Deleting Redis Keys by Pattern in Golang (Detailed Guide w/ Code Examples)
Use Case(s)
Deleting keys by a pattern is commonly used when you need to remove all keys that match a certain pattern. This can be useful for instance when you want to delete session data related to a particular user, or clear entries of a specific type.
Code Examples
To use Golang with Redis, we commonly use the go-redis
library. Below are examples showing how to delete keys by pattern.
Example 1: Deleting keys using a single pattern CODE_BLOCK_PLACEHOLDER_0
Best Practices
When deleting keys by pattern, ensure to limit the usage in production environments because the KEYS
command can be resource-intensive and might affect the performance of the Redis server if the key-space is large. Always test this operation in a controlled setting.
Common Mistakes
One common mistake is not handling errors correctly when getting keys or deleting them. It's important to always check for errors so that you can handle them appropriately and prevent your application from crashing unexpectedly.
FAQs
Q: What if I want to delete keys across all databases? A: In Redis, the KEYS
command only operates on the currently selected database. You would need to run the deletion script individually for each database.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
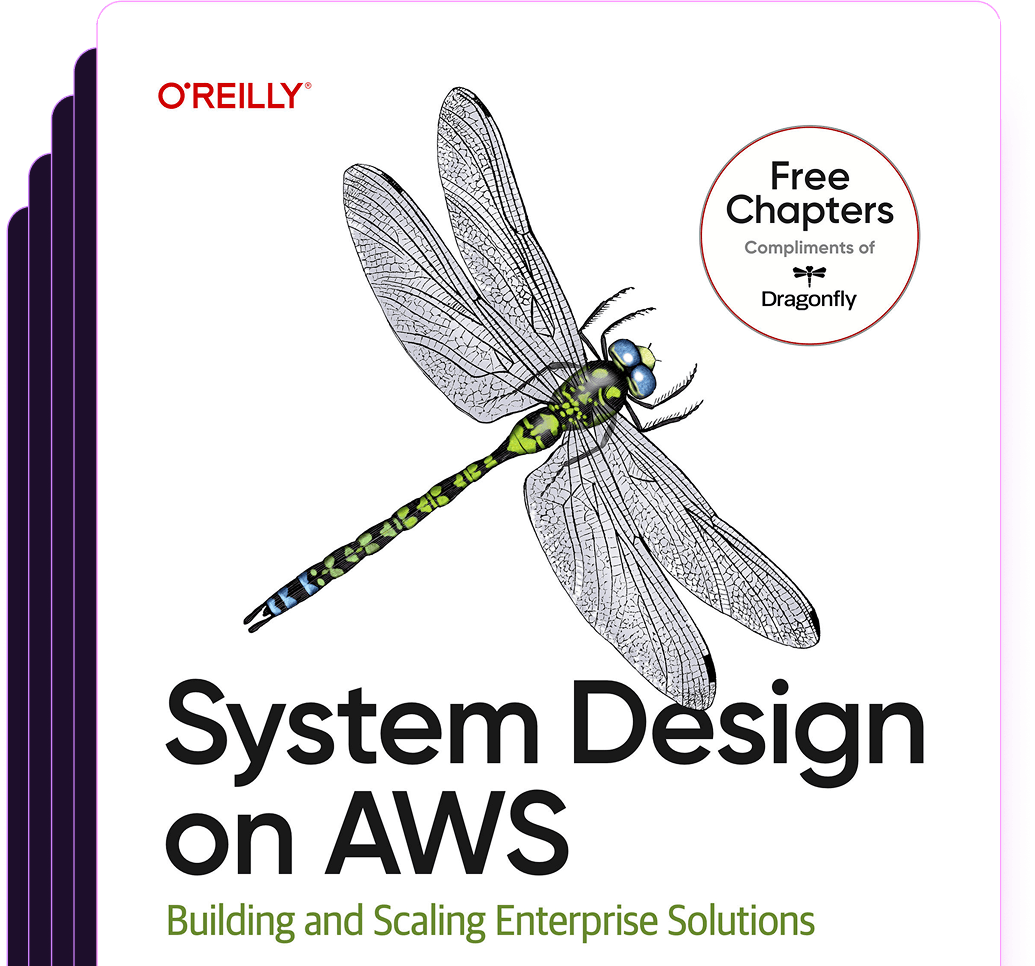
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost