Deleting a Redis Key with GoLang (Detailed Guide w/ Code Examples)
Use Case(s)
Deleting a key from Redis is a common operation that you might need to perform when you want to remove a certain data point from your cache. This can be handy in situations where the data associated with a key has become obsolete or irrelevant and needs to be cleaned up.
Code Examples
To delete a key in Redis using Go, you can use the Del
function provided by the go-redis
package. Here's an example:
package main
import (
"github.com/go-redis/redis/v8"
"context"
)
func main() {
rdb := redis.NewClient(&redis.Options{
Addr: "localhost:6379",
Password: "",
DB: 0,
})
ctx := context.Background()
res, err := rdb.Del(ctx, "key1").Result()
if err != nil {
panic(err)
}
println("Number of keys deleted:", res)
}
In this example, we're deleting the key named key1
. The Del
function returns the number of keys that were deleted.
Best Practices
When deleting keys in Redis with either Go or any other language, aim to delete keys only when necessary as this could lead to data loss if not handled properly. Always ensure you have a solid reason for deleting a key and ensure the key you are deleting is the correct one.
Common Mistakes
A common mistake when deleting keys in Redis with Go is not properly handling errors. When using the Del
function, always check the error that is returned. If an error occurs during deletion, it should be properly logged and handled.
FAQs
Q: Can I delete multiple keys at once in Redis with Go? A: Yes, you can pass multiple keys to the Del
function like so: rdb.Del(ctx, "key1", "key2", "key3")
. This will attempt to delete all the provided keys.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Deleting All Keys in Redis Using Golang
- Deleting a Redis Hash in Golang
- Deleting a Sorted Set in Redis Using Golang
- Deleting a Set in Redis using Golang
- Deleting Redis Keys by Pattern in Golang
- Golang: Deleting Redis Keys by Prefix
- Deleting a Redis Key After Time Using Golang
- Deleting Redis Cache in Golang
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
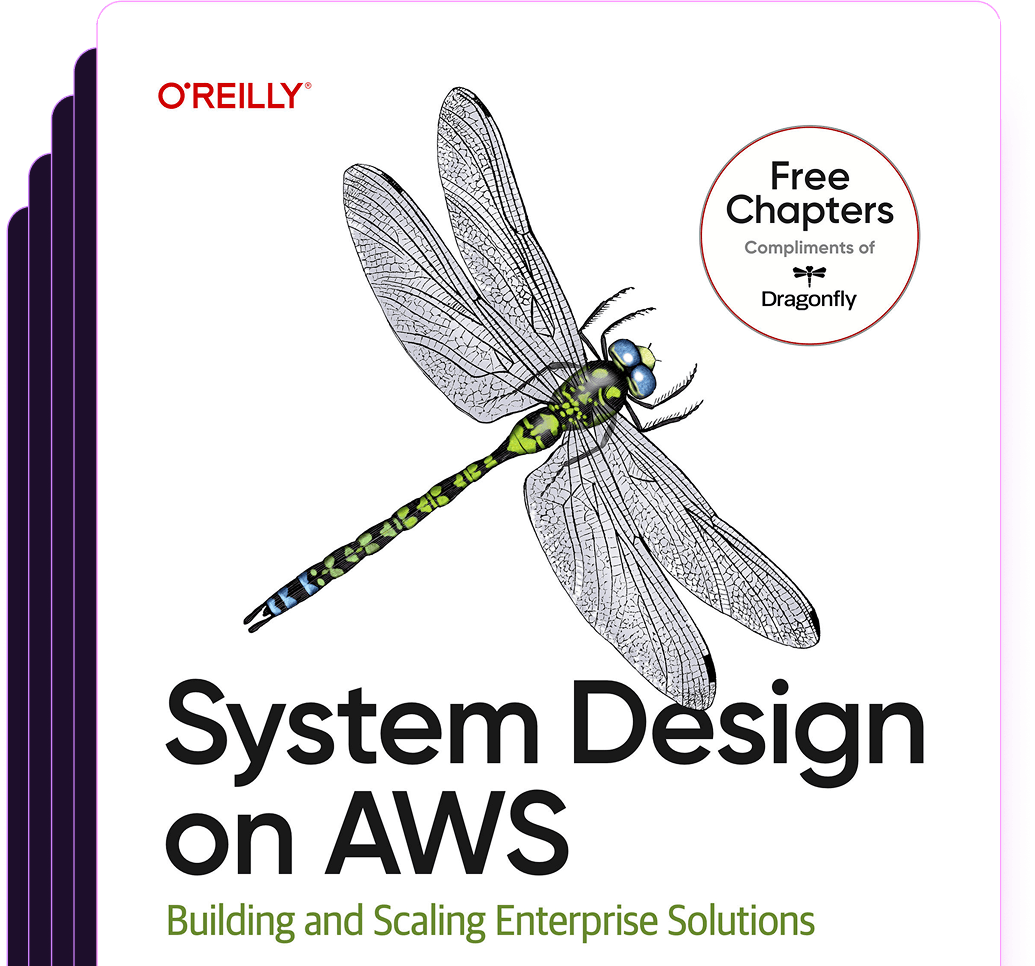
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost