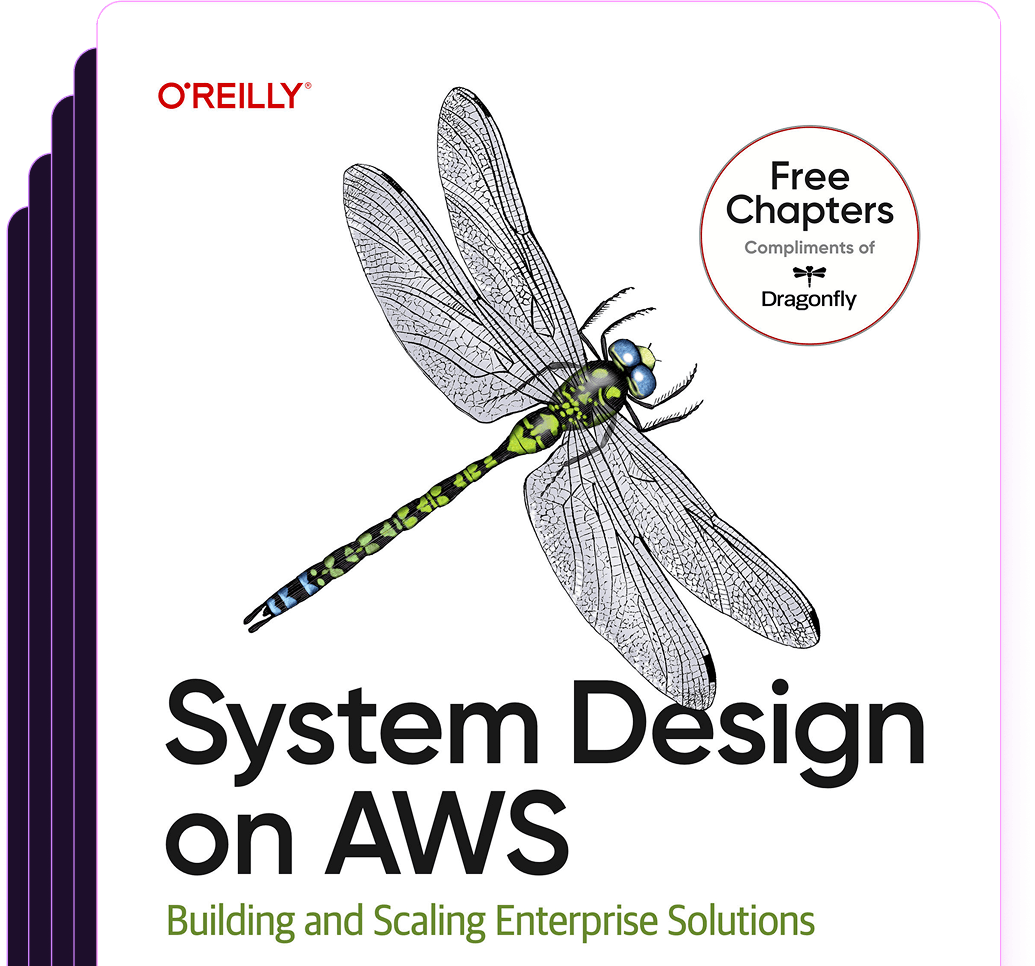
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost