Deleting Redis Cache in Golang (Detailed Guide w/ Code Examples)
Use Case(s)
For web applications, cached data can become outdated or irrelevant over time. In these cases, it is necessary to delete specific keys from the Redis cache. This operation is common during updates to database records that are also stored in cache, or when a session expires and related user data needs to be purged from cache.
Code Examples
To handle such scenarios in Go, we typically use the go-redis
library:
Example 1: Deleting a single key
package main
import (
"github.com/go-redis/redis/v8"
"context"
)
func main() {
ctx := context.Background()
rdb := redis.NewClient(&redis.Options{
Addr: "localhost:6379",
})
err := rdb.Del(ctx, "key1").Err()
if err != nil {
panic(err)
}
println("Deleted key1")
}
In this code piece, we create a connection to a local Redis instance, then delete the key 'key1' from cache.
Example 2: Deleting multiple keys
package main
import (
"github.com/go-redis/redis/v8"
"context"
)
func main() {
ctx := context.Background()
rdb := redis.NewClient(&redis.Options{
Addr: "localhost:6379",
})
err := rdb.Del(ctx, "key1", "key2", "key3").Err()
if err != nil {
panic(err)
}
println("Deleted keys")
}
In this example, we delete multiple keys ('key1', 'key2', 'key3') at once.
Best Practices
Always handle the error returned by Del()
to make sure the operation was successful. If the key doesn't exist, Redis will return 0, not an error, so don't rely solely on error handling to check the existence of a key.
Common Mistakes
Not checking if a key exists before trying to delete it can lead to confusion: Redis won't throw an error if you try to delete a non-existent key, it just returns 0. Thus, if you want to confirm whether a deletion was successful or if a key was already missing, you need to manually check the key's existence beforehand.
FAQs
Q: What happens if I try to delete a key that does not exist? A: Redis won't return an error, but rather a zero, indicating no keys were deleted.
Q: Can I delete multiple keys at once? A: Yes, the Del()
function accepts multiple keys as arguments.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Deleting a Redis Key with GoLang
- Deleting All Keys in Redis Using Golang
- Deleting a Redis Hash in Golang
- Deleting a Sorted Set in Redis Using Golang
- Deleting a Set in Redis using Golang
- Deleting Redis Keys by Pattern in Golang
- Golang: Deleting Redis Keys by Prefix
- Deleting a Redis Key After Time Using Golang
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
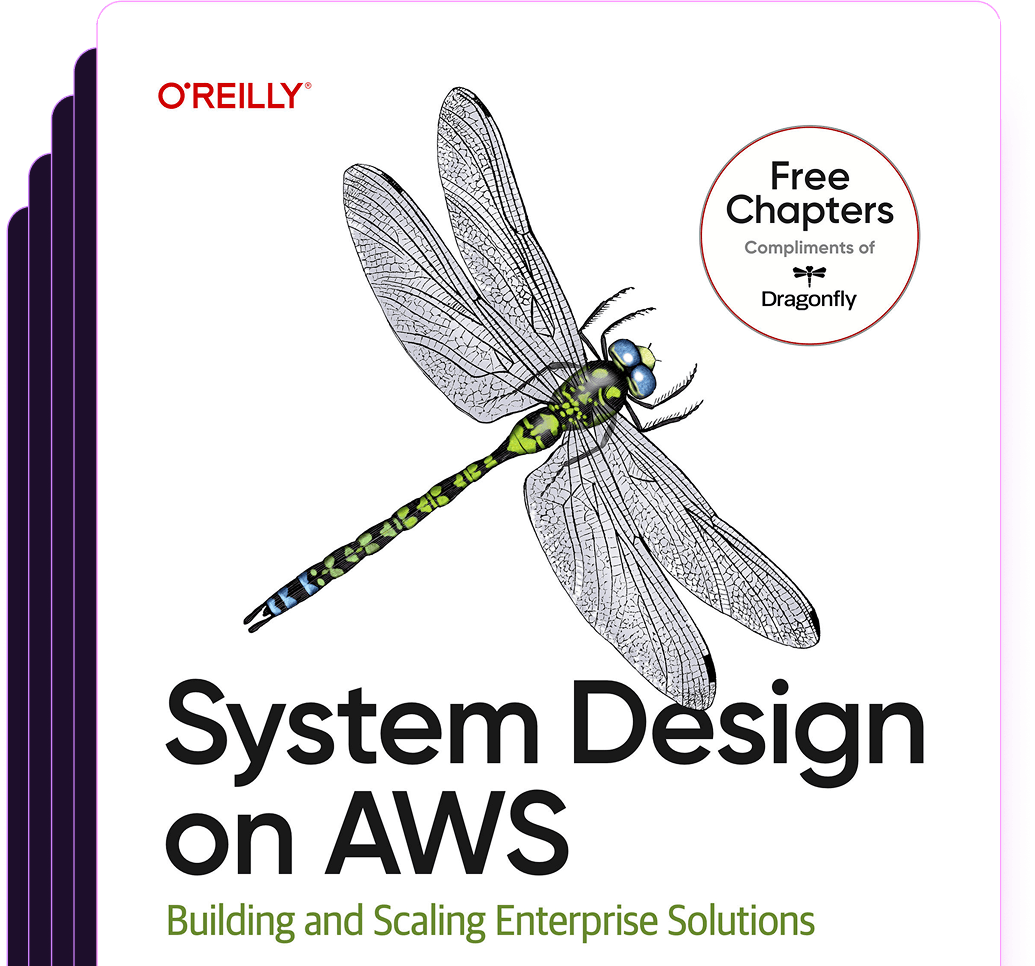
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost