Deleting a Redis Key After Time Using Golang (Detailed Guide w/ Code Examples)
Use Case(s)
In an application, you may want to store temporary data like session information, cached data, or real-time metrics in Redis. To avoid the data staying in Redis indefinitely, we can set a time-to-live (TTL) for these keys which automatically removes them after a certain period.
Code Examples
- Setting a TTL while creating a key: Here we'll create a key with a value and set the TTL during creation. CODE_BLOCK_PLACEHOLDER_0
In this example, 10*time.Second
sets the TTL for 'key' to 10 seconds.
- Setting a TTL for an existing key: We can also set a TTL for an already existing key. CODE_BLOCK_PLACEHOLDER_1
In this example, Expire
method is used to set the TTL for 'key' to 10 seconds.
Best Practices
Remember to handle errors properly in your production code. Also, be careful with the TTL values; setting them too short might cause data to disappear before it's used, setting them too long can consume more memory than necessary.
Common Mistakes
Setting data without a proper TTL where it's required can cause the Redis instance to run out of memory. Misunderstanding the TTL as milliseconds instead of seconds is also a common mistake.
FAQs
Q: Can I view the remaining TTL of a key? A: Yes. You can use the TTL
function: timeLeft, err := client.TTL("key").Result()
to get the remaining time-to-live (in seconds) for the specified key.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
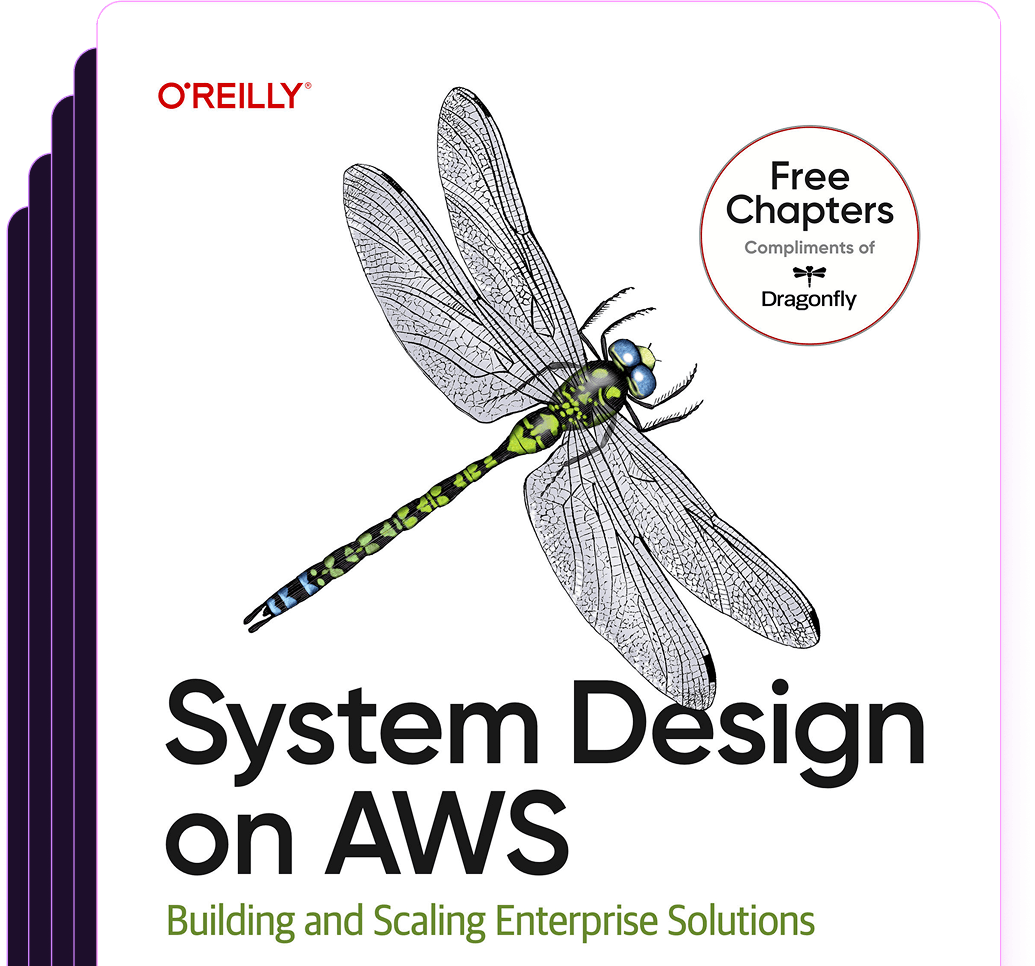
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost