Deleting a Set in Redis using Node.js (Detailed Guide w/ Code Examples)
Use Case(s)
The primary use case for deleting sets in Redis using Node.js is when you want to remove unwanted data from your Redis database. This can help free up memory and keep your database organized.
Code Examples
Here's an example of how to delete a set in Redis using the del
command in Node.js:
const redis = require('redis');
// Create a client and connect to Redis
const client = redis.createClient({
host: 'localhost',
port: 6379,
});
client.on('connect', function() {
console.log('Connected to Redis...');
});
// Delete a set
client.del('mySet', function(err, response) {
if(err) throw err;
console.log(`Deleted ${response} set`);
});
In this example, we first create a Redis client then connect to the Redis server. We then use the del
command to delete a set named 'mySet'. The callback function logs the confirmation of deleted set on successful deletion.
Remember that the del
command can be used to delete not only sets but also keys, lists, hashes, etc.
Best Practices
- When deleting a set, ensure that the set is no longer being used by your application.
- Handle errors appropriately to prevent your application from crashing due to an unexpected error.
- Always disconnect the client when you're done with your operations.
Common Mistakes
- Forgetting to handle errors that may occur during deletion.
- Trying to delete a set that doesn't exist. The
del
command will simply return 0 in this case.
FAQs
Q: What does the del
command return?
A: The del
command returns the number of keys that were removed.
Q: Can I delete multiple sets at once?
A: Yes, you can delete multiple sets at once using the del
command. Simply pass multiple key names as arguments to the del
command.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
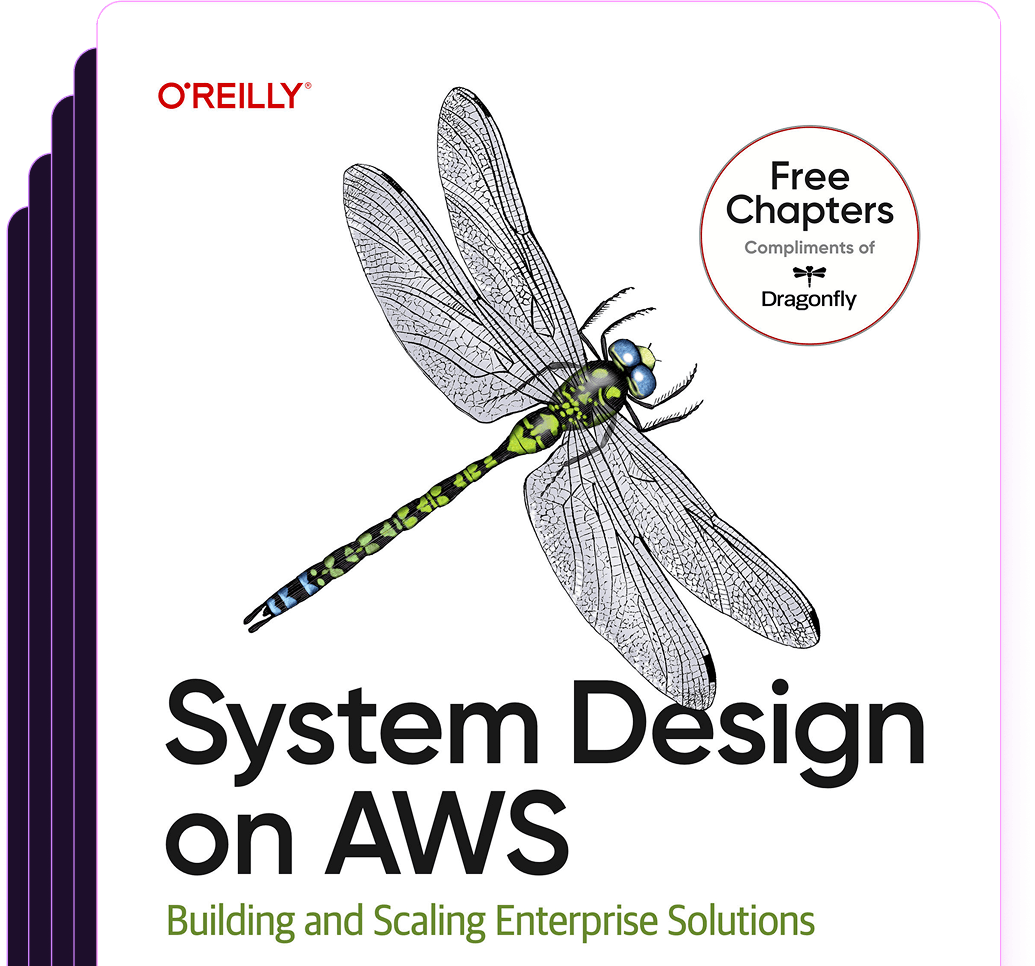
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost