Node Redis: Delete Keys by Pattern (Detailed Guide w/ Code Examples)
Use Case(s)
In a Node.js application backed by Redis, there may be times when you need to delete keys based on a specific pattern. For example, in a caching scenario, you might use key patterns to represent different versions of the same data, and you need to clear out old versions.
Code Examples
To accomplish this, you'll need to use a combination of Redis SCAN
command along with the DEL
command. Here's how it looks:
const redis = require('redis');
const client = redis.createClient();
let cursor = '0';
do {
client.scan(cursor, 'MATCH', 'yourpattern*', 'COUNT', '100', function(err, reply) {
if (err) throw err;
cursor = reply[0];
reply[1].forEach(function(key) {
client.del(key, function(err, reply) {
console.log('Key deleted:', key);
});
});
});
} while (cursor !== '0');
This script will scan your Redis database for keys that match your pattern (yourpattern*
) and delete them. It does it in a loop until it has scanned all keys.
Best Practices
- Avoid using this method during peak load times as it could slow down your application.
- Always test your pattern thoroughly to ensure you're not deleting unintended keys.
Common Mistakes
- Not considering the impact of key deletion on live systems. Be very careful when deleting keys in a production environment.
FAQs
Q: Can I use this method to delete keys across multiple databases in Redis? A: No, the SCAN
command only works within the currently selected database.
Q: What if my pattern matches more keys than the count I specified? A: The script will handle this by continuously looping until all matching keys are deleted.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
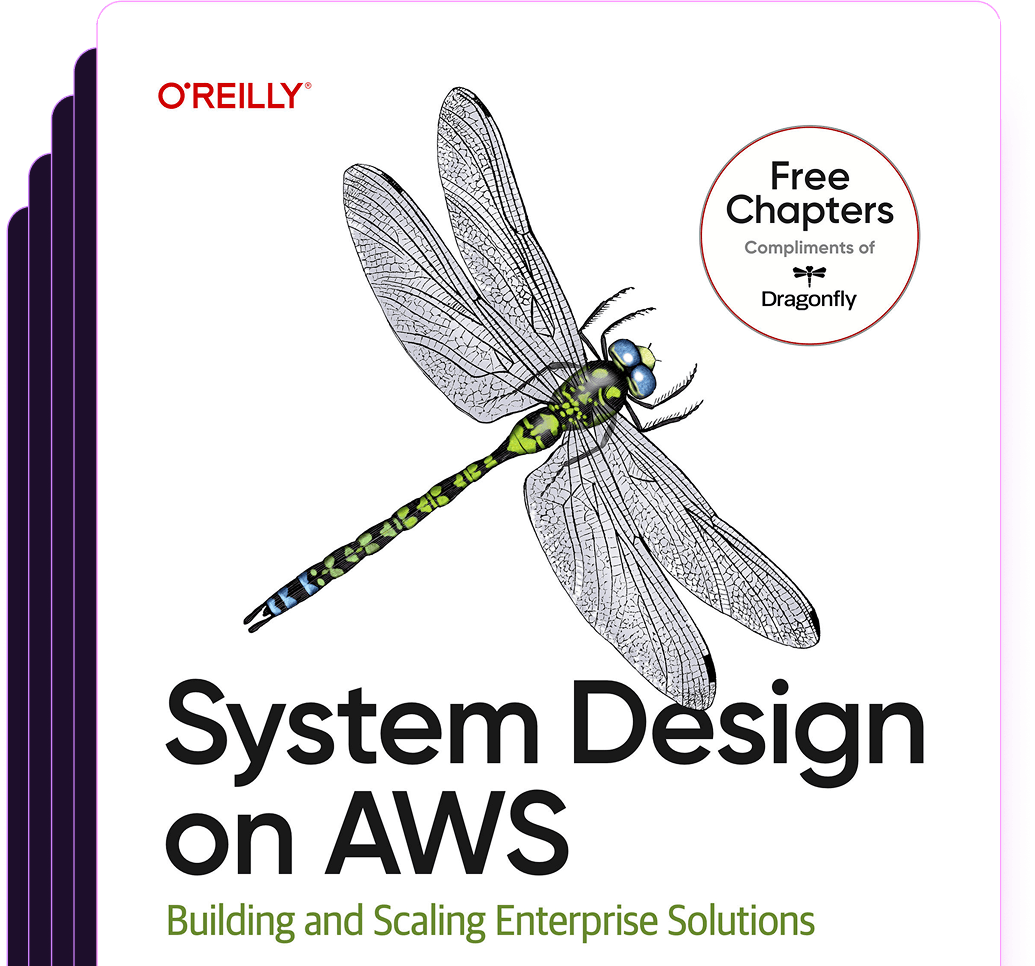
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost