Golang: Deleting Redis Keys by Prefix (Detailed Guide w/ Code Examples)
Use Case(s)
In Golang applications that utilize Redis as a key-value store, it might be necessary to delete keys based on a certain prefix. This can be used for data cleanup processes or when related keys need to be removed at once.
Code Examples
The following example shows how to use the Scan
function with a specified prefix and then remove the matching keys. Please install the go-redis/redis
package before running this code.
package main
import (
"github.com/go-redis/redis/v8"
"context"
"fmt"
)
func main() {
rdb := redis.NewClient(&redis.Options{
Addr: "localhost:6379",
Password: "", // no password set
DB: 0, // use default DB
})
ctx := context.Background()
var cursor uint64
var n int
for {
var keys []string
var err error
keys, cursor, err = rdb.Scan(ctx, cursor, "myprefix*", 10).Result()
if err != nil {
panic(err)
}
for _, key := range keys {
fmt.Println("deleting key: ", key)
rdb.Del(ctx, key)
}
if cursor == 0 {
break
}
}
fmt.Printf("Deleted %d keys\n", n)
}
This code connects to a local Redis instance, then it scans keys that match the prefix myprefix
. Matching keys are then deleted using Del
function.
Best Practices
- Make sure to limit the number of keys per Scan operation to avoid blocking the server for long periods.
- Always handle the errors returned by the
Scan
andDel
functions.
Common Mistakes
- A common mistake is to use the
KEYS
command followed byDEL
in production environments.KEYS
can block the server when used against large databases, preferSCAN
instead.
FAQs
Q: Can I delete keys with multiple different prefixes at once? A: No, you need to run separate Scan
and Del
operations for each prefix.
Q: What happens if there are no keys matching the prefix? A: The Scan
function will simply return an empty list, and no keys will be deleted.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
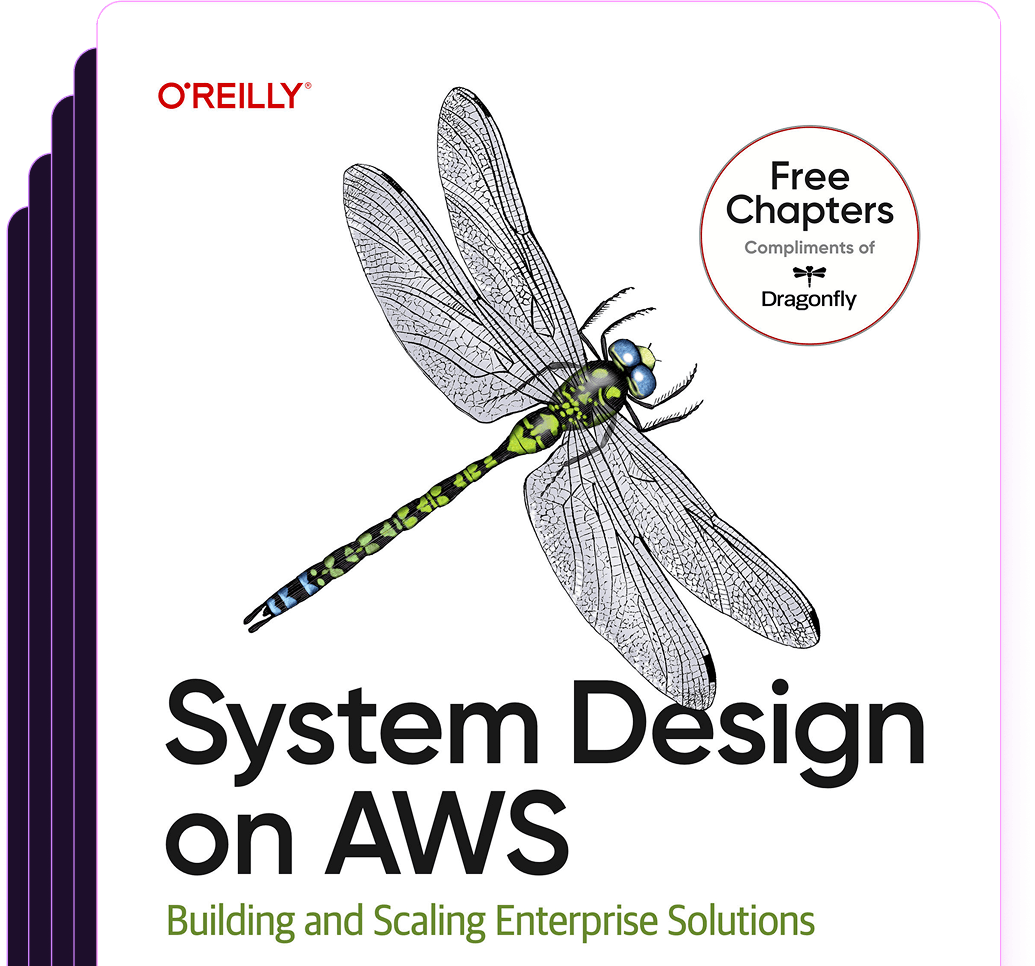
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost