Node.js Redis: Delete Key After Time (Detailed Guide w/ Code Examples)
Use Case(s)
The keyword 'node redis delete key after time' relates to a common use case where you want to automatically delete certain keys from your Redis database after a certain period of time. This can be useful when you're caching data that becomes obsolete after a specified duration.
Code Examples
Example 1: Using client.setex()
In Node.js, we can make use of the setex()
function from the redis
client to set a key to hold a string value and set a timeout on it in seconds.
var redis = require('redis');
var client = redis.createClient();
client.on('connect', function() {
console.log('connected');
});
// setting the key 'session' with expiry of 300 seconds
client.setex('session', 300, 'active');
In this example, we're setting the key 'session' with the value 'active', and it will be automatically deleted after 300 seconds.
Best Practices
- Make sure to handle any errors when connecting to the Redis client or while running commands.
- Use meaningful key names so you can easily identify what the key-value pairs are used for.
- Be mindful of the expiration times you set. Setting too short of an expiration time might result in the data being removed before it is utilized. On the other hand, too long expiration times may unnecessarily occupy memory.
Common Mistakes
One mistake is not considering the TTL (Time-To-Live) value when setting the keys. If keys are set without a TTL, they will persist in Redis until explicitly deleted, which may not be the desired behavior when working with temporary data.
FAQs
Q: What happens if I try to access a key after it has expired? A: When you try to access a key that has expired, Redis will act as if the key does not exist and return a nil value.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
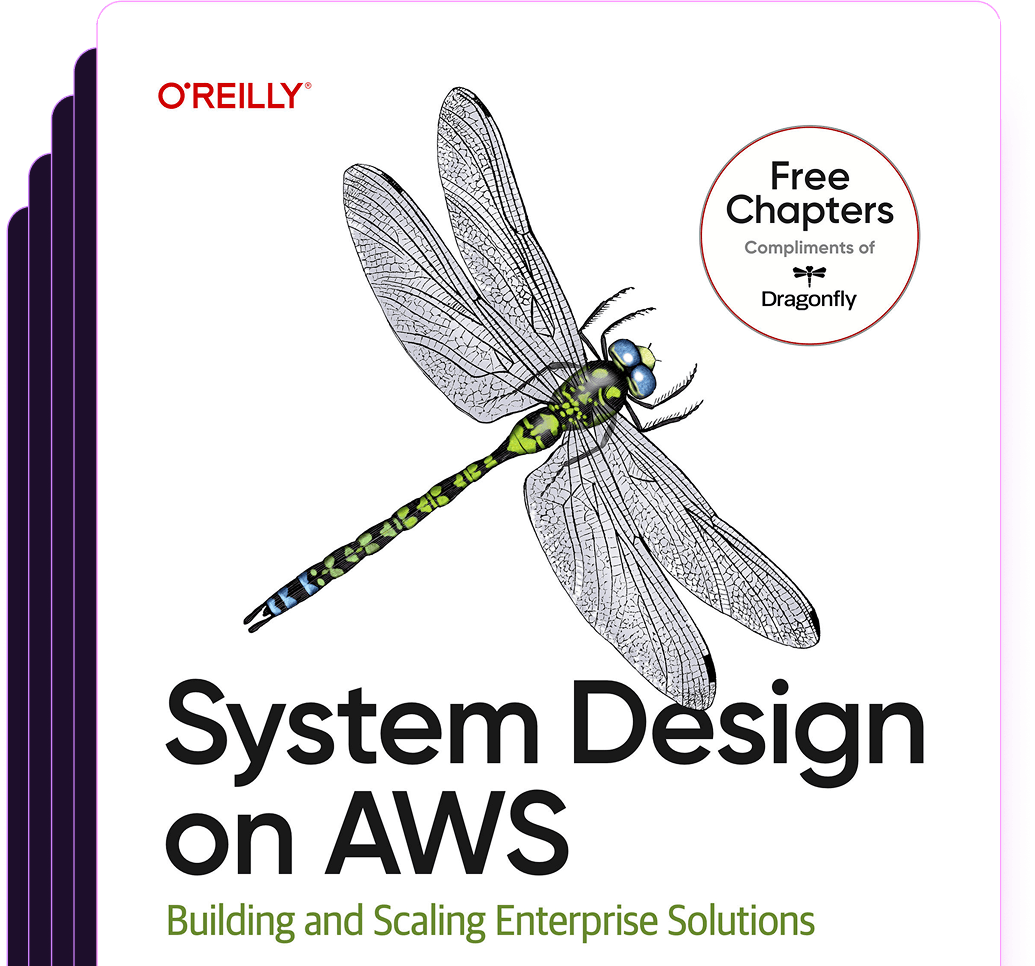
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost