Node Redis: Delete Hash (Detailed Guide w/ Code Examples)
Use Case(s)
In Node.js applications using Redis, one common use case of deleting a hash is when you want to remove an entire set of key-value pairs stored in a hash. This could be useful for cache invalidation, user session termination, or data migration.
Code Examples
To delete a hash in Redis using Node.js, you can use the del
command from the redis node package. Consider the following example:
const redis = require('redis');
let client = redis.createClient();
client.del('hashKey', function(err, response) {
if(err) {
console.log(err);
}
console.log(response);
});
This code will delete the hash with the key 'hashKey'. If successful, it will return 1, else it will return 0.
Best Practices
- Always handle potential errors in your callback function. Ignoring them can lead to unforeseen consequences.
- Ensure that the key you are trying to delete actually exists to avoid unnecessary operations.
Common Mistakes
- Trying to delete a key that does not exist or has already been deleted.
- Not handling errors in the callback function.
FAQs
Q: What happens if I try to delete a key that doesn't exist? A: The del
command will return 0 indicating that no key was deleted.
Q: Are there any performance considerations when deleting a hash? A: Deleting a large hash in a single operation can be costly in terms of performance. If possible, consider deleting large hashes incrementally to avoid blocking the Redis event loop for long periods of time.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
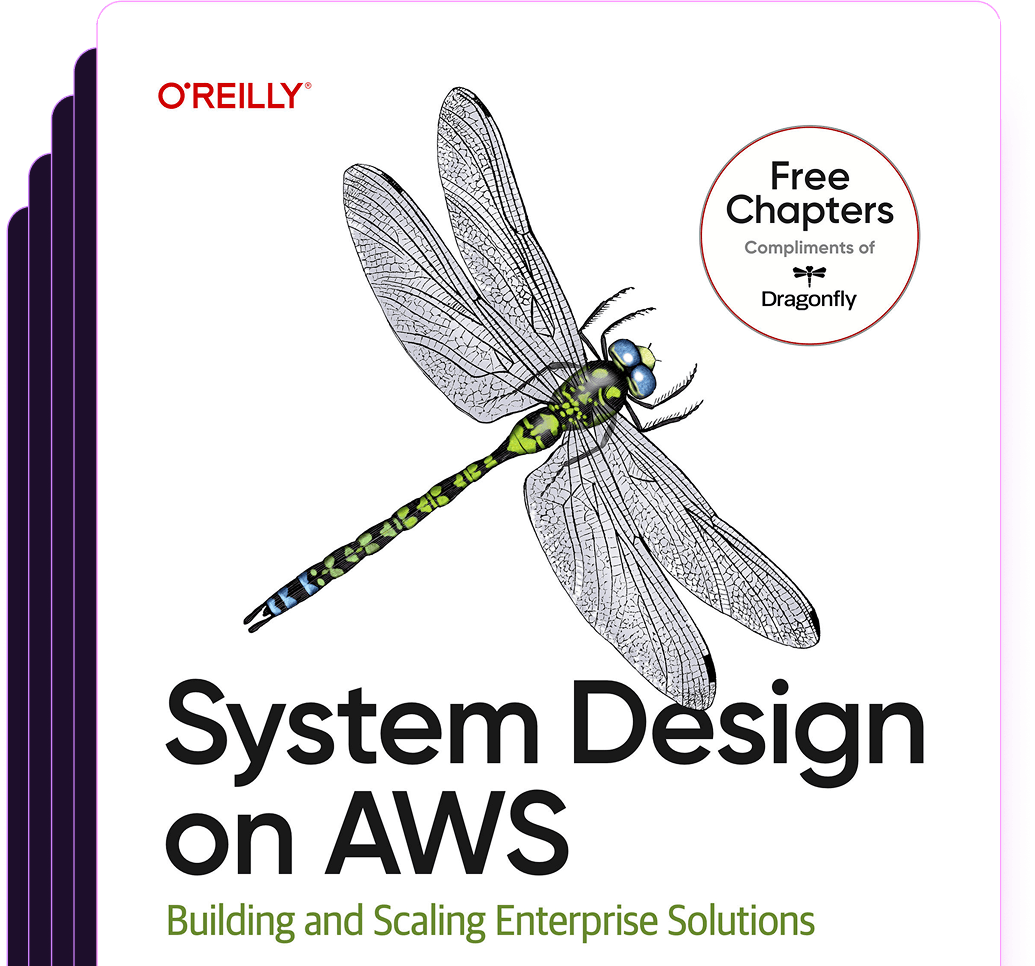
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost