Deleting a Redis Hash in Golang (Detailed Guide w/ Code Examples)
Use Case(s)
Often, you might need to delete specific hashes from Redis, like when data becomes obsolete or for regular cleanup operations. In Golang, you can use the DEL
command to remove these hashes.
Code Examples
Let's look at code examples using the go-redis/redis
package.
To delete a hash: CODE_BLOCK_PLACEHOLDER_0
This example shows how to connect to a Redis server and delete a hash using the Del
method. Replace 'your_hash_key' with the key of your hash.
Best Practices
- It's recommended to handle errors returned by the
Del
function to ensure that the operation was successful. - Always remember to close the connection to the Redis server after performing your operations. You can do this using the
Close
function.
Common Mistakes
- Not handling the error object returned from the
Del
function may lead to silent failures where the hash is not deleted, but the program continues running. - Not closing the Redis connection after finishing your operations can lead to resource leaks.
FAQs
Q: Can I delete multiple hashes at once? A: Yes, you can pass multiple keys to the Del
command like so: rdb.Del(ctx, "key1", "key2", "key3")
. Each key will be deleted from Redis.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Deleting a Redis Key with GoLang
- Deleting All Keys in Redis Using Golang
- Deleting a Sorted Set in Redis Using Golang
- Deleting a Set in Redis using Golang
- Deleting Redis Keys by Pattern in Golang
- Golang: Deleting Redis Keys by Prefix
- Deleting a Redis Key After Time Using Golang
- Deleting Redis Cache in Golang
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
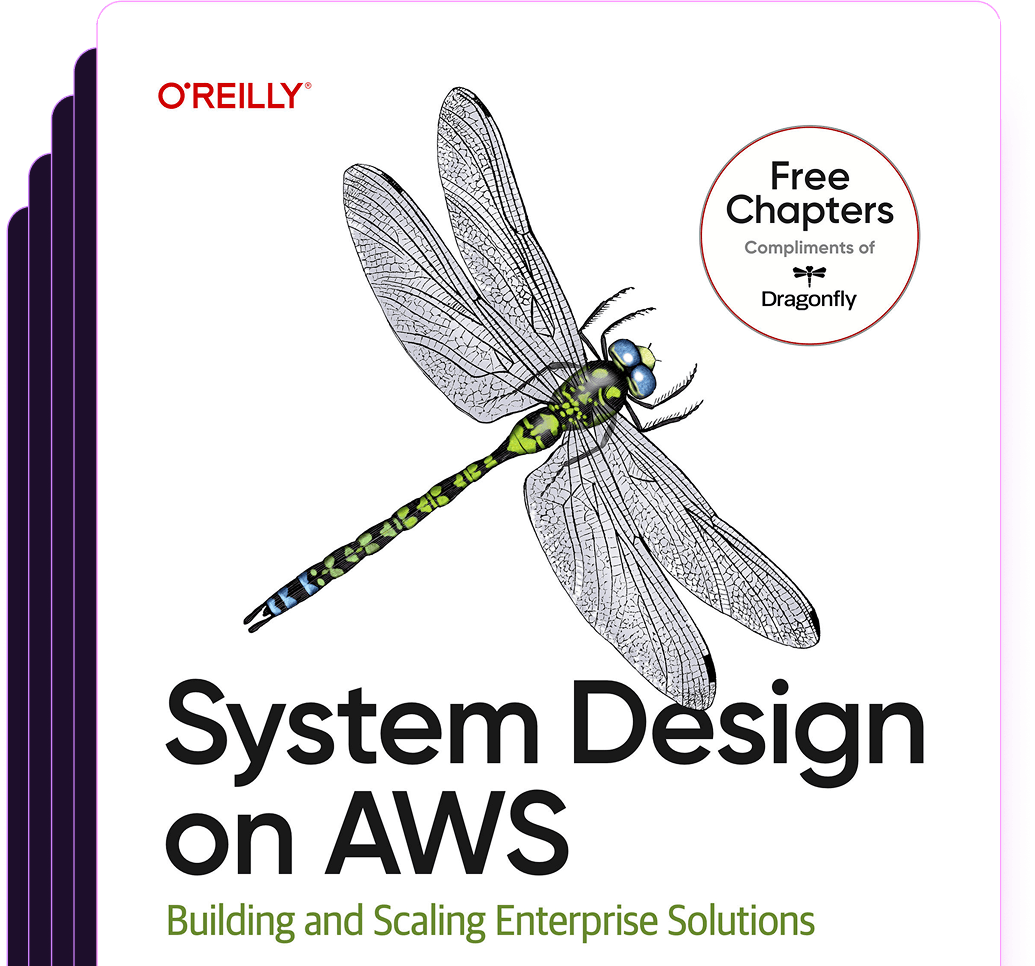
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost